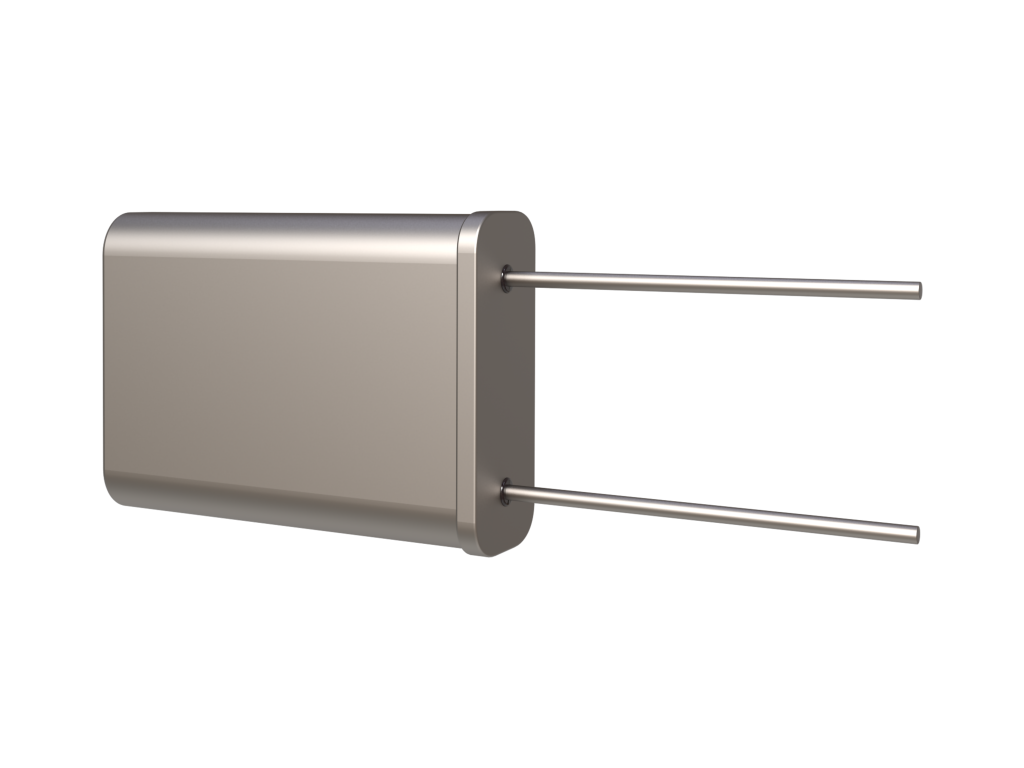
In this guide, we shall use the Master Clock Output (MCO) feature of STM32 to check the external crystal connected to STM32.
In this guide, we shall cover the following:
- What is MCO.
- Configuration.
- Results.
1. What is MCO:
MCO (Microcontroller Clock Output) is a feature available in many STM32 microcontrollers that allows the clock signal from an internal or external clock source to be output on a specific pin. This feature can be useful for various purposes, such as providing a clock signal to other devices in your system or monitoring the clock frequency for debugging.
Key Points About MCO:
- Clock Sources:
• The MCO pin can output different clock signals, including:
• HSI (High-Speed Internal) oscillator: Typically 8 MHz or other frequencies depending on the MCU.
• HSE (High-Speed External) oscillator: This could be a crystal or an external clock source.
• PLL (Phase-Locked Loop) output: The PLL can generate higher frequencies by multiplying the input clock.
• SYSCLK: The system clock, which is the main clock driving the CPU and peripherals.
• LSI (Low-Speed Internal) oscillator: Typically used for low-power modes and watchdog timers.
• LSE (Low-Speed External) oscillator: Typically a 32.768 kHz crystal used for real-time clocks. - MCO Pins:
• The MCO signal is usually available on specific GPIO pins, such as MCO1 and MCO2, depending on the STM32 series.
• MCO1 and MCO2 are often located on different pins, allowing two different clock sources to be output simultaneously. - Configuration:
• MCO is configured via registers in the STM32’s clock control (RCC) peripheral.
• You can select the clock source and set the division factor (prescaler) to adjust the output frequency.
• For example, you might set the MCO1 pin to output the HSI clock divided by 4, giving you a 2 MHz output. - Common Applications:
• Clock Sharing: Providing a clock signal to other microcontrollers or devices in your system that need synchronization.
• Debugging: Verifying the clock configuration by measuring the MCO output with an oscilloscope.
• Synchronization: Outputting a stable clock source for peripherals that require precise timing.
2. Configuration:
Create new project with name of Verify_External_Clock. For how to create project, please follow this guide here.
After creating new project. In main.c file, include main STM32F4 header as following:
#include "stm32f4xx.h"
Next, we need to know which pins support MCO.
From STM32F411 datasheet:

We can see that PA8 supports MCO1, hence we shall use it.
First, enable clock access to GPIOA and configure PA8 in alternate function as following:
int main(void) { /*Enable Clock Access to GPIOA*/ RCC->AHB1ENR|=RCC_AHB1ENR_GPIOAEN; /*Set PA8 as alternate function*/ GPIOA->MODER|=GPIO_MODER_MODE8_1; GPIOA->MODER&=~GPIO_MODER_MODE8_0;
Next, we shall configure MCO as following:
- MCO output from HSE.
- Set prescaller to MCO to 5.

/*Set MCO1 to be HSE*/ RCC->CFGR|=RCC_CFGR_MCO1_1; /*Set prescaler to 5*/ RCC->CFGR|=(RCC_CFGR_MCO1PRE);
Finally, enable external oscillator as following:

/*Enable external oscillator*/ RCC->CR|=RCC_CR_HSEON; /*Wait until the external oscillator is enabled*/ while((RCC->CR & RCC_CR_HSERDY) !=RCC_CR_HSERDY);
hats it for the code.
Save the project, build it and run it on your STM32F411 Nucleo-64.

3. Results:
By probing PA8 using either logic analyzer or oscilloscope, you can see that the frequency is 1.6MHz. By multiplying by 5, we will get 8MHz which is the external frequency supplied by the embedded ST-Link.

Happy coding 😉
Add Comment