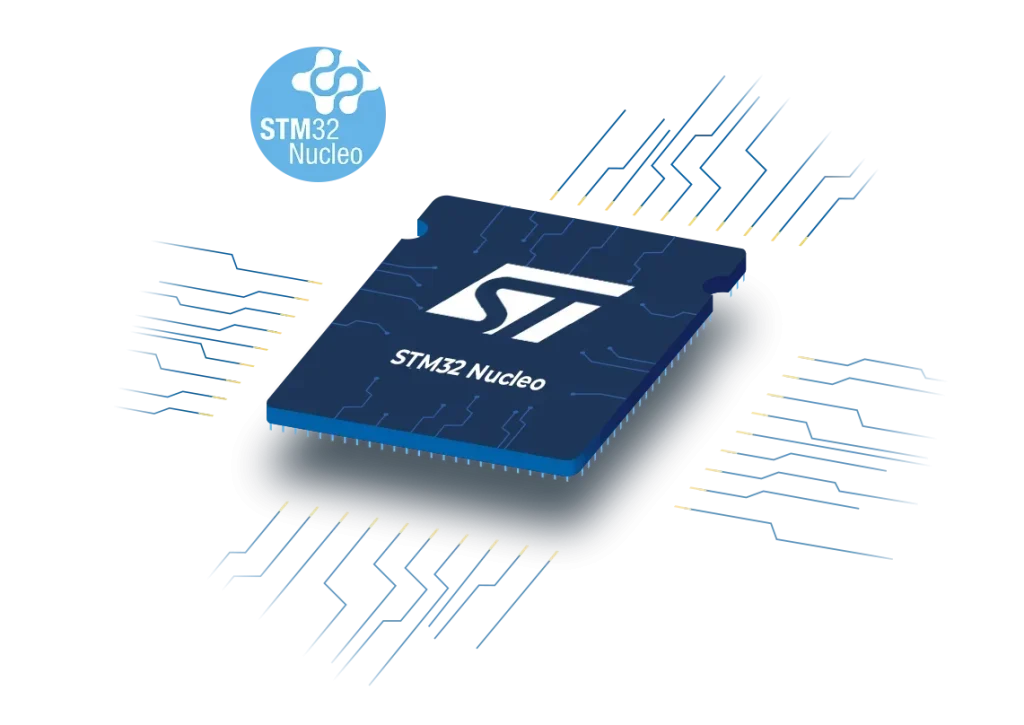
In the first part of timer series on STM32H5, we shall use the timer to generate PWM signal to control the brightness of the LED connected to one of the timer channels.
In this guide, we shall cover the following:
- What is PWM.
- STM32CubeMX Setup.
- Driver development.
- Results.
1. What is PWM:
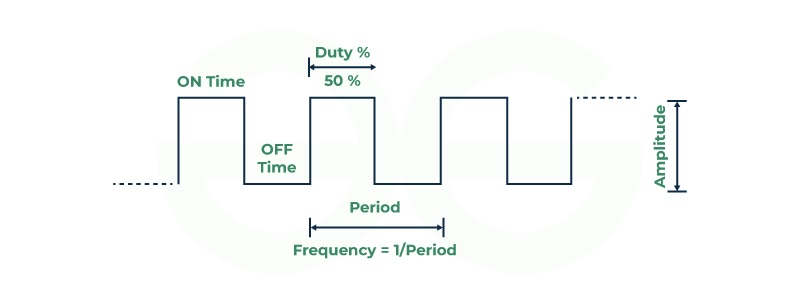
Pulse-width modulation (PWM), also known as pulse-duration modulation (PDM) or pulse-length modulation (PLM), is a technique used to represent a signal as a rectangular wave with a varying duty cycle (and sometimes a varying period). Let’s break it down:
- Duty Cycle: The duty cycle refers to the ratio of the time the signal is “on” (high) to the total time of one complete cycle. It is expressed as a percentage. For example, if a signal is on for 60% of the time and off for 40%, the duty cycle is 60%.
