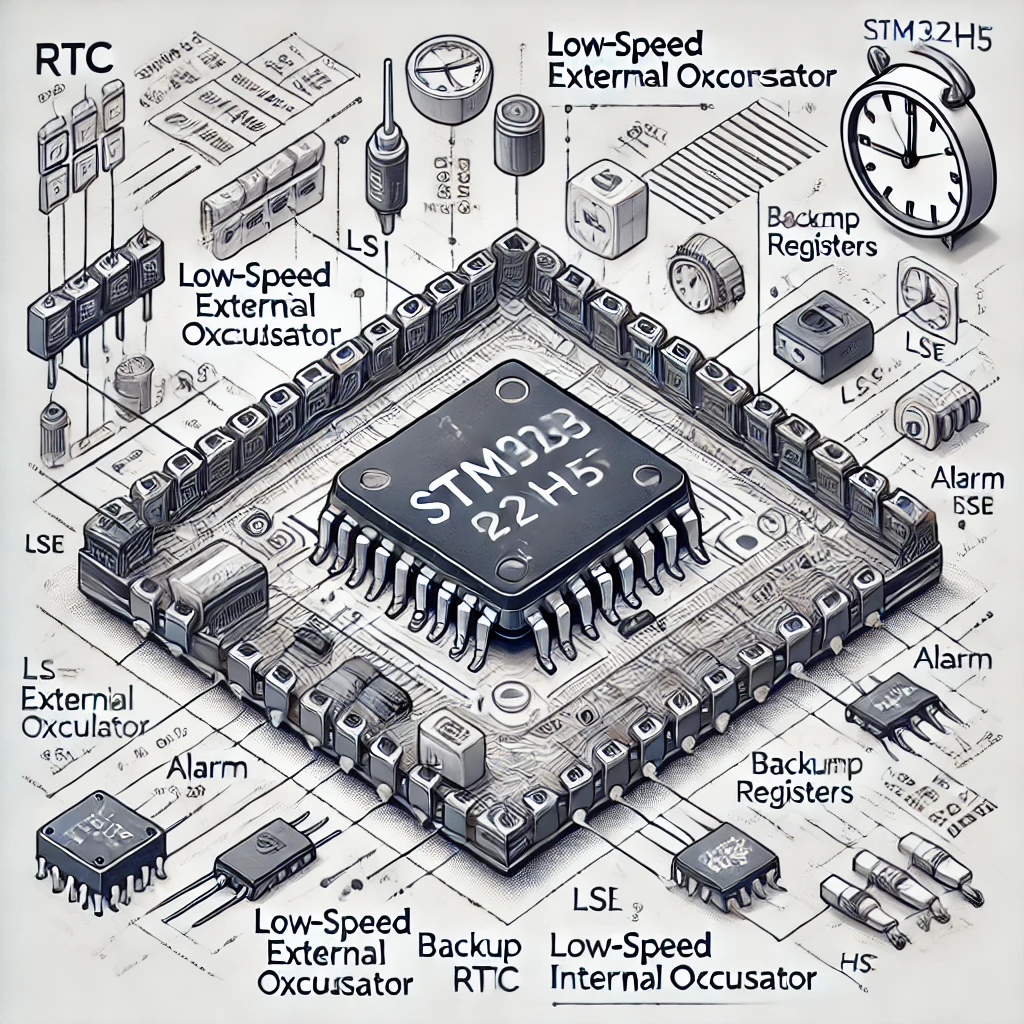
In this guide on RTC (Real-Time Clock), we shall explore the features of the RTC on STM32H5, its functional block diagram, and how to develop a driver using STM32CubeMX.
In this guide, we shall cover the following:
• What is RTC.
• STM32CubeIDE Configuration.
• Firmware development.
• Results.
1. What is RTC:
Introduction to the STM32H5 Internal RTC
The STM32H5 microcontroller features a highly versatile Real-Time Clock (RTC) designed for applications that require precise timekeeping and date tracking. The internal RTC operates independently of the main system clock, ensuring accurate functionality even during low-power modes. This makes it ideal for devices such as smart meters, data loggers, wearable electronics, and other time-sensitive embedded systems.
Key Features of the Internal RTC:
1. Low-Power Operation:
• The RTC can remain operational in Standby or Stop modes, consuming minimal power.
