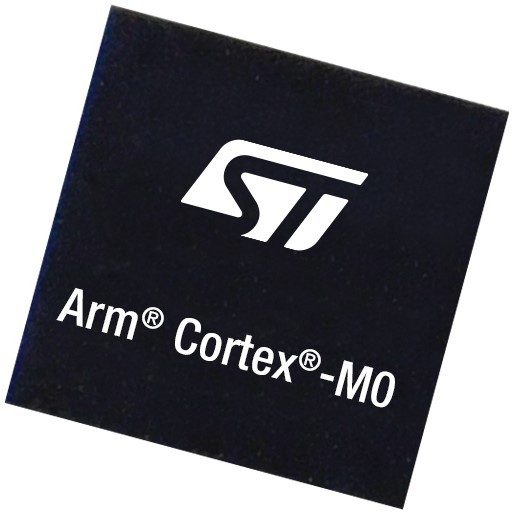
In te previous guide (here), we took a look how to configure the GPIO as input. In this guide, we shall cover how to use UART in STM32L051 to send a single character.
In this cover, we will cover the following:
- UART.
- Configuring pins to work in UART mode.
- Configure the UART.
- Send character.
- Code.
- Results.
1. UART:
To transfer data, computers adopt two method: serial and parallel. In serial communication data is sent a bit at a time (1 line)while in parallel communication 8 or more lines are often used to transfer data. The UART is a form of serial communication. When distance is short, digital signal can be transferred as it is on a single wire and require no modulation. This is how PC keyboard transfer data between the keyboard and motherboard. For long distances like telephone lines, serial data communication requires a modem to modulate.We will not go into the details of this in this lesson, this shall be left for subsequent lessons.
Synchronous vs. Asynchronous
Serial communication operate in two ways : synchronous and asynchronous. In the synchronous mode, a block of data is sent at a time while in the asynchronous mode, a single byte of data is sent at a time. It is possible to write code to provide both the synchronous and asynchronous serial communication functions however this could turn out tedious and over complicated therefore, special chips are manufactured to perform these functions. When these chips are added to a microcontroller, they become known as Serial Communication Interface (SCI). The chip that provides the the asynchronous communication (UART) is the main theme of this lesson. The chip for synchronous communication is known as the USART and shall left for another lesson.
Asynchronous Communication Data Framing
The data is received in zeros and ones format, in order to make sense of the data, the sender and the receiver must agree on a set of rules i.e. a protocol, on how data is packed, how many bits constitute a character and when data begins and ends.
The Protocol
- Start and Stop bits
Asynchronous serial communication is widely used in character transfer. Each character is packed between start and stop bits. This is known as the framing.
start bit : Always 1 bit, value is always 0
stop bit: 1 or 2 bits, value is always 1
Lets take an example. Lets say we are transferring ASCII character ‘A’
its binary code is 0100 0001 and 0x41 in hex.Furthermore, it is framed between a start bit and 2 stop bits. This is what its frame will look like.
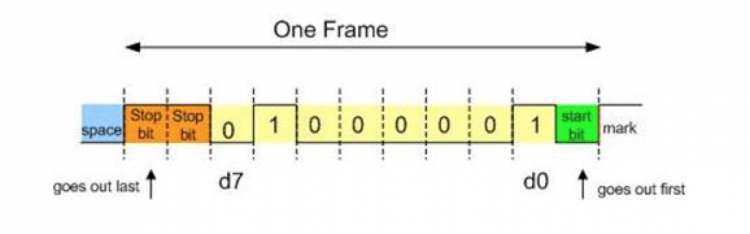
2. Parity bit
Some systems require parity bit in order to maintain data integrity.The parity bit of a character byte is included in the data frame after the stop bit(s).
3.Rate of data transfer : Baud rate/bps
We shall explain this concept with an example. Lets say we are asked to calculate the number of bits used and time taken in transferring 50 pages of text, each with 80×25 characters. Assuming 8 bits per character and 1 stop bit
solution
For each character a total of 10 bits is used ( 1 start bit, 8 bits character, 1 stop bit).
Therefore total number of bits = 80 x 25 x10 = 20,000 bits per page
50 pages implies, 50×20,000 = 1,000,000 bits.
Therefore it will take 1 million bits to transfer this information.
The time it takes to transfer the entire data using
a. 9600 baudrate implies, 1,000,000 / 9600 = 204 seconds
b. 57,600 baudrate implies 1,000,000 /57,600 = 17 seconds
Baudrate simply means the transfer of bits per second. There are various standardized baudrate we can choose from when we program our serial communication devices. The key thing is, both communication devices must have the same baudrate. We shall see this later on in our example code.
