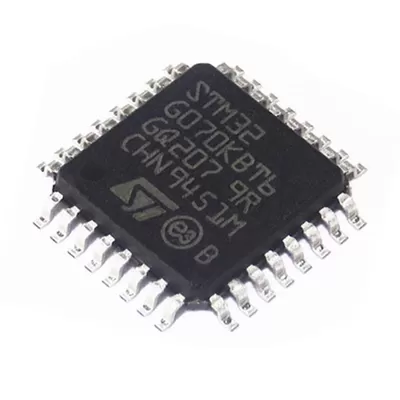
In this guide, we shall take a look at the UART protocol and how to setup UART using STM32CubeMX.
In this guide, we shall cover the following:
- What is UART.
- UART Setup using STM32CubeMX.
- Code.
- Results.
1. What is UART:
To transfer data, computers adopt two method: serial and parallel. In serial communication data is sent a bit at a time (1 line)while in parallel communication 8 or more lines are often used to transfer data. The UART is a form of serial communication. When distance is short, digital signal can be transferred as it is on a single wire and require no modulation. This is how PC keyboard transfer data between the keyboard and motherboard. For long distances like telephone lines, serial data communication requires a modem to modulate.We will not go into the details of this in this lesson, this shall be left for subsequent lessons.
Synchronous vs. Asynchronous
Serial communication operate in two ways : synchronous and asynchronous. In the synchronous mode, a block of data is sent at a time while in the asynchronous mode, a single byte of data is sent at a time. It is possible to write code to provide both the synchronous and asynchronous serial communication functions however this could turn out tedious and over complicated therefore, special chips are manufactured to perform these functions. When these chips are added to a microcontroller, they become known as Serial Communication Interface (SCI). The chip that provides the the asynchronous communication (UART) is the main theme of this lesson. The chip for synchronous communication is known as the USART and shall left for another lesson.
Asynchronous Communication Data Framing
The data is received in zeros and ones format, in order to make sense of the data, the sender and the receiver must agree on a set of rules i.e. a protocol, on how data is packed, how many bits constitute a character and when data begins and ends.
The Protocol
- Start and Stop bits
Asynchronous serial communication is widely used in character transfer. Each character is packed between start and stop bits. This is known as the framing.
start bit : Always 1 bit, value is always 0
stop bit: 1 or 2 bits, value is always 1
Lets take an example. Lets say we are transferring ASCII character ‘A’
its binary code is 0100 0001 and 0x41 in hex.Furthermore, it is framed between a start bit and 2 stop bits. This is what its frame will look like.
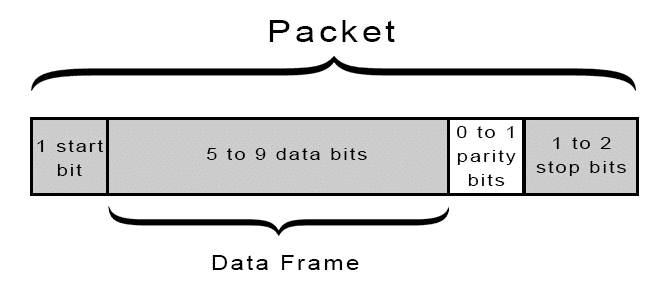
2. Parity bit
Some systems require parity bit in order to maintain data integrity.The parity bit of a character byte is included in the data frame after the stop bit(s).
3.Rate of data transfer : Baud rate/bps
We shall explain this concept with an example. Lets say we are asked to calculate the number of bits used and time taken in transferring 50 pages of text, each with 80×25 characters. Assuming 8 bits per character and 1 stop bit
solution
For each character a total of 10 bits is used ( 1 start bit, 8 bits character, 1 stop bit).
Therefore total number of bits = 80 x 25 x10 = 20,000 bits per page
50 pages implies, 50×20,000 = 1,000,000 bits.
Therefore it will take 1 million bits to transfer this information.
The time it takes to transfer the entire data using
a. 9600 baudrate implies, 1,000,000 / 9600 = 204 seconds
b. 57,600 baudrate implies 1,000,000 /57,600 = 17 seconds
Baudrate simply means the transfer of bits per second. There are various standardized baudrate we can choose from when we program our serial communication devices. The key thing is, both communication devices must have the same baudrate. We shall see this later on in our example code.
2. UART Setup in STM32CubeMX:
Open STM32CubeIDE and create new project and name it UART. For more information how to setup the project using STM32CubeIDE, please refer to this guide.
After that, Open connectivity and enable UART2 as following:
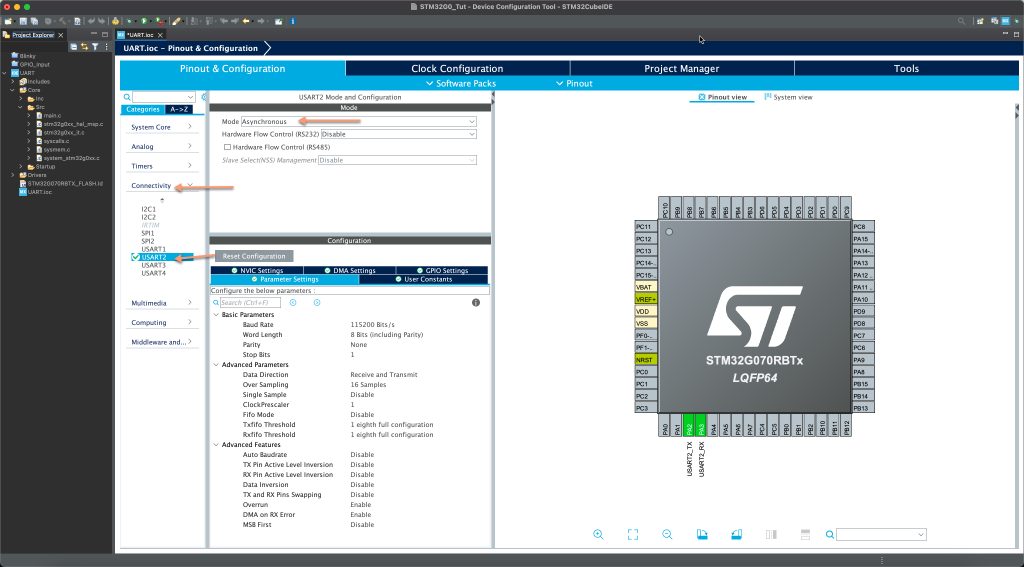
Set the mode to be Asynchronous and leave the parameter to default values.