- Average Power Control: PWM is particularly useful for controlling the average power or amplitude delivered by an electrical signal. By switching the supply between 0% and 100% at a rate faster than the load can respond, we can effectively control the average power delivered to the load.
- Applications:
- Motor Control: PWM is commonly used to control motors (such as in fans, pumps, and robotics) because motors are not easily affected by discrete switching.
- Solar Panels: It’s a key method for controlling the output of solar panels to match battery requirements.
- Digital Controls: PWM works well with digital controls, allowing precise duty cycle adjustments.
- Communication Systems: In some cases, PWM duty cycles have been used to convey information over communication channels.
- Switching Frequency: The switching frequency (how often the signal switches on and off) varies depending on the application. It can range from several times a minute (e.g., electric stoves) to tens or hundreds of kilohertz (e.g., audio amplifiers).
- Advantages:
- Low power loss in switching devices (almost zero current when off).
- Works well with digital control systems.
- Used in various applications due to its flexibility.
- Disadvantages:
- Choosing the right switching frequency is crucial for smooth control.
- Too high a frequency can stress mechanical components.
- Too low a frequency can cause load oscillations.
In summary, PWM allows precise control of average power delivery by rapidly switching the signal on and off.
2. STM32CubeMX Configuration:
We start off by creating new project with name of PWM. For how to create project using STM32CubeIDE, please refer to this guide here.
Since STM32H563Zi Nucleo-144 has arduino pinout, we can use that.
From the user guide of STM32H563ZI Nucleo-144 we can find that A0 of Arduino pin is connected to PA6 as following:
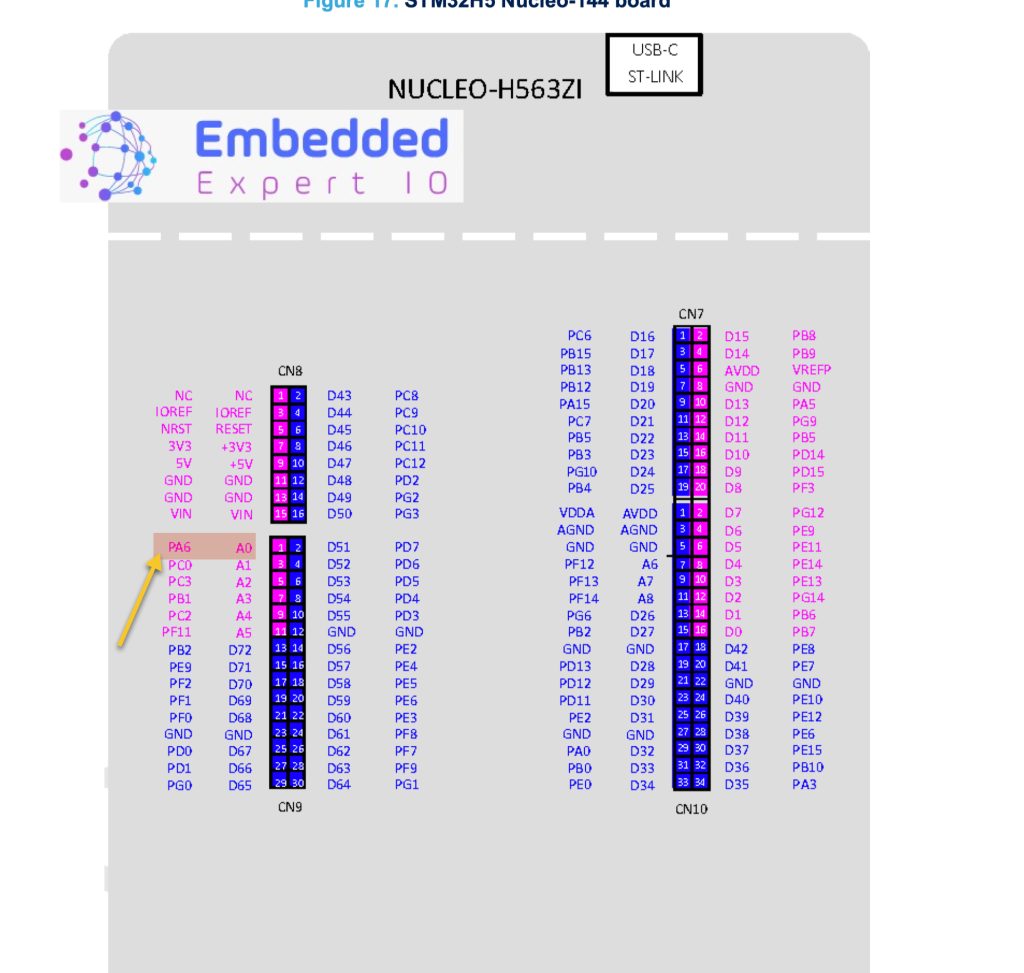
Hence we shall connect external LED through 220Ohm resistor to limit the current as following:
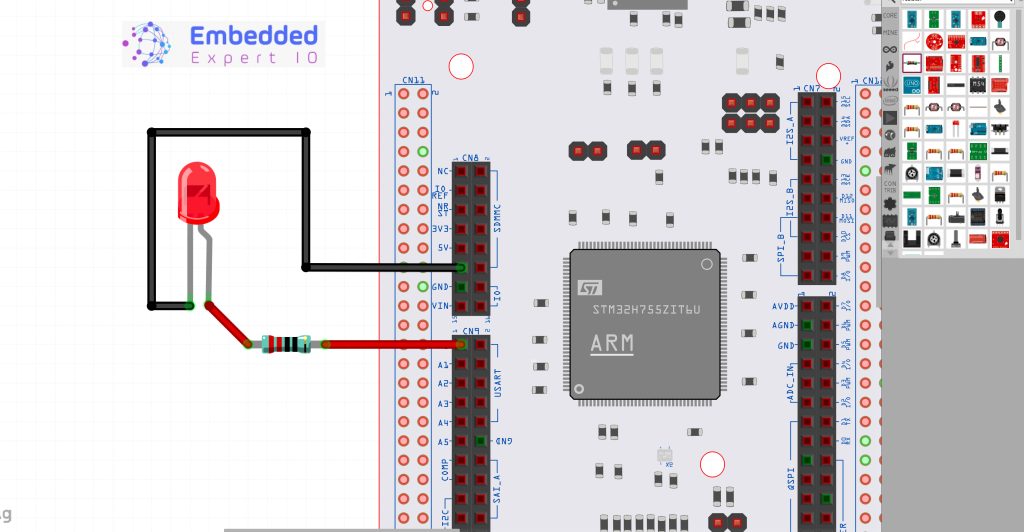
From STM32CubeIDE enable PA6 as TIM3_CH1 as following:
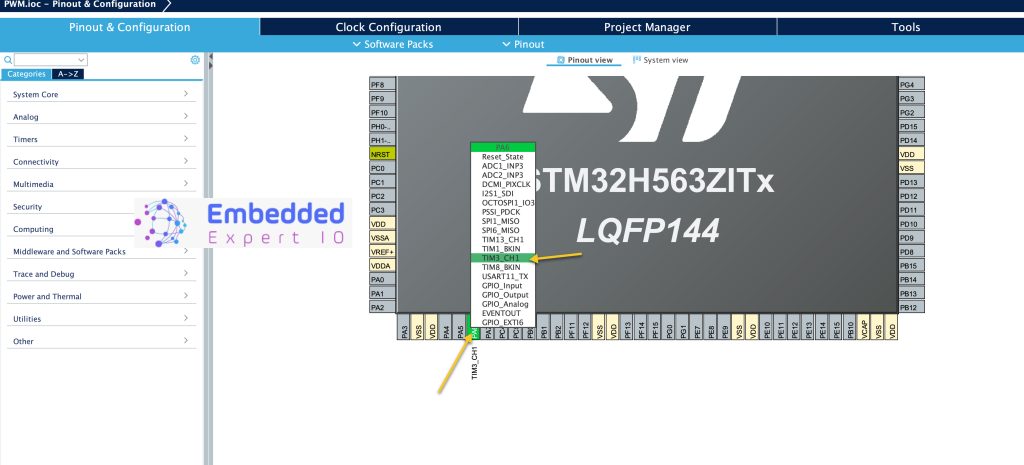
Next, from timers section, enable TIM3 as following:
- Set the clock source to be internal.
