
In the previous guide (here), we took a look at how to configure the pin as output. In this guide, we shall configure pins as input and control the state of the 3 on board LEDs.
In this guide, we shall cover the following:
- Input mode.
- Driver development.
- Results.
1. Input Modes:
GPIO input modes include
- high impedance
- pull-up
- pull-down
Floating, High Impedance, Tri-Stated
Floating, high impedance, and tri-stated are three terms that mean the same thing: the pin is just flopping in the breeze. Its state is indeterminate unless it is driven high or low externally. You only want to configure a pin as floating if you know it will be driven externally. Otherwise, configure the input using pulling resistors.
Pull Up/Down
If an input is configured with an internal pull-up, it will be high unless it is externally driven low. Pull-down inputs do the opposite ( they’re low unless driven high).
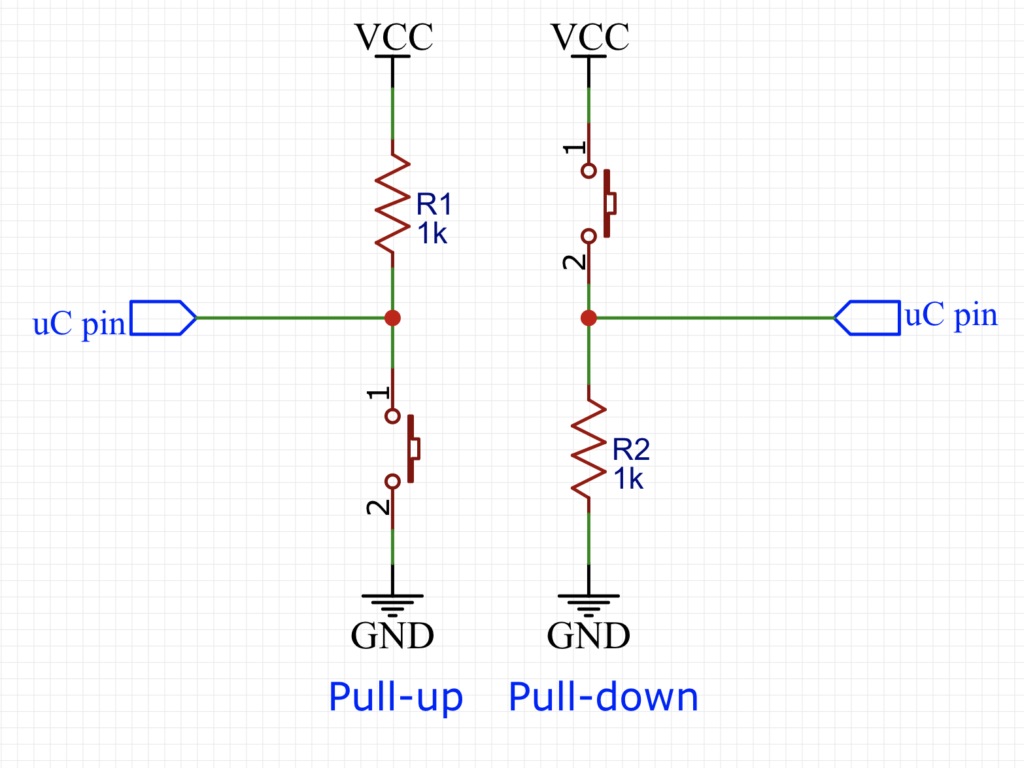
2. Driver Development:
Start off by creating new project and name it GPIO_Input.
After the creating the project.
Configure the LEDs pins and clocks as shown here.
From the STM32WB55 schematic, we can find that the Nucleo has 3 user pish-buttons as following:

SW1 is connected to PC4, SW2 to PD0 and SW3 to PD1.
