
In the previous guide (here), we saw how to setup Nextion editor and STM32F4 to work with Nextion display. In this guide, we shall see how to send some strings from STM32 to Nextion display.
In this guide, we will cover the following:
- Nextion Display setup
- STM32F4 setup.
- Demo.
1. Nextion Display Setup:
We start of by opening Nextion editor. Then, File, New:
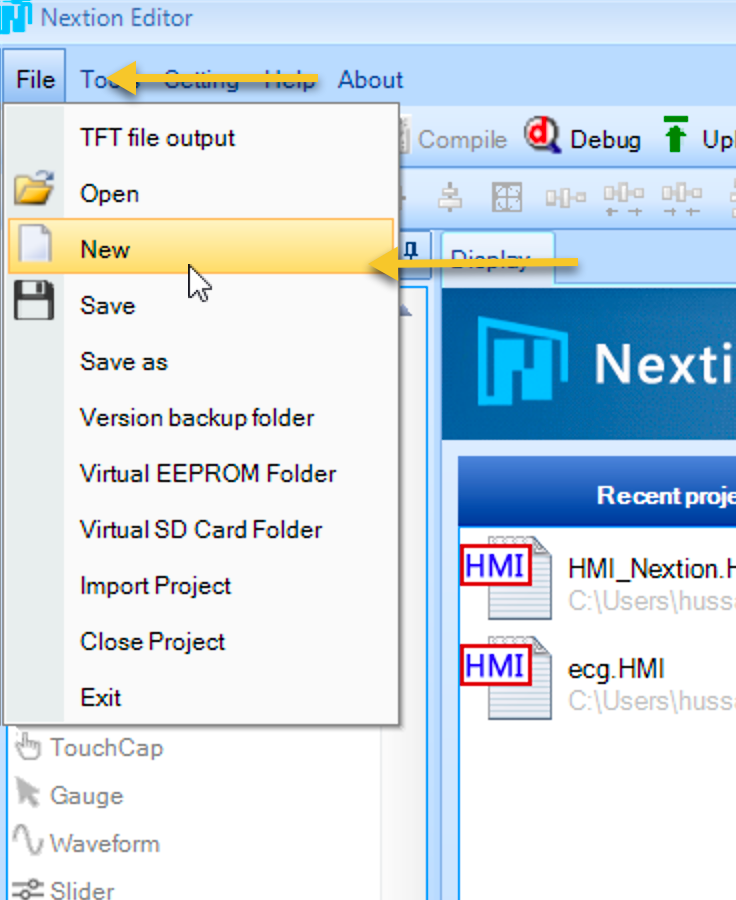
Then give the project a name.
After that, select your model (mine is NX3224K024_011):
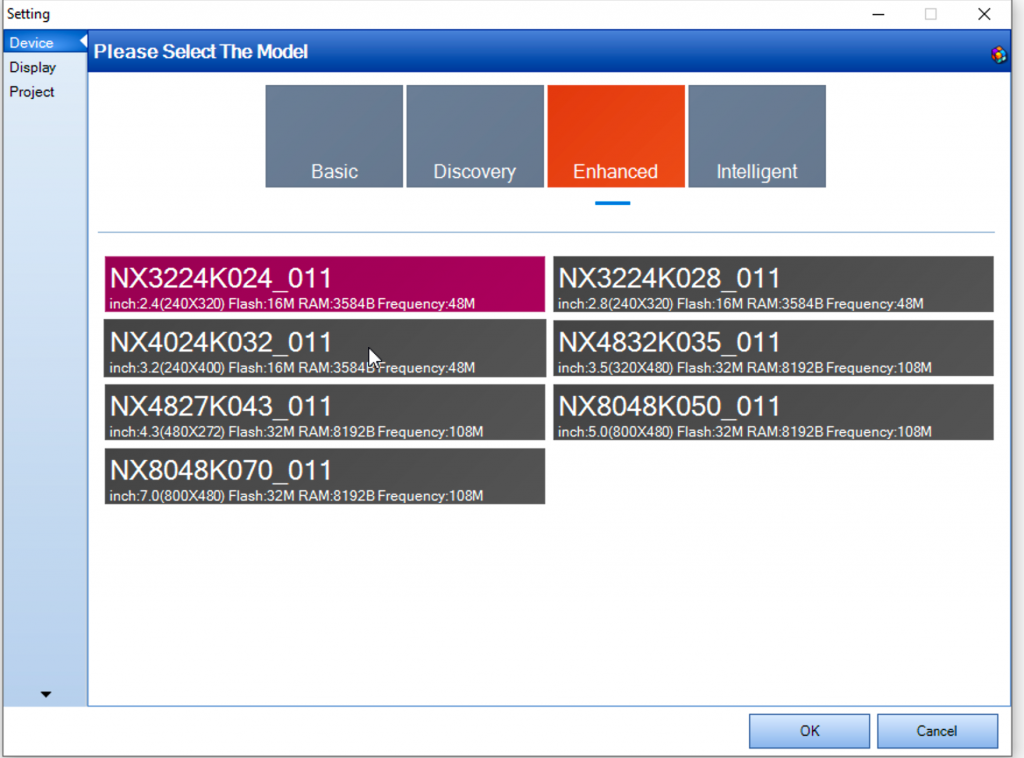
Select the direction:
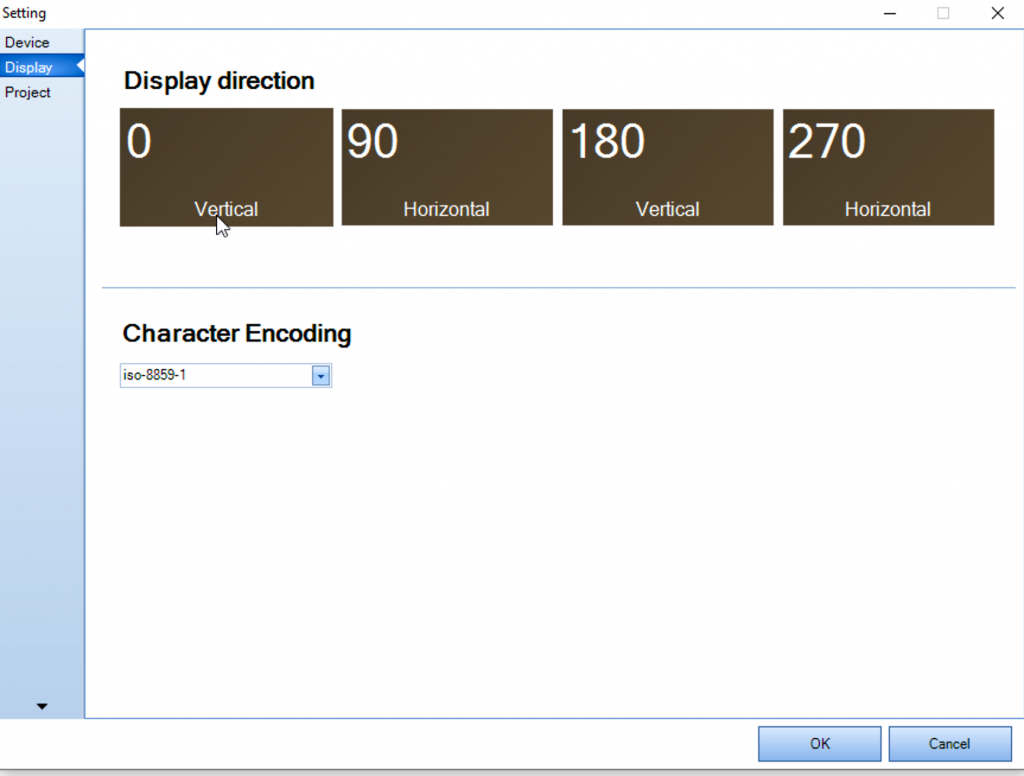
In my case, horizontal 90.
Finally press OK.
After that, you will be greeted by empty project.
