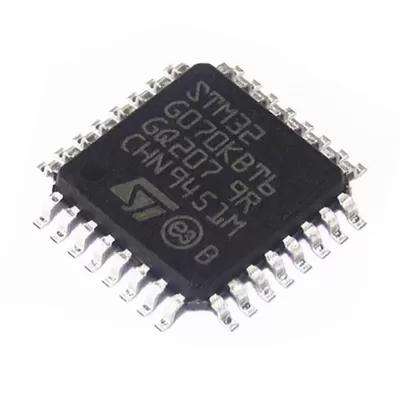
In the previous guide (here), we took a look at the UART and we were able to configure the STM32G070 to transmit data. Now, we shall receive data using UART in interrupt mode and echo back the received data.
In this guide, we shall cover the following:
- Enable USART2 interrupt.
- Code developing.
- Results.
1. Enable USART2 Interrupt:
By continue from the previous guide, open UART.ioc (depending on the name of the project name), Connectivity, then USART2, NVIC Settings and enable the USART2 Global interrupt as shown in the figure below
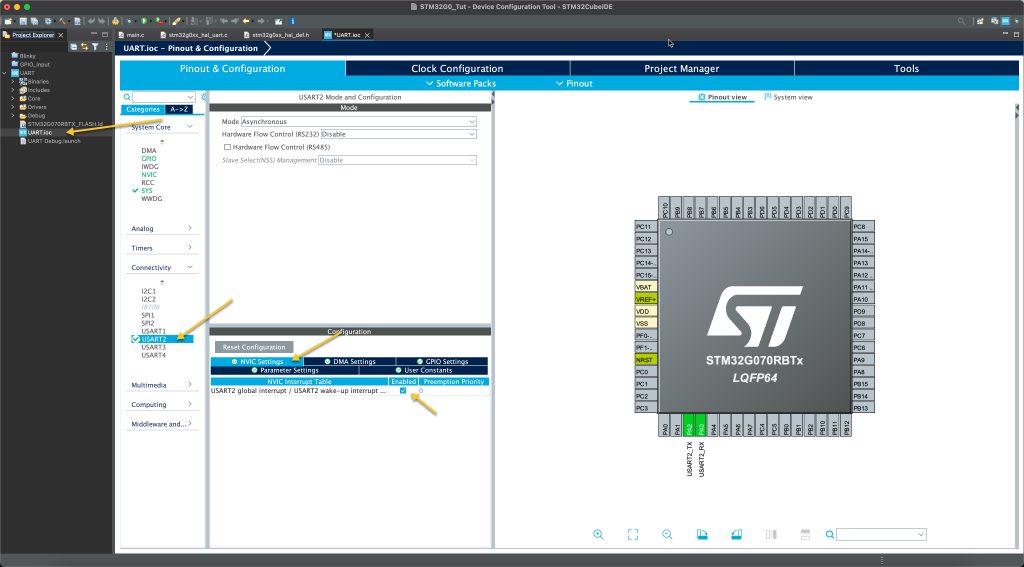
Save the project to generate the code.
Now, the USART2 is configured with interrupt capability.
2. Code Developing:
Since we are dealing with interrupt, HAL offers some interrupt drivers for UART interrupt.
From project tree, open drivers, STM32G0xx_HAL_DRIVER, then SRC then stm32g0xx_hal_uart.c
At line 2665, you will find this function:
__weak void HAL_UART_RxCpltCallback(UART_HandleTypeDef *huart) { /* Prevent unused argument(s) compilation warning */ UNUSED(huart); /* NOTE : This function should not be modified, when the callback is needed, the HAL_UART_RxCpltCallback can be implemented in the user file. */ }
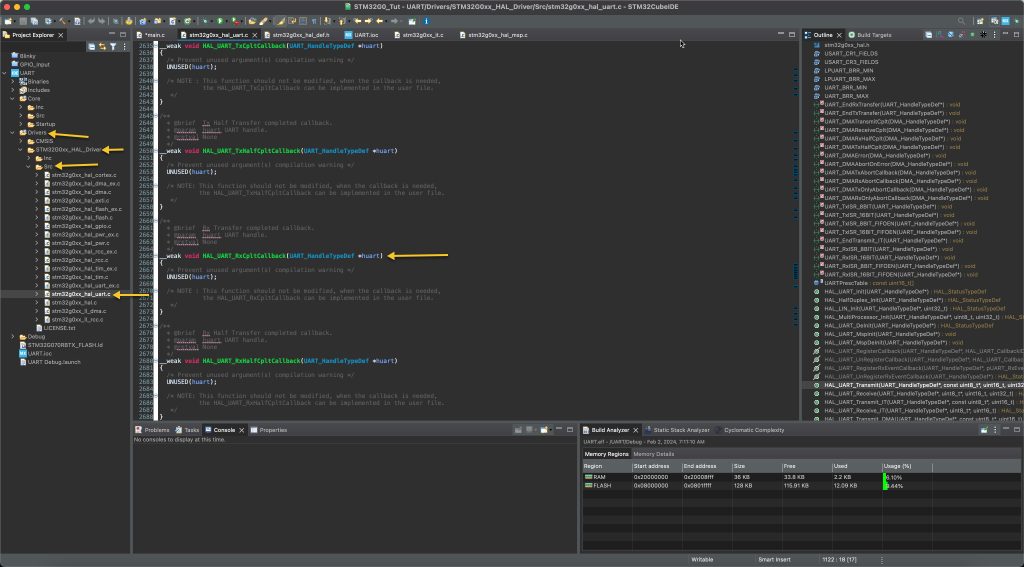
This function is defined as weak which can be overridden by the user. The purpose of this function is when the UART received the characters, this function shall be called.
