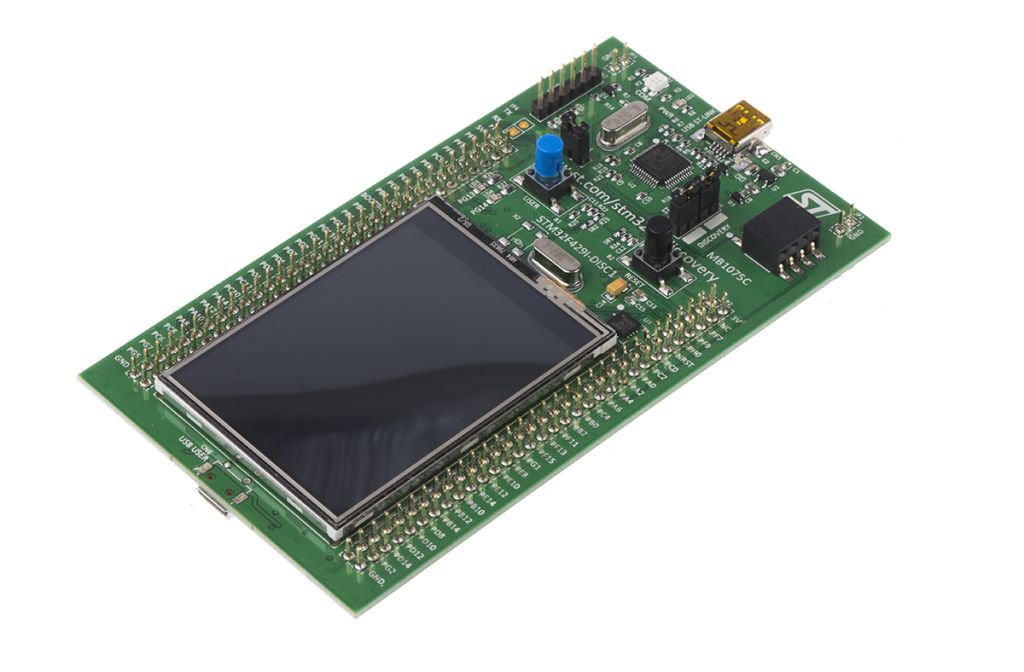
In the previous guide (here), we took a look at STM32F429 Discovery and it’s features and the environment has been setup. In this guide, we shall cover the following:
- Delay initializing.
- LCD initializing.
- Support functions.
- Code
- Results.
1. Delay initializing:
We start off by creating new header file with name of delay.h.
Within the header file, include the header guard:
#ifndef DELAY_H_ #define DELAY_H_ #endif /* DELAY_H_ */
Include the following header file:
#include "stdint.h"
Declare the following functions:
void delay_init(uint32_t freq); uint64_t millis(); void delay(uint32_t time);
Hence, the entire header file as following:
#ifndef DELAY_H_ #define DELAY_H_ #include "stdint.h" void delay_init(uint32_t freq); uint64_t millis(); void delay(uint32_t time); #endif /* DELAY_H_ */
Now. Create new source code with name of delay.c.
Within the source file:
#include "delay.h" #include "stm32f4xx.h" #define CTRL_ENABLE (1U<<0) #define CTRL_CLKSRC (1U<<2) #define CTRL_COUNTFLAG (1U<<16) #define CTRL_TICKINT (1U<<1) volatile uint64_t mil; void delay_init(uint32_t freq) { SysTick->LOAD = (freq/1000) - 1; /*Clear systick current value register */ SysTick->VAL = 0; /*Enable systick and select internal clk src*/ SysTick->CTRL = CTRL_ENABLE | CTRL_CLKSRC ; /*Enable systick interrupt*/ SysTick->CTRL |= CTRL_TICKINT; } uint64_t millis() { __disable_irq(); uint64_t ml=mil; __enable_irq(); return ml; } void delay(uint32_t time) { uint64_t start=millis(); while((millis() - start) < time); } void SysTick_Handler(void) { mil++; }
How to implement the systick interrupt, check this guide.
Thats all for the delay part. Next, the LCD initialization.
2. LCD Initializing:
Create new header file with name of ILI9341.h.