From left toolbox, select text:
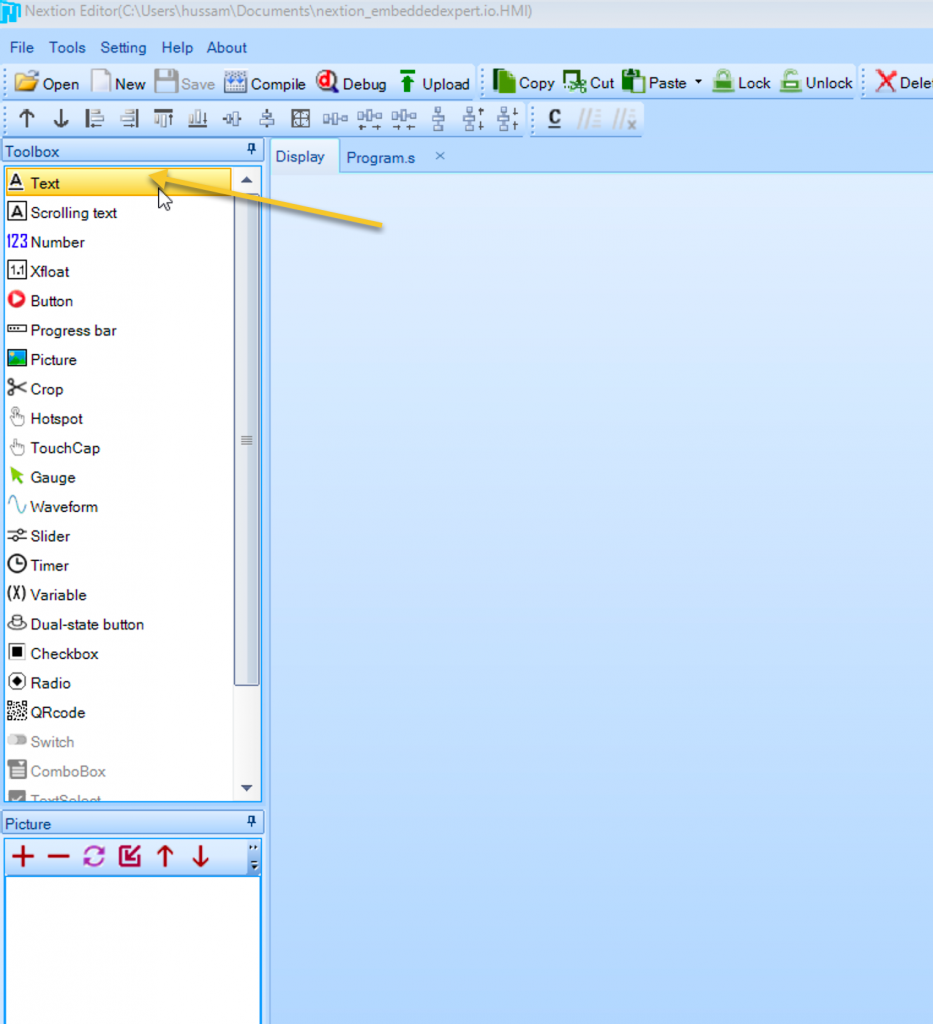
Resize the text size according to your screen.
