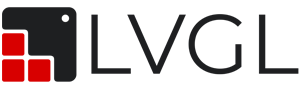
In this guide, we shall take a look at what is LvGL and setup the environment to run LvGL on STM32F429-disco.
In this guide, we shall cover the following:
- What is LvGL.
- Environment Setup.
1. What is LvGL:
LVGL (Light and Versatile Graphics Library) is an open-source graphics library designed for creating embedded graphical user interfaces (GUIs). It provides a comprehensive set of tools and features to build beautiful UIs with ease. Here are some key points about LVGL:
- Versatility: LVGL is suitable for a wide range of devices, including microcontrollers (MCUs), microprocessors (MPUs), and various display types. Whether you’re working on a smartwatch, smartphone-like interface, or any other embedded system, LVGL can be a great choice.
- Widgets and Layouts: LVGL offers 30+ built-in widgets, which are pre-designed UI elements like buttons, labels, sliders, and more. Additionally, it provides web-inspired layout managers to arrange these widgets efficiently.
- Typography and Fonts: LVGL supports a typography system that can handle many languages. You can easily render text with different fonts and styles.
- Low Memory Footprint: Despite its rich features, LVGL maintains a small memory footprint. For example, the LVGL library itself requires only around 50KB of RAM and 100KB of flash memory.
- No External Dependencies: LVGL is fully open-source and doesn’t rely on any external libraries. This makes it easy to port to different platforms and integrate with various operating systems (OS) or bare-metal setups.
- Commercial Use: LVGL is free even for commercial projects, making it an attractive choice for both hobbyists and professional developers.
Collaborations and Support
Several companies and communities collaborate with LVGL:
- NXP: NXP tightly integrates LVGL into their ecosystem, allowing easy integration into MCUXpresso projects. They are also working on first-class support for VG-Lite and PXP accelerators on i.MX RT devices.
- Espressif: LVGL seamlessly integrates with Espressif’s package manager, making it straightforward to get started. Espressif’s display drivers work well with LVGL for IoT development.
- Xiaomi: Xiaomi has used LVGL in numerous devices and appreciates its performance and lightweight nature. They actively collaborate with LVGL’s open-source community.
- Nuvoton: Nuvoton’s customers use LVGL for developing smart devices related to the Industrial Internet of Things (IIoT).
- Renesas: Renesas pairs its advanced microcontrollers with LVGL, providing ready-to-use projects for their boards.
2. Environment Setup:
Before we start, we need to setup STM32CubeIDE to work in register level programming, please following this guide to set STM32CubeIDE.
Just change STM32F411xE to the following:
STM32F429xx
This will tell the compiler to use STM32F429 as main header file
We start off by creating new source file with name of sys_init.c. This source file shall contain the core setup to run STM32F429 at maximum speed of 180MHz.
Within the source file:
#include "stm32f4xx.h" void SystemInit (void) { #define PLL_M 4 #define PLL_N 180 #define PLL_P 2 #define PLL_Q 9 __IO uint32_t StartUpCounter = 0, HSEStatus = 0; RCC->CR |= ((uint32_t)RCC_CR_HSEON); do { HSEStatus = RCC->CR & RCC_CR_HSERDY; StartUpCounter++; } while((HSEStatus == 0) && (StartUpCounter != 3000)); if ((RCC->CR & RCC_CR_HSERDY) != RESET) { HSEStatus = (uint32_t)0x01; } else { HSEStatus = (uint32_t)0x00; } if (HSEStatus == (uint32_t)0x01) { RCC->APB1ENR |= RCC_APB1ENR_PWREN; PWR->CR &= (uint32_t)~(PWR_CR_VOS); RCC->CFGR |= RCC_CFGR_HPRE_DIV1; RCC->CFGR |= RCC_CFGR_PPRE2_DIV1; RCC->CFGR |= RCC_CFGR_PPRE1_DIV2; RCC->PLLCFGR = PLL_M | (PLL_N << 6) | (((PLL_P >> 1) -1) << 16) | (RCC_PLLCFGR_PLLSRC_HSE) | (PLL_Q << 24); RCC->CR |= RCC_CR_PLLON; while((RCC->CR & RCC_CR_PLLRDY) == 0) { } /* Configure Flash prefetch, Instruction cache, Data cache and wait state */ FLASH->ACR = FLASH_ACR_ICEN |FLASH_ACR_DCEN |FLASH_ACR_LATENCY_5WS; /* Select the main PLL as system clock source */ RCC->CFGR &= (uint32_t)((uint32_t)~(RCC_CFGR_SW)); RCC->CFGR |= RCC_CFGR_SW_PLL; /* Wait till the main PLL is used as system clock source */ while ((RCC->CFGR & (uint32_t)RCC_CFGR_SWS ) != RCC_CFGR_SWS_PLL) {;} } else { /* If HSE fails to start-up, the application will have wrong clock configuration. User can add here some code to deal with this error */ } /*Enable FPU*/ SCB->CPACR |= ((3UL << 10*2)|(3UL << 11*2)); /*Enable cell compensation*/ RCC->APB2ENR|=RCC_APB2ENR_SYSCFGEN ; SYSCFG->CMPCR|=(1<<0); while(!((SYSCFG->CMPCR)&(1<<8))){;} }
For more information about how to configure the core, please refer to this guide.
Now, create new source and header file with name of delay.c and delay.h respectively.
Within delay.h header file:
#ifndef DELAY_H_ #define DELAY_H_ #include "stdint.h" void delay_init(uint32_t freq); uint64_t millis(); void delay(uint32_t time); #endif /* DELAY_H_ */
Within delay.c source file:
#include "delay.h" #include "stm32f4xx.h" #define CTRL_ENABLE (1U<<0) #define CTRL_CLKSRC (1U<<2) #define CTRL_COUNTFLAG (1U<<16) #define CTRL_TICKINT (1U<<1) volatile uint64_t mil; void delay_init(uint32_t freq) { SysTick->LOAD = (freq/1000) - 1; /*Clear systick current value register */ SysTick->VAL = 0; /*Enable systick and select internal clk src*/ SysTick->CTRL = CTRL_ENABLE | CTRL_CLKSRC ; /*Enable systick interrupt*/ SysTick->CTRL |= CTRL_TICKINT; } uint64_t millis() { __disable_irq(); uint64_t ml=mil; __enable_irq(); return ml; } void delay(uint32_t time) { uint64_t start=millis(); while((millis() - start) < (time+1)); } void SysTick_Handler(void) { mil++; }
In part 2, we shall cover the initialization of LCD and touch driver.
Stay tuned.
Happy coding 😉
Add Comment