2. Flexible Clock Source:
• Supports multiple clock sources, including the Low-Speed External oscillator (LSE), Low-Speed Internal oscillator (LSI), and High-Speed External oscillator (HSE).
3. Calendar Functionality:
• Tracks year, month, day, hours, minutes, and seconds with leap year compensation.
4. Programmable Alarms and Wakeup Timer:
• Features two alarms and a wakeup timer for precise timing events and system wake-ups.
5. Backup Registers:
• Includes 20 backup registers to retain user data during power loss or system resets.
6. Tamper Detection:
• Monitors tamper events and secures critical data.
Block Diagram Overview
The block diagram of the RTC provides a clear representation of its components and connections. It includes the following elements:
• Clock Sources:
• LSE, LSI, and HSE provide the flexibility to select the most appropriate clock source for the application.
• Prescaler and Divider:
• Adjusts the input clock to generate the 1 Hz signal required for the RTC core.
• Calendar Unit:
• Maintains accurate time and date with leap year adjustments.
• Alarm and Wakeup Unit:
• Enables system alerts and wakeup events.
• Backup Registers:
• Stores user data securely during power cycles.
• Tamper Detection:
• Protects sensitive information by detecting external tamper events.
The internal RTC of the STM32H5 is a reliable and flexible solution for managing time-critical operations in embedded systems. The following block diagram illustrates its architecture and connections:
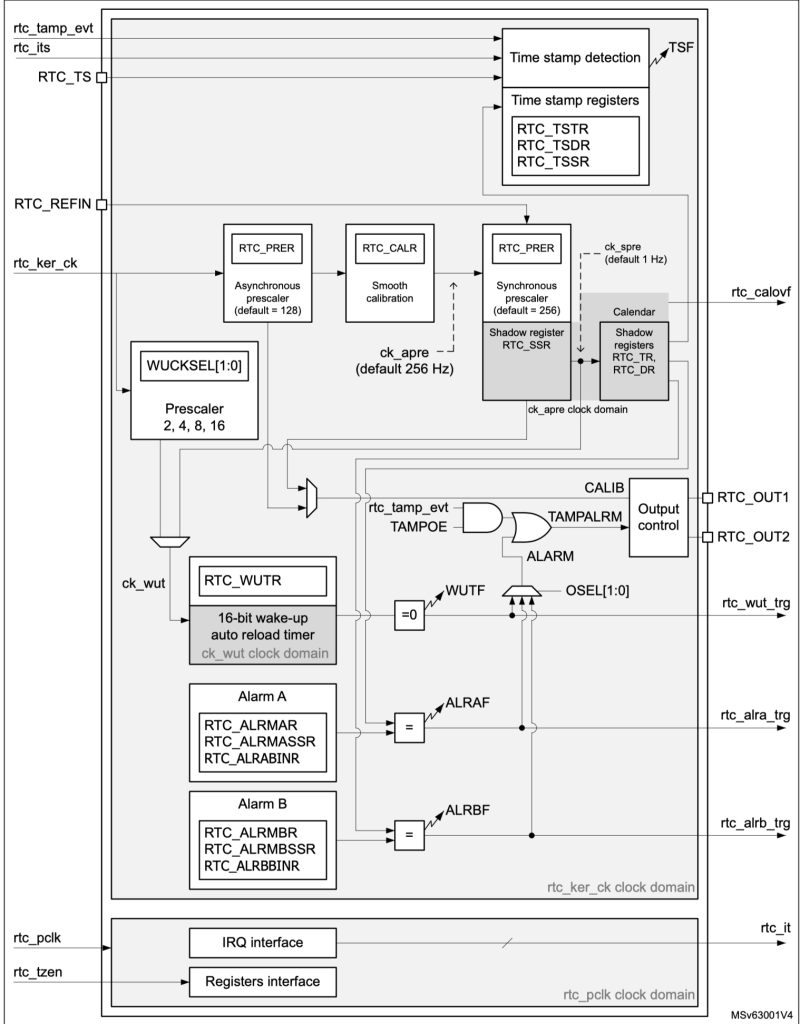
2. STM32CubeIDE Configuration:
We start off by creating new project with name of Internal RTC. For how to create project using STM32CubeIDE, please refer to this guide here.
After creating the project, the STM32CubeMX configuration window will appear.
