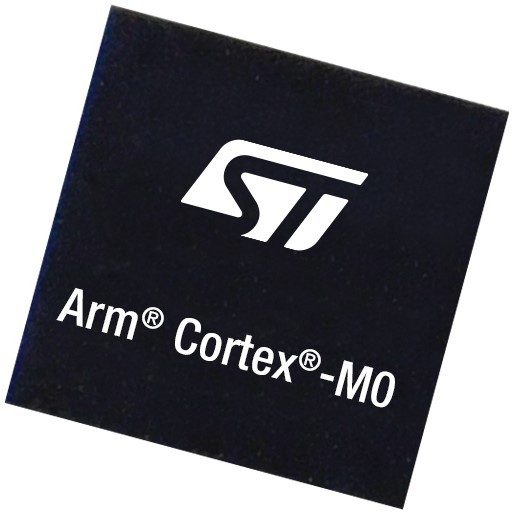
In the previous guide (here), we took a look how to configure the UART of STM32L051 in transmit mode. Now we shall see how to configure the UART to receive data and echo back the received data (Transmit receive mode).
In this guide, we shall cover the following:
- Configure the UART in RX mode.
- Code.
- Demo.
1. Configure the UART in RX mode:
From the previous guide, we noticed that PA2 and PA3 are connected to UART of ST-Link and since PA2 and PA3 are USART2 of STM32L053, we can configure the PA2 to work in alternate function as following:
GPIOA->MODER|=GPIO_MODER_MODE3_1; GPIOA->MODER&=~GPIO_MODER_MODE3_0;
Then we can select which alternate function as following:
#define AF04 0x04 GPIOA->AFR[0]|=(AF04<<12);
Now we can enable the RX part in CR1 of USART2:
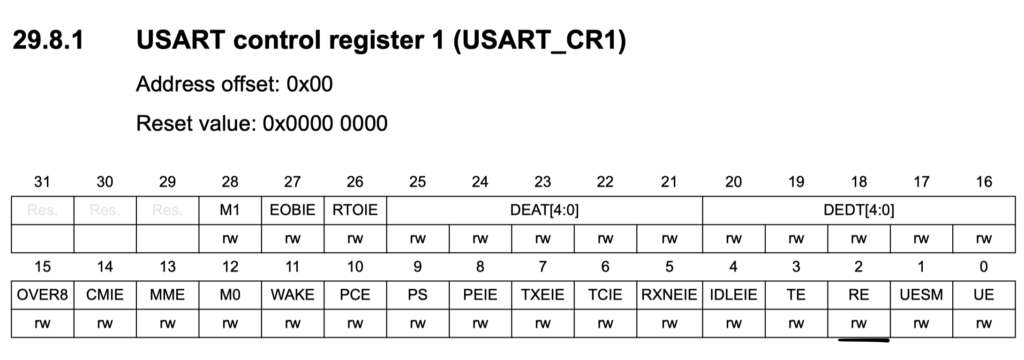
USART2->CR1 = USART_CR1_TE|USART_CR1_RE; /*TE from previous guide*/
And finally, enable UART
/*Enable uart module*/ USART2->CR1 |= USART_CR1_UE;
Now we need to check if there is new data as following:
We can check the RXNE (Read data register not empty) bit in USART interrupt and status register (USART_ISR)
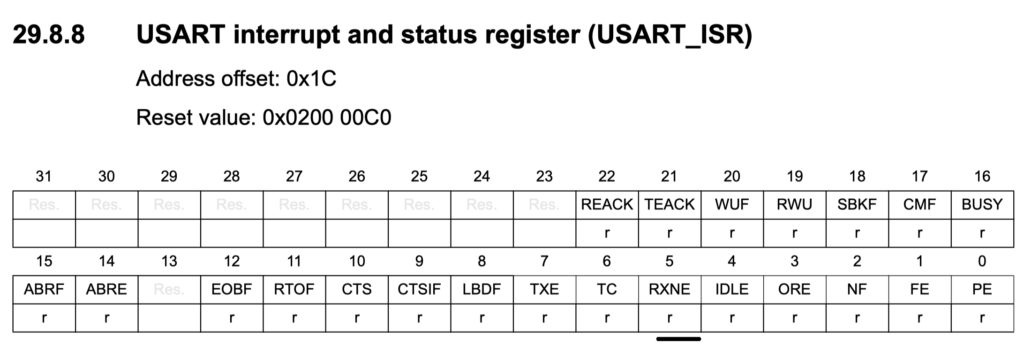
We can create a function that allows us to check this bit and return 1 if the receiver buffer is not empty as following:
int8_t uart2_data_available(void) { /*Wait for char to arrive*/ if((USART2->ISR & USART_ISR_RXNE)){return 1;} else return 0; }
And finally a function that allows us to read the content of the UART receiver buffer:
char uart2_read(void) { return(USART2->RDR); }
2. Code:
In the main.c file, we can check for the data is available and echo back the received character:
#include "uart.h" int main(void) { uart_init(); while(1) { if(uart2_data_available()==1) { uart2_write(uart2_read()); uart2_write('\r');uart2_write('\n'); } } }
You may download the source code from here:
3. Demo:
Happy coding 🙂
Add Comment