Yo will notice that PA2 and PA3 has been enabled automatically.
PA2 is the UART TX while PA3 is UART RX.
Now, save the project and this will generate the code.
3. Code:
Now within USER CODE BEGIN Includes, include the stdio.h header as following:
#include "stdio.h"
Now, within USER CODE BEGIN PV, declare an array to hold the characters to be transmitted as following:
char uart_data[100];
Also, declare the counter value which will be incremented in the while loop as following:
uint32_t counter;
In USER CODE BEGIN 3, declare a new variable called len as following:
uint32_t len;
This will hold the length of the string to be transmitted.
Now, we shall use sprintf to create character array to be transmitted over UART as following:
len = sprintf(uart_data,"Counter value is %d\r\n",counter++);
Since sprintf returns number of characters, we shall use it.
Next, we shall transmit data as following:
HAL_UART_Transmit(&huart2, uart_data, len, 100);
The function HAL_UART_Transmit will transmit data over uart using blocking mode.
The parameters of the function as following:
- UART handle typedef is which UART to be used (UART2 in this case).
- Pointer to the data array to be transmitted.
- Length of the data to be transmitted.
- Time out which if the function spend more time that the specified, it will return time out.
The function shall return one of these three:
- HAL_OK means everything has been transmitted.
- HAL_ERROR, an error occurred like invalid parameter etc.
- HAL_BUSY, the peripheral is currently occupied.
- HAL_TIMEOUT means the function took longer than expected and exited.
Finally, delay by 100ms as following:
HAL_Delay(100);
4. Results:
Run the code on your STM32G0x0 and select the baudrate to be 115200, you should get the following:
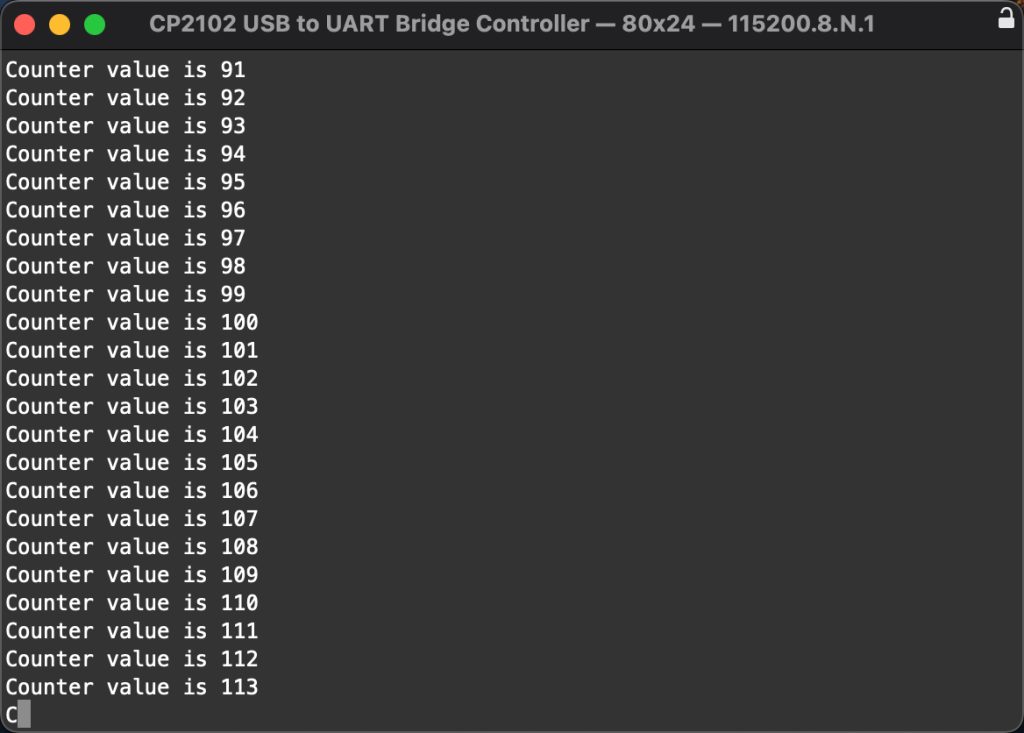
4 Comments
Dear Sir,
Good Morning,
Please let me know IN your projects published where SPI, I2C, UART, CAN using
Interrupts (Enable/Disable, Clear, Status, Masked/unMasked, Registered/UnRegisted)
thanks with regards,
HSR-Rao.
Hi,
It will be mentioned in the title.
Dear Sir,
Requesting you,
While Explaining, the Theory part, Please explain, Exactly, the difference between,
(1) Polling / NonPolling
(2) Blocking / NonBlocking
(3) Need & Why Interrupts are inevitable.
this will Help us to understand in depth.
thanks with regards,
HSR-Rao.
(3)
Will do.
Add Comment