From System Core from left, select RCC then enable external low speed oscillator as following:
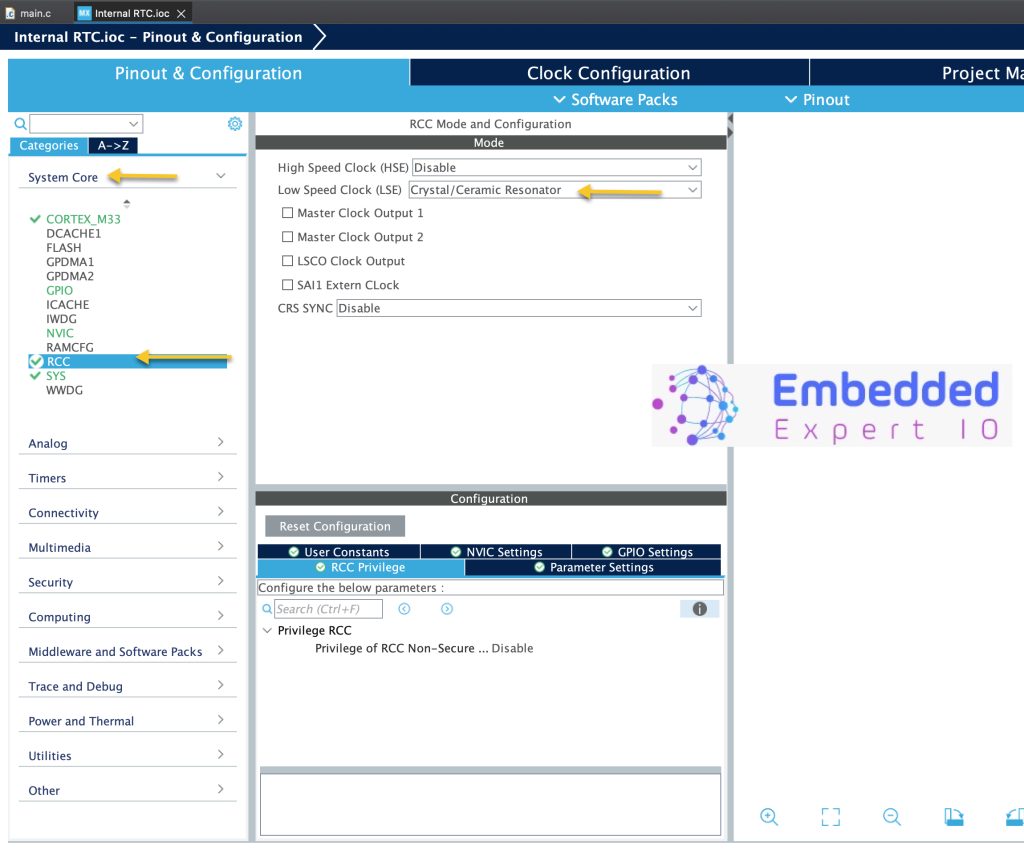
Next, from timers section, select RTC then activate Clock Sources and Calendar as following:
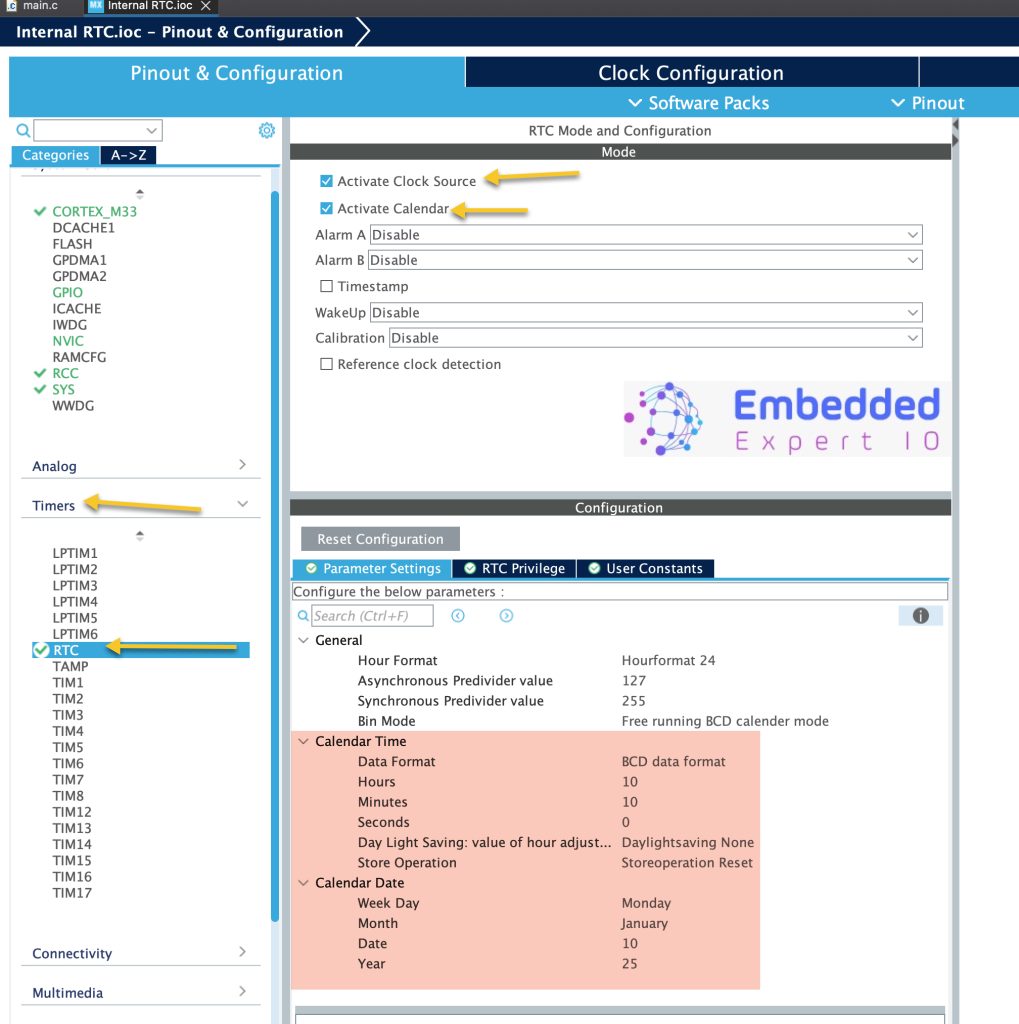
Also, set the time and date as you wish. Here, the values are random.
Next, go to clock configuration, set the RTC clock source to be LSE (Low Speed External) as following:
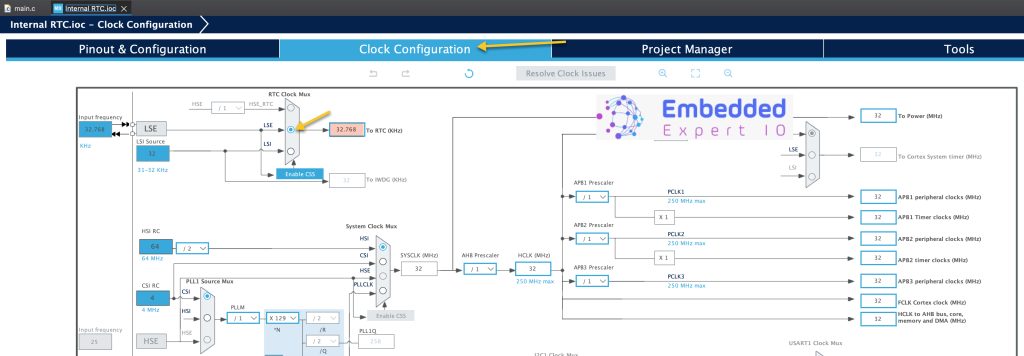
Also, enable the UART to print the RTC value. For how to enable the uart, please refer to this guide here.
Thats all for the configuration.
Save the project and this will generate the project.
3. Firmware Development:
In main.c file.
In user begin includes, include the stdio header file as following:
/* USER CODE BEGIN Includes */ #include "stdio.h" /* USER CODE END Includes */
Next, in user code begin PV, declare the following two data structure for the RTC operation:
/* USER CODE BEGIN PV */ RTC_TimeTypeDef gTime = {0}; RTC_DateTypeDef gDate = {0}; /* USER CODE END PV */
First data structure is to store the time while the second one is to store the date.
