In the pervious guide, we took a look at how to send data over SPI (from here). In this guide, we shall use SPI to drive a lcd and display some variables on it.
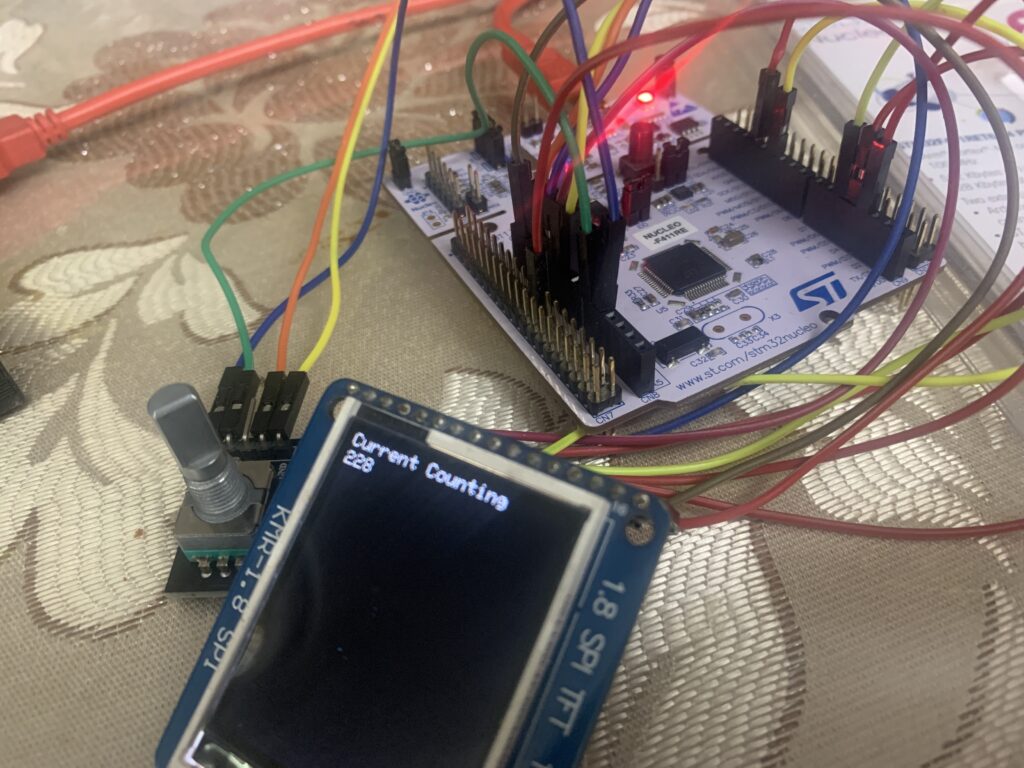
In this guide, we will need the following topics:
In this guide, we shall cover the following:
- Connection
- ST7735 initialization
- Code
- Demo
1. Connection:
My ST7735 based TFT has the following pinout
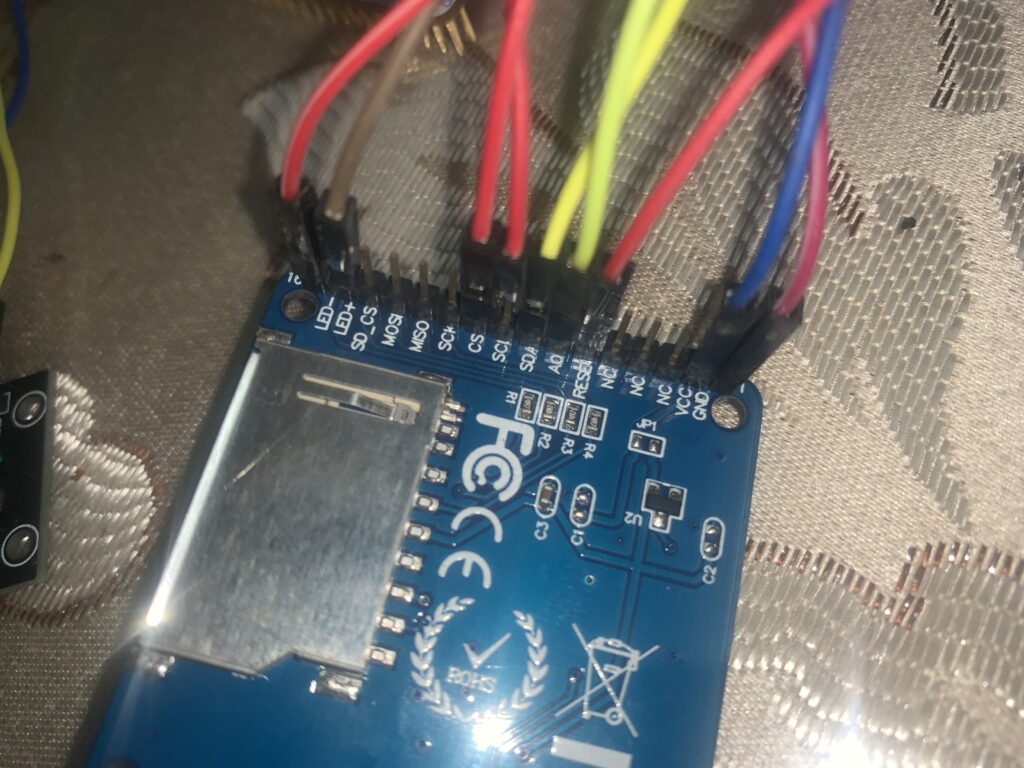
The pins as following:
Pin | discerption |
GND | Ground |
Vcc | 5V input |
RESET | Reset pin |
A0 | Data/Command |
SDA | MOSI |
SCL | SCK |
CS | slave select |
LED+ | 3v3 for LED |
LED- | Ground of LED |
Since we will use STM32F411RE Nucelo-64, the connection shall be as following:
LCD pin | Nucleo Pin |
GND | GND |
Vcc | 5V |
RESET | PB3 |
A0 | PB4 |
SDA | PA7 |
SCL | PA5 |
CS | PB5 |
LED+ | 3v3 |
LED- | GND |
2. ST7735 initializing:
We start off by defining some macros for reset, slave select and data/command
#define LCD_RST1 GPIOB->BSRR|=GPIO_BSRR_BS3 #define LCD_RST0 GPIOB->BSRR|=GPIO_BSRR_BR3 #define LCD_DC1 GPIOB->BSRR|=GPIO_BSRR_BS4 #define LCD_DC0 GPIOB->BSRR|=GPIO_BSRR_BR4 #define LCD_CS1 GPIOB->BSRR|=GPIO_BSRR_BS5 #define LCD_CS0 GPIOB->BSRR|=GPIO_BSRR_BR5
In SPI, to enable the slave, we need to set CS pin to low
