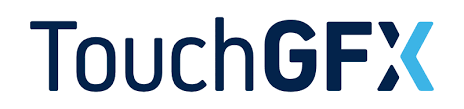
In this fifth and final guide on TouchGFX, we shall use intertask communication to send the data to UI.
This method is recommended to send data to UI since it will run in constant time interval.
In this guide, we shall cover the following:
- Firmware development.
- Results.
1. Firmware Development:
We shall start from the previous guide here.
From project explorer, open the ioc file as following:
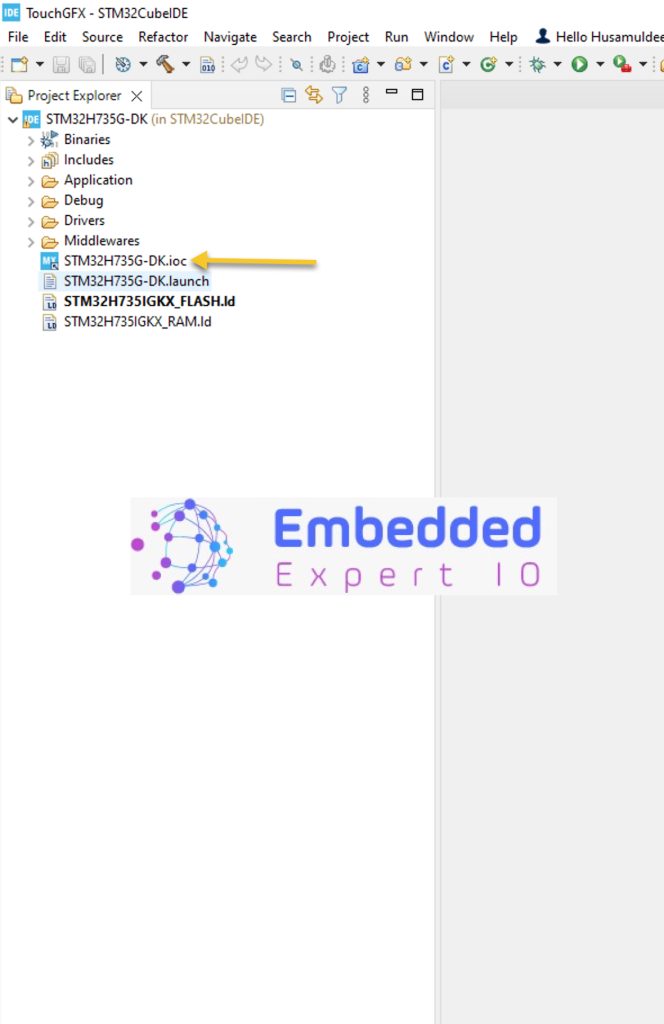
Then from middleware and software pack, select freeRTOS then Tasks and Queues tab as following:
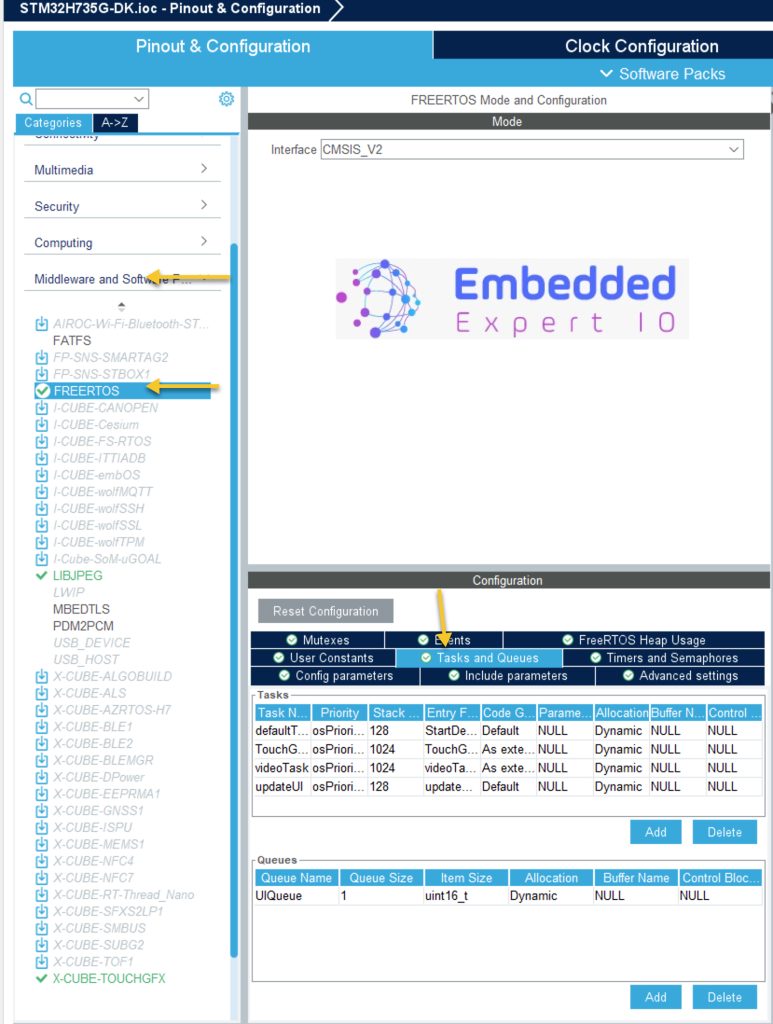
From Tasks and Queues, add the following task with the following parameters:
Task Name: updateUI
Priority: Normal (recommended).