In user code begin 0, we shall retarget the printf to user UART as following:
int __io_putchar(int ch) { HAL_UART_Transmit(&huart2, &ch, 1, 5); return ch; }
This will allow us to use terminal to display the results.
In RTC_MX_Init function, at user code begin check_RTC_BKUP:
/* USER CODE BEGIN Check_RTC_BKUP */ if (HAL_RTCEx_BKUPRead(&hrtc, RTC_BKP_DR1)== 0x4567) { return ; } /* USER CODE END Check_RTC_BKUP */
This line, will prevent entering new date and time once the MCU is reseted.
At user code begin RTC_Init_2, add this line:
/* USER CODE BEGIN RTC_Init 2 */ HAL_RTCEx_BKUPWrite(&hrtc, RTC_BKP_DR1, 0x4567); /* USER CODE END RTC_Init 2 */
By writing any value to the RTC backup register, this will lock the RTC from configuration. During the boot, we shall check this register if it has the same value, then no need to reinitialize the RTC data.
In while 1 loop, user code begin 3:
if (HAL_RTC_GetTime(&hrtc, &gTime, RTC_FORMAT_BIN) != HAL_OK) { Error_Handler(); } if(HAL_RTC_GetDate(&hrtc, &gDate, RTC_FORMAT_BIN)!=HAL_OK) { Error_Handler(); } printf("Time is %d:%d:%d\r\n",gTime.Hours,gTime.Minutes,gTime.Seconds); printf("Date is %d/%d/%d\r\n",gDate.Date,gDate.Month,gDate.Year); HAL_Delay(500);
Thats all for the firmware development.
Save the project, build it and run it on your STM32H563Zi board.

4. Results:
After the running the project, open your favourite serial terminal and set the baud rate to 115200 and you should get the RTC values as following:
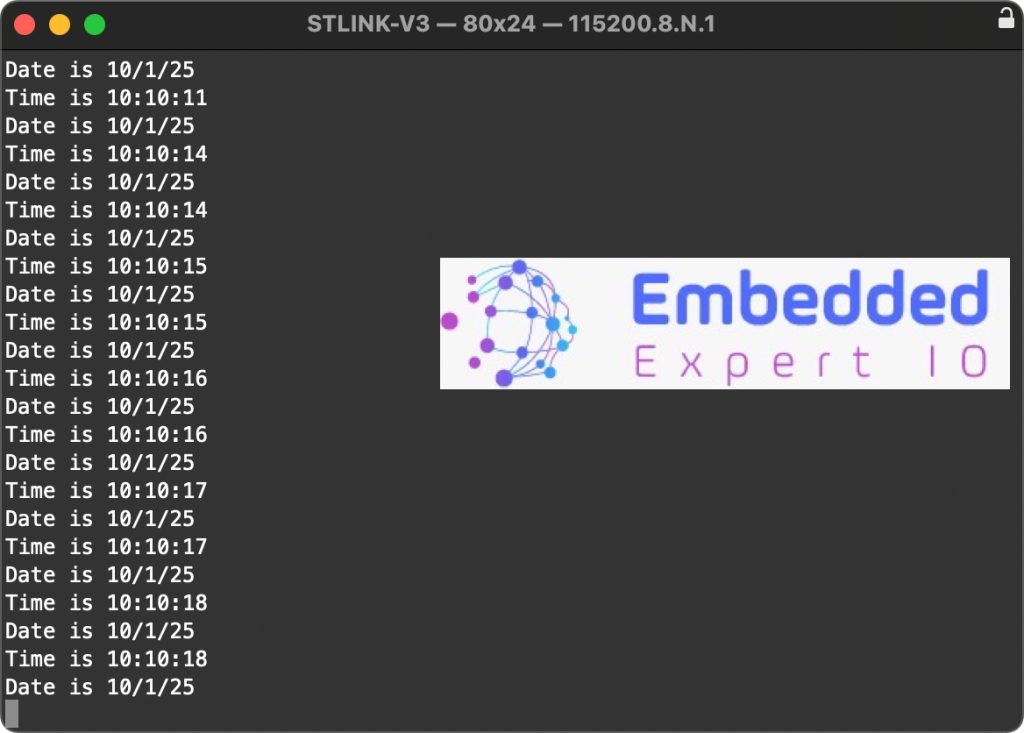
Happy coding 😉
Add Comment