Now, in main.c, the section between:
/* USER CODE BEGIN 0 */
and
/* USER CODE END 0 */
Declare the following variable:
First:
Buffer to hold the received characters:
uint8_t RxData[5];
In this example, we shall receive 5 characters.
Buffer to hold the data to be transmitted:
uint8_t TxData[40];
Adapt the size according to your application.
Declare a volatile variable which acts as flag:
volatile uint8_t finished;
Now, redeclare the weak as following:
void HAL_UART_RxCpltCallback(UART_HandleTypeDef *huart) { finished=1; HAL_UART_Receive_IT(&huart2, RxData, 5); }
In the function, we shall set the flag to 1 and start the interrupt again.
In User code begin 2 in the main function:
Start the UART to receive in interrupt mode:
HAL_UART_Receive_IT(&huart2, RxData, 5);
In user code begin 3:
if(finished==1) { len=sprintf((char*)TxData,"Received Data are %c %c %c %c %c \r\n", RxData[0],RxData[1],RxData[2],RxData[3],RxData[4]); HAL_UART_Transmit(&huart2, TxData, len, 100); finished=0; }
Check if the flag is set, if yes, print the received characters and set the flag back to 0.
Thats all for the code.
3. Results:
Using any serial port program, set the baud rate of 115200, send any 5 characters without \r\n and see the echoed characters.
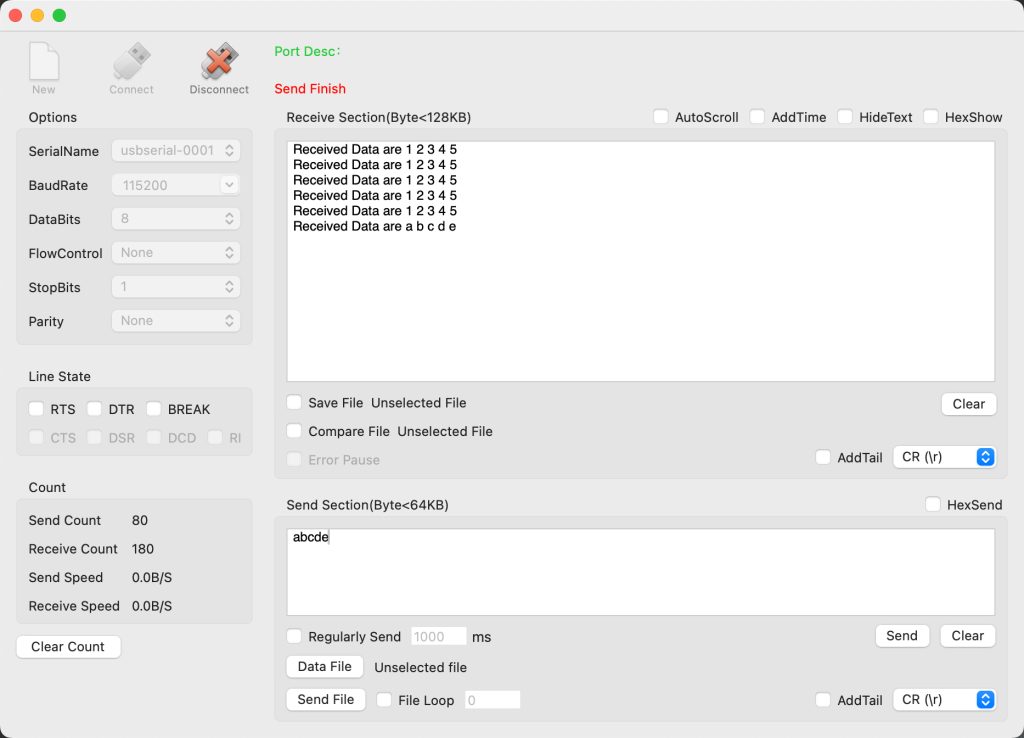
Happy coding 🙂
Add Comment