From right side, attribute, note the objname and set txt_maxl to 30:
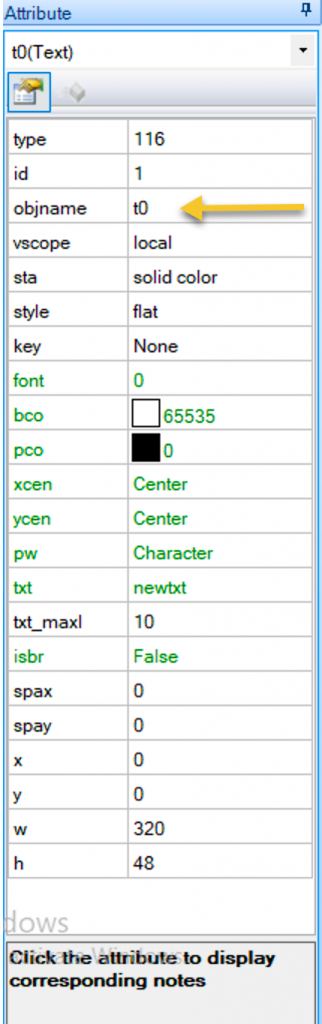
Then, we need to generate fonts as following:
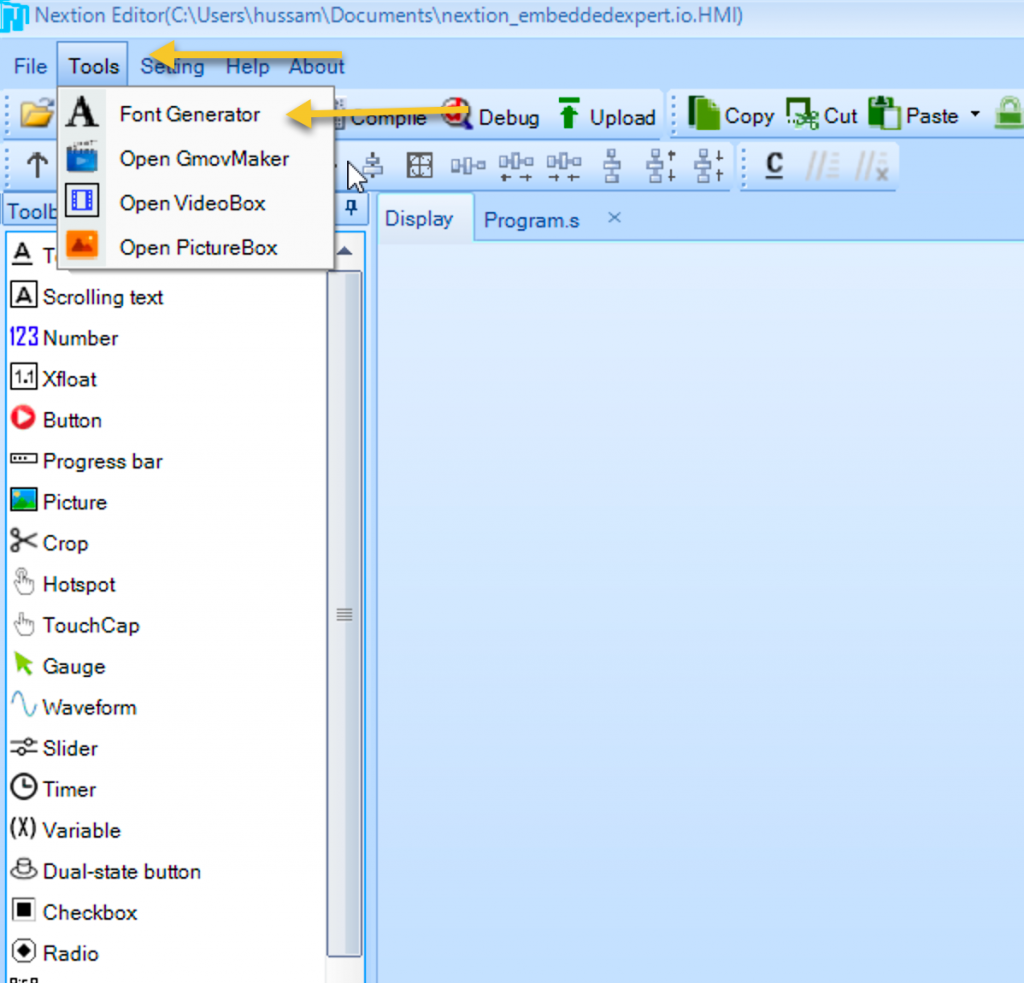