Entry Function: updateUITask.
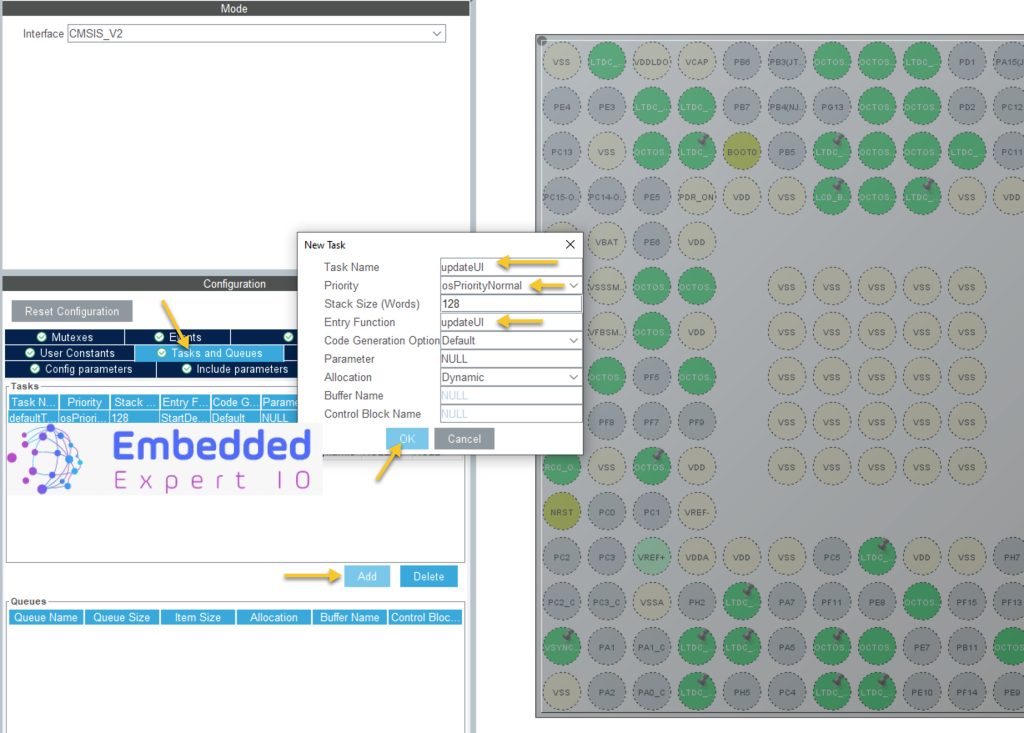
Then add the following queue:
Queue Name: UIQueue.
Queue Size: 1 (Enough for this example)
Item size: uint16_t.