2. Configuring the pins in UART Mode:
In order to set the pin in UART, we need to figure out which pins of ST-Link V2 on Nucleo-L053 is connected.
From the user manual of Nucleo-64 UM1724, we can find that PA2 and PA3 are connected to UART2 of STM32L053.
Hence we start off by enabling clock access to GPIOA as following:
/*Enable clock access to GPIOC*/ RCC->IOPENR |= RCC_IOPENR_GPIOAEN;
Since PA2 is the TX pin, we need to set it to alternative mode:
Since the default mode is analog mode, we need to set bit 5 and reset bit4
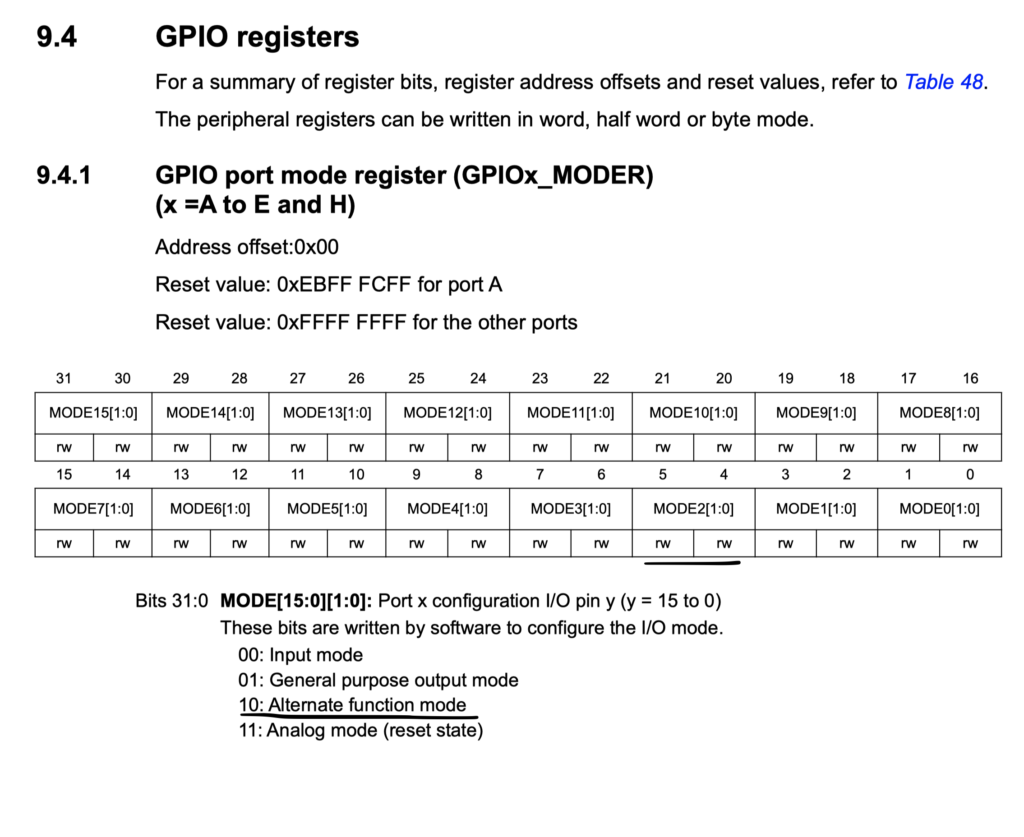
GPIOA->MODER|=GPIO_MODER_MODE2_1; GPIOA->MODER&=~GPIO_MODER_MODE2_0;
Then we need to find which alternate function is related to USART2:
We can find this information from datasheet of STM32L053 table 16.