Select the type of font you like and give a name to the font:
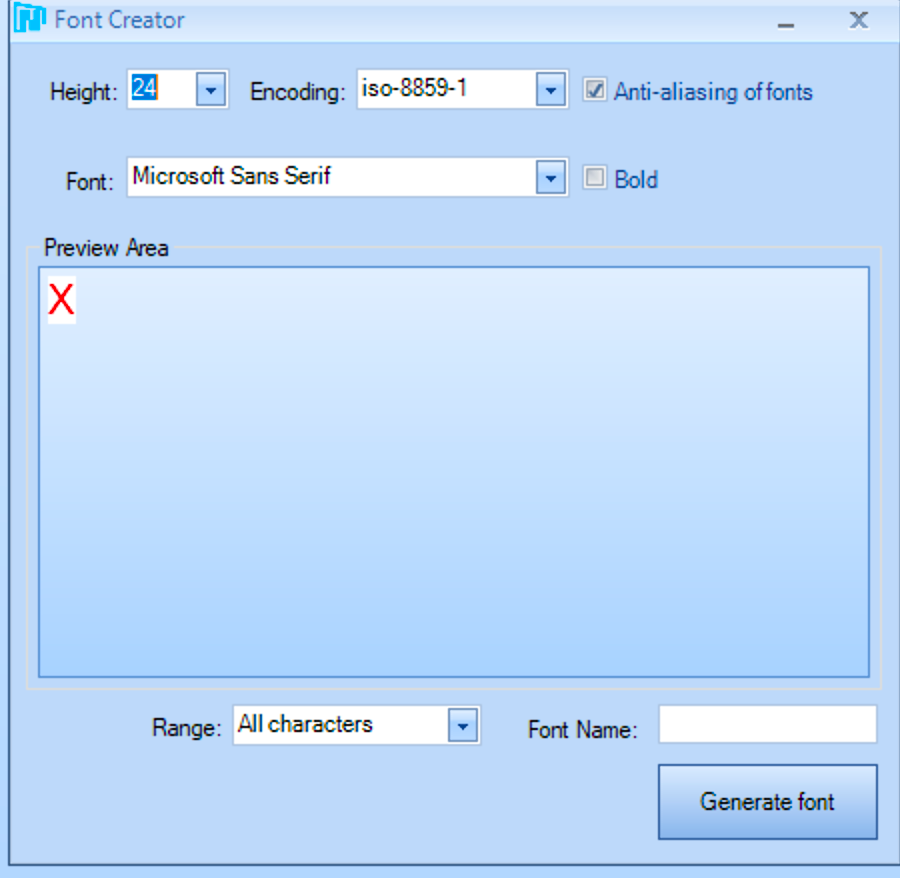
Press Generate font and save the file
After that, press yes.
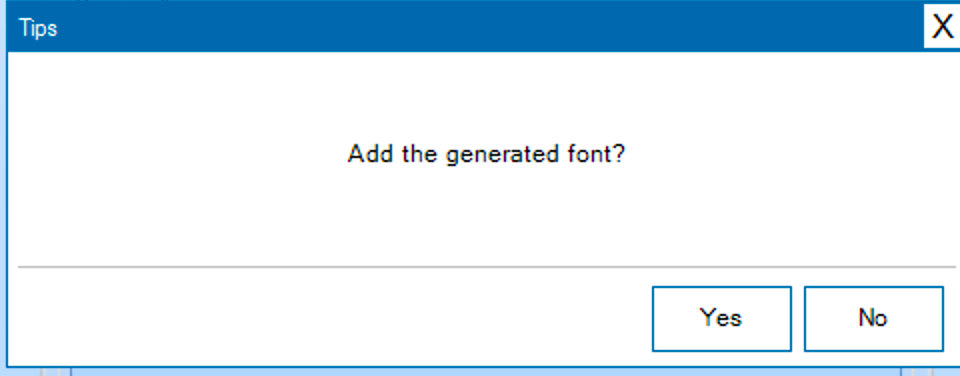
Now you good to go.