- Enable Channel 1 to be in PWM mode as following:
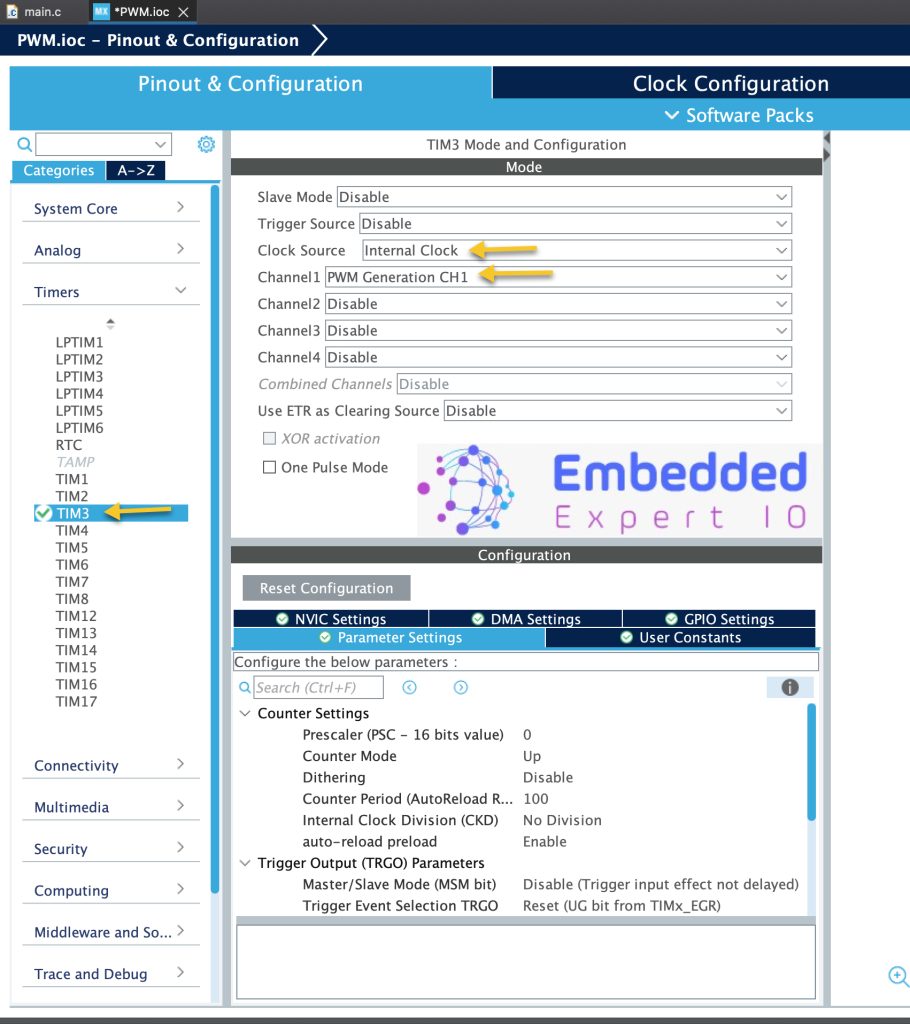
Next, from the Parameters settings:
- Set the counter value to be 100 (maximum counter shall count).
- Set the Mode to be PWM mode 1 for PWM Generation channel.
- Enable Auto-reload preload.