The Required AF is AF04, hence we can create macro for that:
#define AF04 0x04
hence we can configure the PA2 function in AFR[0]
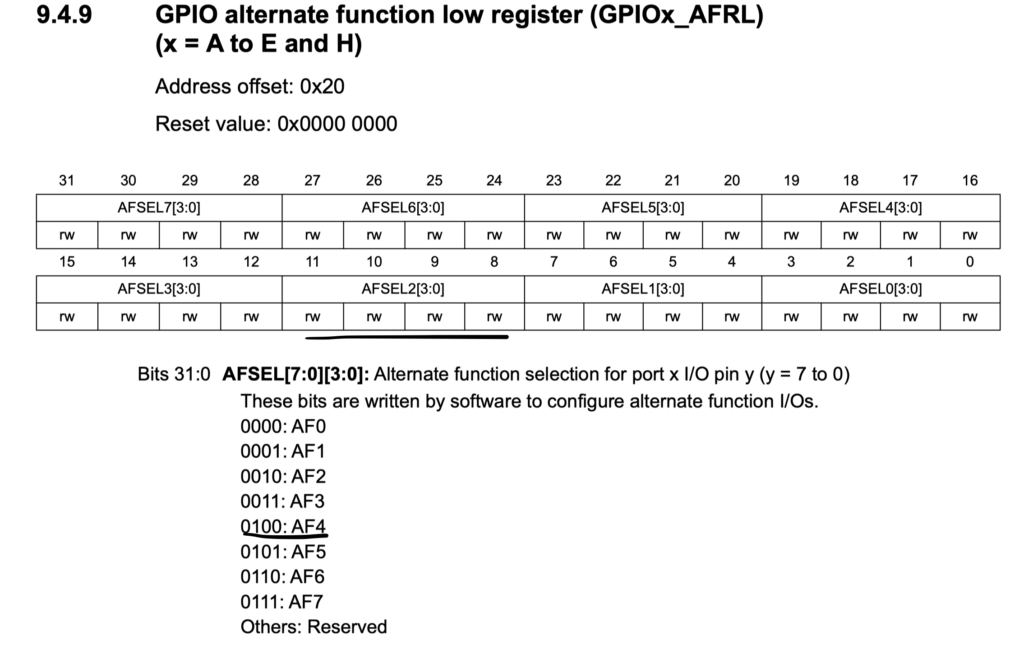
GPIOA->AFR[0]|=(AF04<<8);
Thats all for the GPIO.
3. Configure the UART:
First we enable clock access to UART2:
UART2 is connected to APB1:
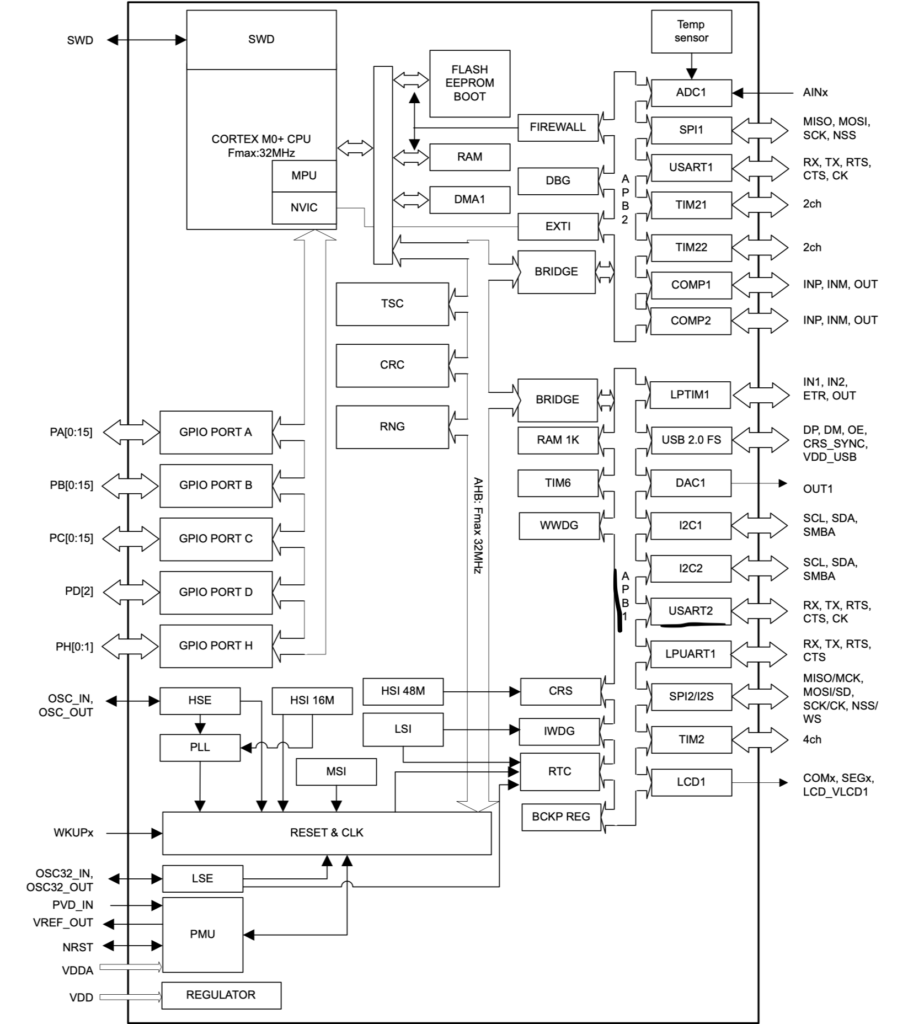
RCC->APB1ENR |= RCC_APB1ENR_USART2EN;
Then we need to create a function that allows us to configure the baudrate as following:
static uint16_t compute_uart_bd(uint32_t PeriphClk, uint32_t BaudRate) { return ((PeriphClk + (BaudRate/2U))/BaudRate); }
Hence we can set the baudrate of UART2 as following:
USART2->BRR=compute_uart_bd(APB1_CLK,UART_BAUDRATE);
By default, STM32L053 is running at 2.09MHz, hence we can create symbolic name as following:
#define SYS_FREQ 2097000 #define APB1_CLK SYS_FREQ #define UART_BAUDRATE 9600
Then we enable TX and Direction as following:
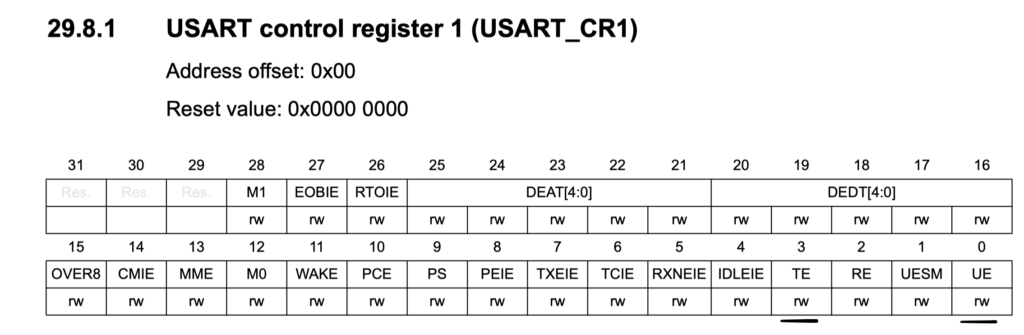
/*Configure transfer direction*/ USART2->CR1 = USART_CR1_TE; /*Enable uart module*/ USART2->CR1 |= USART_CR1_UE;
That’s all for configuring the uart.
4. Transmit single character:
In order to send a single character, we need to wait until the bure is empty and transmit the data as following:
void uart2_write(int ch) { /*Make sure transmit data register is empty*/ while(!(USART2->ISR & USART_ISR_TXE)){} /*Write to the transmit data register*/ USART2->TDR = (ch & 0xFF); }
5. Code:
You may download the code from here:
6. Results:
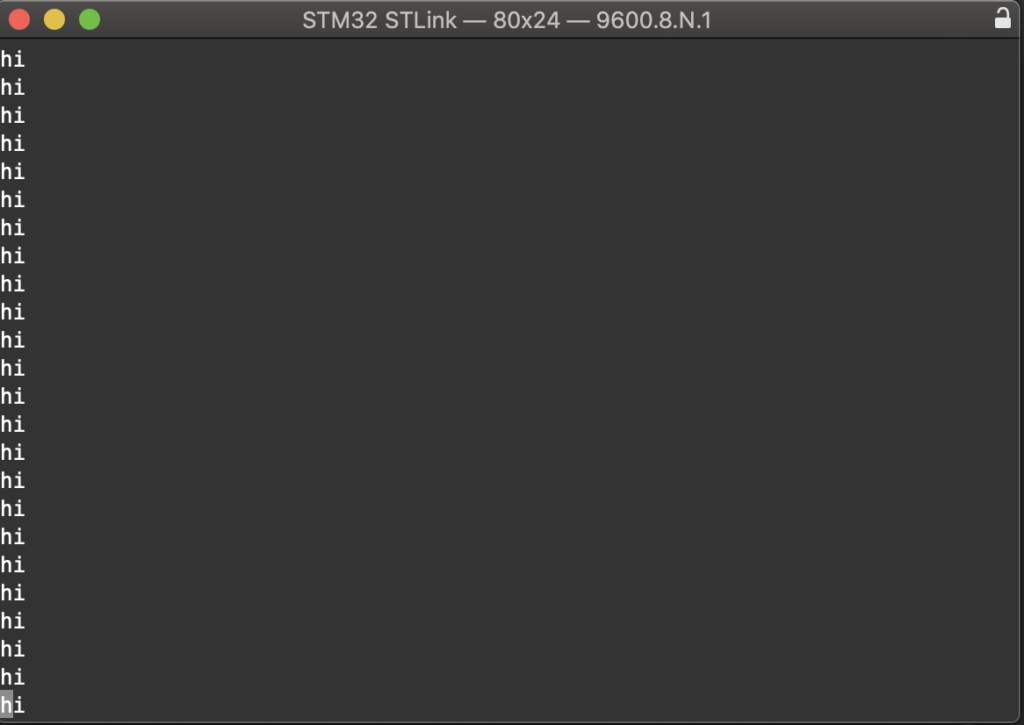
Happy coding 🙂
Add Comment