After that, press on debug to see the result:
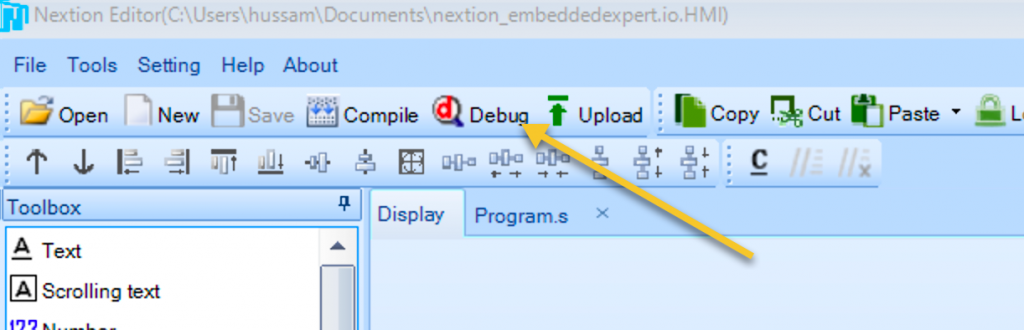
In String area, type the following and press enter:
t0.txt="hello"
You should see something similar:
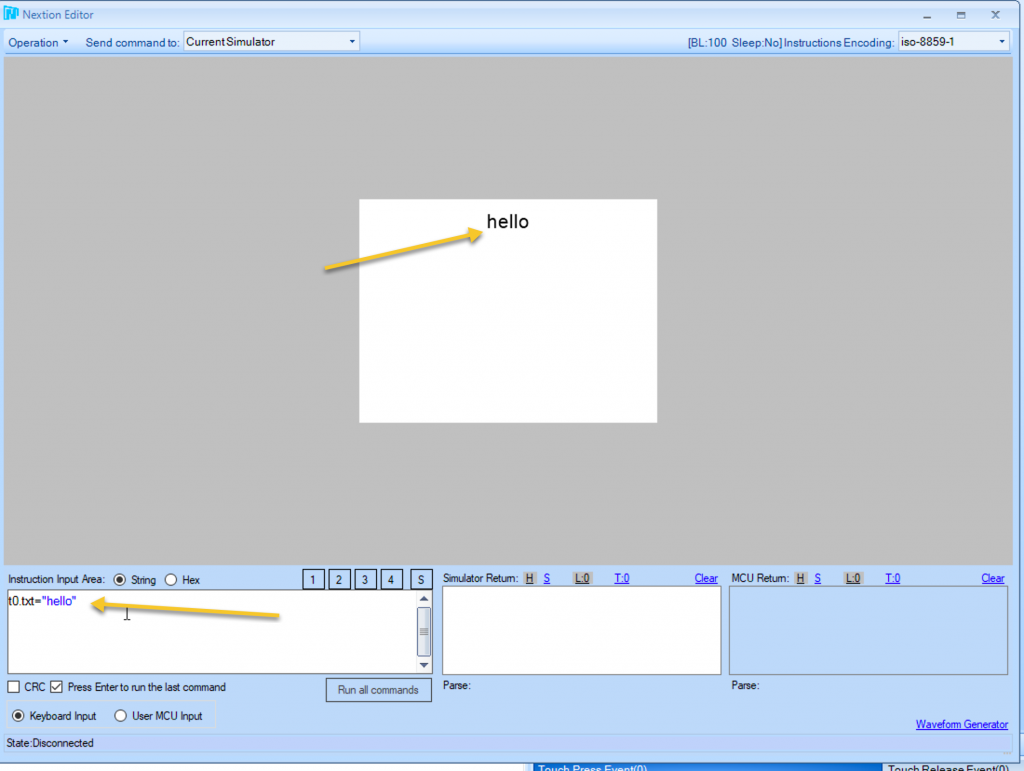
Now, press on hex:
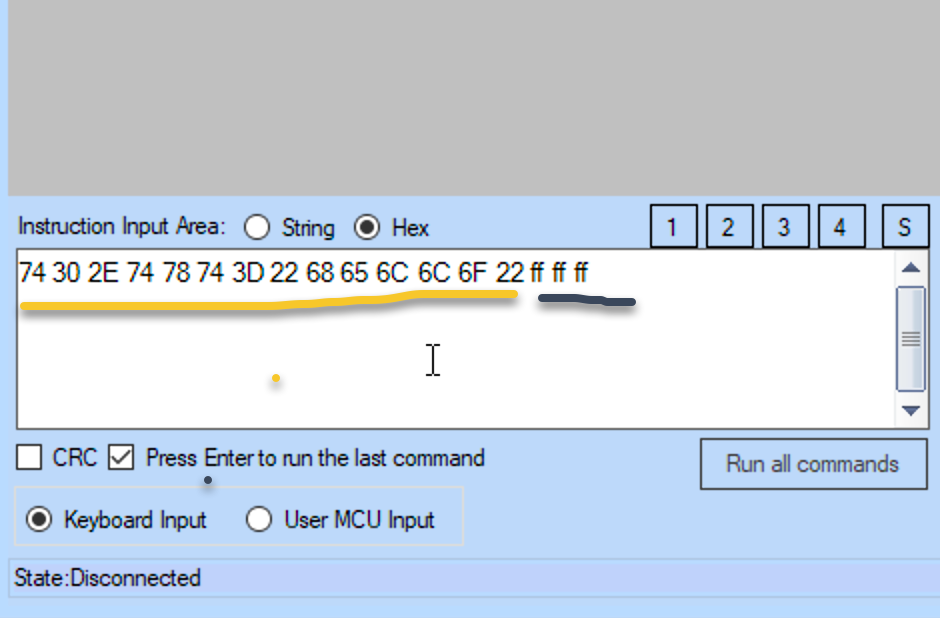
You will get the equivalent hex format to t0.txt=”hello” and extra 3 0xFF which indicate the end of the command. This shall be sent at the end of each string in our code.
Now, insert an SD-Card to your computer and generate TFT file to load it to our display:
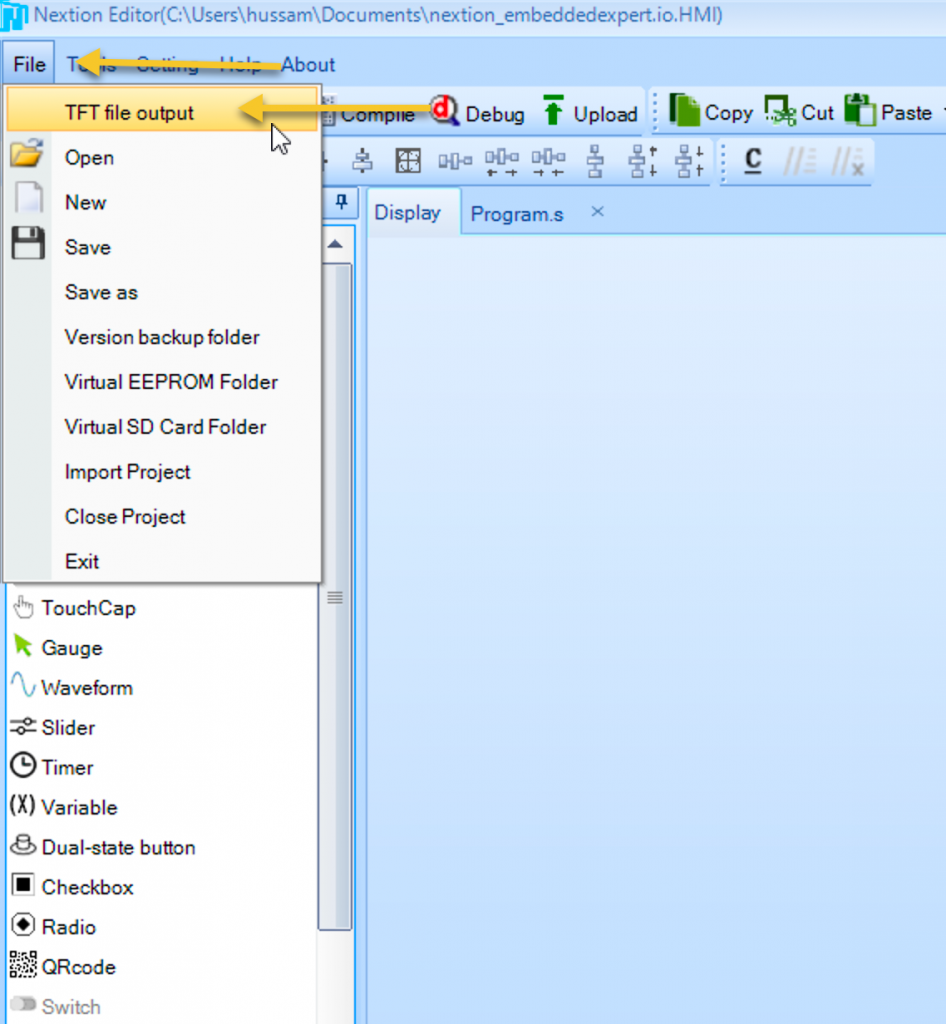
After that, select your SD-Card:
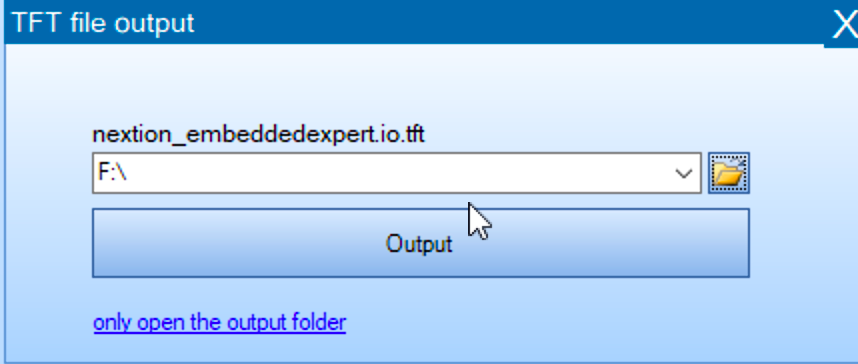
Press on output to copy the file to SD-Card.
Now, insert the SD-Card into your Nextion display:

You should see a message on the display saying the code is being uploaded.

When it is ready, it should display the following message:

Remove the power from the Nextion display, and unplug the microSD card.
After than, you should see newtxt is being displayed on the screen. This is the end of step one.
2. STM32F4 Setup:
The function that will send the string to Nextion display shall take two arguments:
- First one character pointer to the id.
- Second one character pointer to the string to be sent.
void nextion_write_text(char *id,char * p)
Inside the function, we declare character array of 5 to hold the data:
char buf[50];
Then using sprintf, we shall combine the id and the string into single character array:
sprintf(buf,"%s.txt=\"%s\"",id,p);
Send the buffer:
send_uart(buf);
Write the 3 0xFF:
uart1_write(0xFF); uart1_write(0xFF); uart1_write(0xFF);
Hence, the entire function as following:
void nextion_write_text(char *id,char * p) { char buf[50]; sprintf(buf,"%s.txt=\"%s\"",id,p); send_uart(buf); uart1_write(0xFF); uart1_write(0xFF); uart1_write(0xFF); }
In main function:
#include "delay.h" #include "string.h" #include "nextion_uart.h" /*Nextion Buffer size*/ #define NEXTION_BUFF_LENG 10 volatile char NEXTION_BUFF[NEXTION_BUFF_LENG]; volatile uint32_t itr; char data_send[10]; uint8_t counter; int main(void) { SCB->CPACR |= ((3UL << 10*2)|(3UL << 11*2)); systick_init_ms(16000000); nextion_uar_init(115200,16000000); while(1) { sprintf(data_send,"Count=%d",counter++); nextion_write_text("t0",data_send); delay(100); } }
Note: my Nextion display works with 115200 baudrate.
3. Demo:
Happy coding 🙂
Add Comment