Configure the pins as input as shown here:
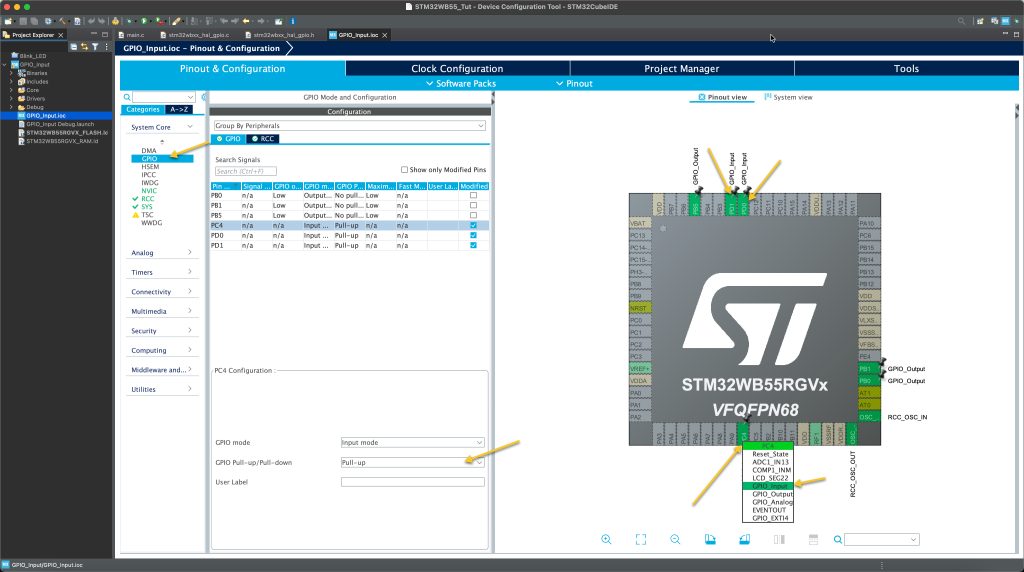
From System Core, select GPIO and enable the pullup for each input pins.
This is necessary since the buttons don’t have external pullup resistors.
Now, save the project and this shall generate the required code and open main.c.
In user begin 3 within the while 1 loop:
Add the following lines:
if(HAL_GPIO_ReadPin(GPIOC, GPIO_PIN_4)==GPIO_PIN_RESET) { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_5, GPIO_PIN_SET); } else { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_5, GPIO_PIN_RESET); } if(HAL_GPIO_ReadPin(GPIOD, GPIO_PIN_0)==GPIO_PIN_RESET) { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_0, GPIO_PIN_SET); } else { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_0, GPIO_PIN_RESET); } if(HAL_GPIO_ReadPin(GPIOD, GPIO_PIN_1)==GPIO_PIN_RESET) { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_1, GPIO_PIN_SET); } else { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_1, GPIO_PIN_RESET); }
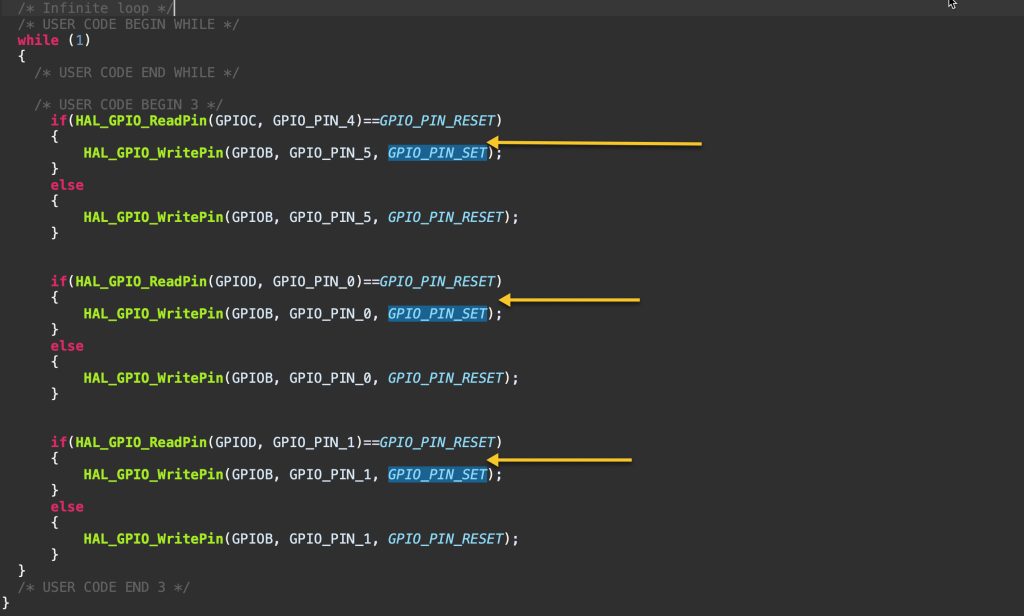
Save the project and run it on your Nucleo.
3. Results:
Happy coding 🙂
Add Comment