To reset the LCD, we need to set the reset pin to low and set it back to high to release from reset
For Data/Command: When the pin is high, the LCD is in data mode (set pixel color for example). When pin is low, the lcd in command mode (set pixel position for example).
hence, the write data command can be like this:
void static writecommand(uint8_t c) { LCD_CS0; LCD_DC0; //Set DC low lcd7735_senddata(c); delayuS(2); LCD_CS1; } void static writedata(uint8_t d) { LCD_CS0; LCD_DC1;//Set DC HIGH lcd7735_senddata(d); delayuS(2); LCD_CS1; }
The intializing sequence is as following:
LCD_RST0; // RST=0 delay(10); //delay for 10 millisecond LCD_RST1; // RST=1 delay(10); //delay for 10 millisecond LCD_CS0; //enable LCD lcd7735_sendCmd(0x11); delay(10); lcd7735_sendCmd (0x3A); //Set Color mode lcd7735_sendData(0x05); //16 bits lcd7735_sendCmd (0x36); lcd7735_sendData(0x14); lcd7735_sendCmd (0x29);//Display on
Regarding drawing some shapes etc can be found in the header file of ST7735.h
3. Code:
you can download the entire code from here
link : Enocder-Interface-with-stm32
2 Comments
…you write “We start off by defining some micros for reset, slave select and data/command” …it is MACROS not MICROS
Hi,
thank you and I fixed it 🙂
Add Comment