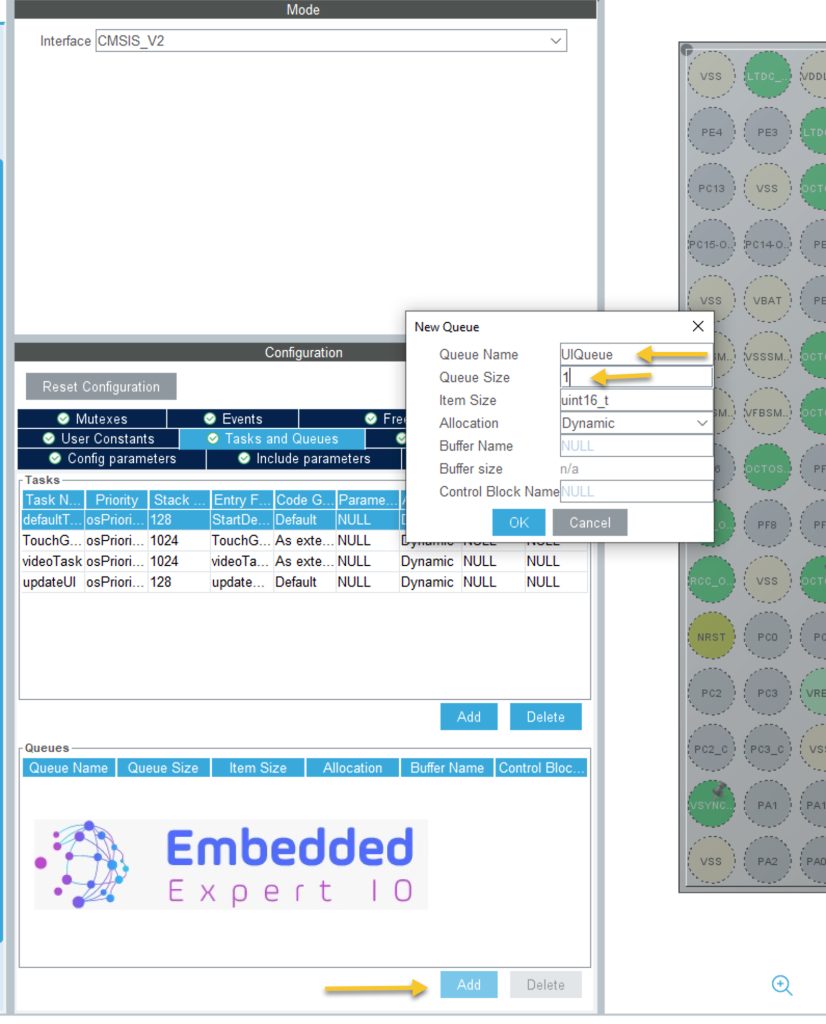
Save the project and this will generate the project.
In main.c file:
In user include header file, include stdbool header file as following:
#include "stdbool.h"
In user code begin PV, declare the following variables:
unsigned int value=0; bool dir=false;
Value is the counter value.
dir is direction, increment or decrement.
In updateUI task which is in main.c file:
void updateUITask(void *argument) { /* USER CODE BEGIN updateUITask */ /* Infinite loop */ for(;;) { if(dir==false)//Increase { value++; if(value==1000) { value=1000; dir=true; //change direction. } } if(dir==true)//Decrease { value--; if(value==0) { value=0; dir=false; //change direction. } } osMessageQueuePut(UIQueueHandle, &value, 0, 0); osDelay(10); } /* USER CODE END updateUITask */ }
In the infinite loop:
First check the direction, if false increment the value, once it is reaches 100, change the direction from increment to decrement.
If the direction is decrement, decrement the value, once the value is 0, change direction.
Then send the data to the queue as following:
osMessageQueuePut(UIQueueHandle, &value, 0, 0);
The function takes the following:
- Queue ID which is UIQueueHandle in this case.
- Address of the variable to be stored.
- Priority, set to 0.
- Timeout, set to 0.
Finally, delay by 10ms. You must use osDelay rather than HAL_Delay to prevent wasting CPU cycles.
Save main.c.
Open Model.cpp from Application, user, GUI as following:
Remove the old variables and the content of Model::tick function

In the source file, include cmsisosv2 header file as following:
#include "cmsis_os2.h"
Declare the queue as external as following:
extern osMessageQueueId_t UIQueueHandle;
Declare the following variable to store the value to be send to UI as following:
uint16_t value_ui;
In Model::tick function:
if(osMessageQueueGetCount(UIQueueHandle)>0) { osMessageQueueGet(UIQueueHandle, &value_ui, 0, 0); modelListener->UpdateGauge(value_ui); }
Check if there is new data in the queue, if there is new value, get it the new value and push it to the UI.
Thats all for the firmware development.
Save the project, build it and run it on your STM32H735G-DK board.

2. Results:
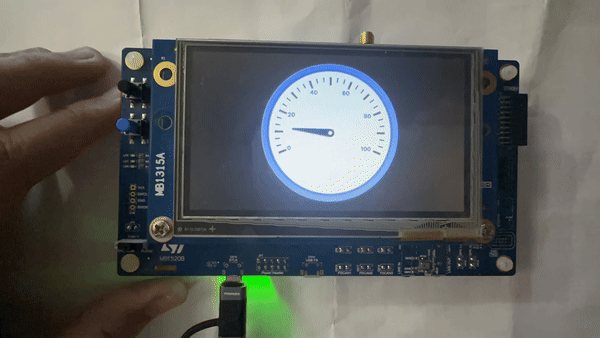
Happy coding 😉
Add Comment