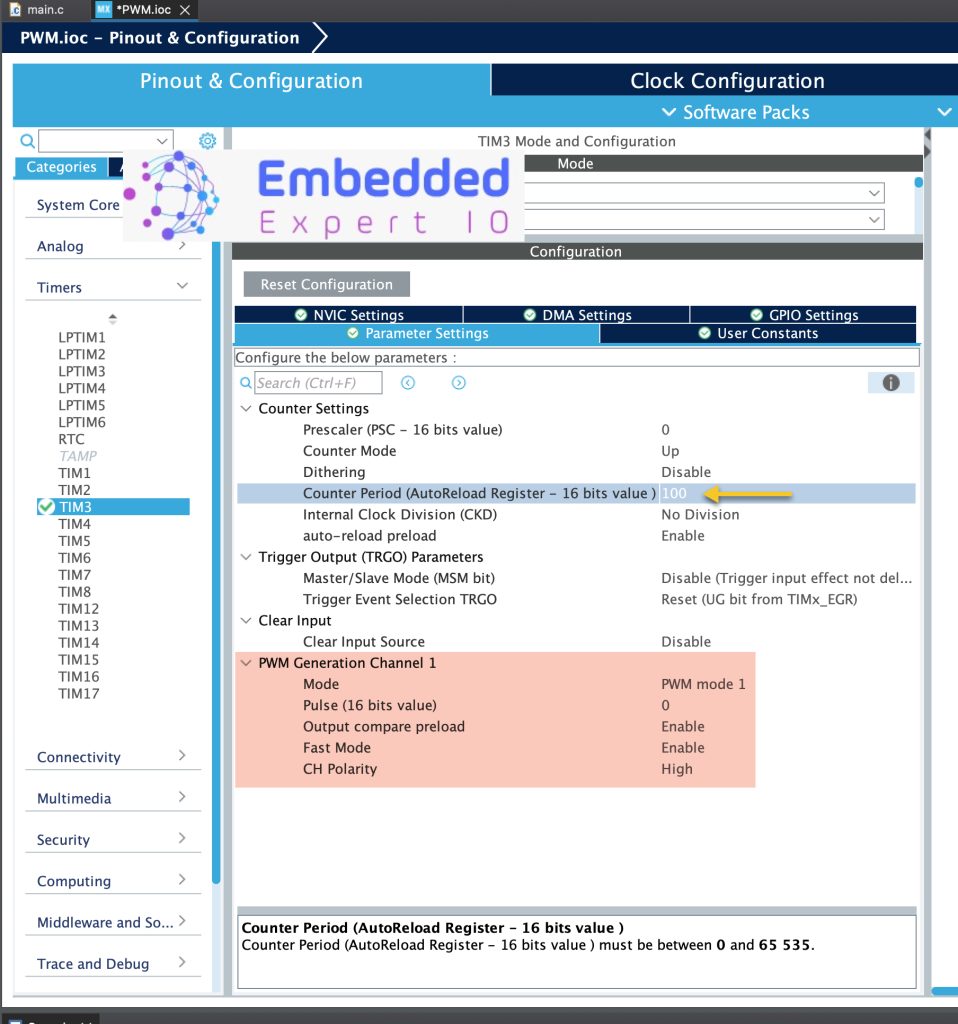
Thats all for the configuration, save the project and this will generate the project.
3. Firmware Development:
Once the project has been generated, main.c file shall be opened.
In main.c file, in user code begin 2 in the main function, we shall start the timer in PWM mode in for channel 1 as following:
HAL_TIM_PWM_Start(&htim3, TIM_CHANNEL_1);
The function shall take the following parameters:
- Pointer to the timer handler which is htim3 in this case.
- Timer channel which is timer channel 1 in this case.
In user code begin 3 in while 1 loop of the main code:
We shall change the duty cycle for fading effect as following:
for (int i=0;i<100;i++) { __HAL_TIM_SET_COMPARE(&htim3,TIM_CHANNEL_1,i); HAL_Delay(10); } HAL_Delay(10); for (int i=100;i>0;i--) { __HAL_TIM_SET_COMPARE(&htim3,TIM_CHANNEL_1,i); HAL_Delay(10); } HAL_Delay(10);
The macro:
__HAL_TIM_SET_COMPARE
Takes the following parameters:
- Instant to the timer which is tim3.
- Channel of the timer which is channel 1.
- The new value which is i in this case.
That all for the guide.
Save the project, build it and run it on your STM32H563Zi board.

4. Results:
You should get the following once the project has been uploaded to the board:
Happy coding 😉
Add Comment