Include the header guard as following:
#ifndef ILI9341_H_ #define ILI9341_H_ #endif
Within the header file, include the following header files:
//List of includes #include <stdbool.h> #include "delay.h" #include <stdint.h> #include <math.h>
Now, we shall declare list of symbolics, we start off by the dimensions of the LCD as following:
//LCD dimensions defines #define ILI9341_WIDTH 240 #define ILI9341_HEIGHT 320
Total pixels:
#define ILI9341_PIXEL_COUNT ILI9341_WIDTH * ILI9341_HEIGHT
List of LCD commands:
//ILI9341 LCD commands #define ILI9341_RESET 0x01 #define ILI9341_SLEEP_OUT 0x11 #define ILI9341_GAMMA 0x26 #define ILI9341_DISPLAY_OFF 0x28 #define ILI9341_DISPLAY_ON 0x29 #define ILI9341_COLUMN_ADDR 0x2A #define ILI9341_PAGE_ADDR 0x2B #define ILI9341_GRAM 0x2C #define ILI9341_TEARING_OFF 0x34 #define ILI9341_TEARING_ON 0x35 #define ILI9341_DISPLAY_INVERSION 0xb4 #define ILI9341_MAC 0x36 #define ILI9341_PIXEL_FORMAT 0x3A #define ILI9341_WDB 0x51 #define ILI9341_WCD 0x53 #define ILI9341_RGB_INTERFACE 0xB0 #define ILI9341_FRC 0xB1 #define ILI9341_BPC 0xB5 #define ILI9341_DFC 0xB6 #define ILI9341_Entry_Mode_Set 0xB7 #define ILI9341_POWER1 0xC0 #define ILI9341_POWER2 0xC1 #define ILI9341_VCOM1 0xC5 #define ILI9341_VCOM2 0xC7 #define ILI9341_POWERA 0xCB #define ILI9341_POWERB 0xCF #define ILI9341_PGAMMA 0xE0 #define ILI9341_NGAMMA 0xE1 #define ILI9341_DTCA 0xE8 #define ILI9341_DTCB 0xEA #define ILI9341_POWER_SEQ 0xED #define ILI9341_3GAMMA_EN 0xF2 #define ILI9341_INTERFACE 0xF6 #define ILI9341_PRC 0xF7 #define ILI9341_VERTICAL_SCROLL 0x33 #define ILI9341_MEMCONTROL 0x36 #define ILI9341_MADCTL_MY 0x80 #define ILI9341_MADCTL_MX 0x40 #define ILI9341_MADCTL_MV 0x20 #define ILI9341_MADCTL_ML 0x10 #define ILI9341_MADCTL_RGB 0x00 #define ILI9341_MADCTL_BGR 0x08 #define ILI9341_MADCTL_MH 0x04
List of colors:
//List of colors #define COLOR_BLACK 0x0000 #define COLOR_NAVY 0x000F #define COLOR_DGREEN 0x03E0 #define COLOR_DCYAN 0x03EF #define COLOR_MAROON 0x7800 #define COLOR_PURPLE 0x780F #define COLOR_OLIVE 0x7BE0 #define COLOR_LGRAY 0xC618 #define COLOR_DGRAY 0x7BEF #define COLOR_BLUE 0x001F #define COLOR_BLUE2 0x051D #define COLOR_GREEN 0x07E0 #define COLOR_GREEN2 0xB723 #define COLOR_GREEN3 0x8000 #define COLOR_CYAN 0x07FF #define COLOR_RED 0xF800 #define COLOR_MAGENTA 0xF81F #define COLOR_YELLOW 0xFFE0 #define COLOR_WHITE 0xFFFF #define COLOR_ORANGE 0xFD20 #define COLOR_GREENYELLOW 0xAFE5 #define COLOR_BROWN 0XBC40 #define COLOR_BRRED 0XFC07
Required macros:
//Functions defines Macros #define swap(a, b) { int16_t t = a; a = b; b = t; } #define min(a,b) (((a)<(b))?(a):(b))
Font declarations:
static //Text simple font array (You can your own font) const unsigned char Font[] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x3E, 0x5B, 0x4F, 0x5B, 0x3E, 0x3E, 0x6B, 0x4F, 0x6B, 0x3E, 0x1C, 0x3E, 0x7C, 0x3E, 0x1C, 0x18, 0x3C, 0x7E, 0x3C, 0x18, 0x1C, 0x57, 0x7D, 0x57, 0x1C, 0x1C, 0x5E, 0x7F, 0x5E, 0x1C, 0x00, 0x18, 0x3C, 0x18, 0x00, 0xFF, 0xE7, 0xC3, 0xE7, 0xFF, 0x00, 0x18, 0x24, 0x18, 0x00, 0xFF, 0xE7, 0xDB, 0xE7, 0xFF, 0x30, 0x48, 0x3A, 0x06, 0x0E, 0x26, 0x29, 0x79, 0x29, 0x26, 0x40, 0x7F, 0x05, 0x05, 0x07, 0x40, 0x7F, 0x05, 0x25, 0x3F, 0x5A, 0x3C, 0xE7, 0x3C, 0x5A, 0x7F, 0x3E, 0x1C, 0x1C, 0x08, 0x08, 0x1C, 0x1C, 0x3E, 0x7F, 0x14, 0x22, 0x7F, 0x22, 0x14, 0x5F, 0x5F, 0x00, 0x5F, 0x5F, 0x06, 0x09, 0x7F, 0x01, 0x7F, 0x00, 0x66, 0x89, 0x95, 0x6A, 0x60, 0x60, 0x60, 0x60, 0x60, 0x94, 0xA2, 0xFF, 0xA2, 0x94, 0x08, 0x04, 0x7E, 0x04, 0x08, 0x10, 0x20, 0x7E, 0x20, 0x10, 0x08, 0x08, 0x2A, 0x1C, 0x08, 0x08, 0x1C, 0x2A, 0x08, 0x08, 0x1E, 0x10, 0x10, 0x10, 0x10, 0x0C, 0x1E, 0x0C, 0x1E, 0x0C, 0x30, 0x38, 0x3E, 0x38, 0x30, 0x06, 0x0E, 0x3E, 0x0E, 0x06, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x5F, 0x00, 0x00, 0x00, 0x07, 0x00, 0x07, 0x00, 0x14, 0x7F, 0x14, 0x7F, 0x14, 0x24, 0x2A, 0x7F, 0x2A, 0x12, 0x23, 0x13, 0x08, 0x64, 0x62, 0x36, 0x49, 0x56, 0x20, 0x50, 0x00, 0x08, 0x07, 0x03, 0x00, 0x00, 0x1C, 0x22, 0x41, 0x00, 0x00, 0x41, 0x22, 0x1C, 0x00, 0x2A, 0x1C, 0x7F, 0x1C, 0x2A, 0x08, 0x08, 0x3E, 0x08, 0x08, 0x00, 0x80, 0x70, 0x30, 0x00, 0x08, 0x08, 0x08, 0x08, 0x08, 0x00, 0x00, 0x60, 0x60, 0x00, 0x20, 0x10, 0x08, 0x04, 0x02, 0x3E, 0x51, 0x49, 0x45, 0x3E, 0x00, 0x42, 0x7F, 0x40, 0x00, 0x72, 0x49, 0x49, 0x49, 0x46, 0x21, 0x41, 0x49, 0x4D, 0x33, 0x18, 0x14, 0x12, 0x7F, 0x10, 0x27, 0x45, 0x45, 0x45, 0x39, 0x3C, 0x4A, 0x49, 0x49, 0x31, 0x41, 0x21, 0x11, 0x09, 0x07, 0x36, 0x49, 0x49, 0x49, 0x36, 0x46, 0x49, 0x49, 0x29, 0x1E, 0x00, 0x00, 0x14, 0x00, 0x00, 0x00, 0x40, 0x34, 0x00, 0x00, 0x00, 0x08, 0x14, 0x22, 0x41, 0x14, 0x14, 0x14, 0x14, 0x14, 0x00, 0x41, 0x22, 0x14, 0x08, 0x02, 0x01, 0x59, 0x09, 0x06, 0x3E, 0x41, 0x5D, 0x59, 0x4E, 0x7C, 0x12, 0x11, 0x12, 0x7C, 0x7F, 0x49, 0x49, 0x49, 0x36, 0x3E, 0x41, 0x41, 0x41, 0x22, 0x7F, 0x41, 0x41, 0x41, 0x3E, 0x7F, 0x49, 0x49, 0x49, 0x41, 0x7F, 0x09, 0x09, 0x09, 0x01, 0x3E, 0x41, 0x41, 0x51, 0x73, 0x7F, 0x08, 0x08, 0x08, 0x7F, 0x00, 0x41, 0x7F, 0x41, 0x00, 0x20, 0x40, 0x41, 0x3F, 0x01, 0x7F, 0x08, 0x14, 0x22, 0x41, 0x7F, 0x40, 0x40, 0x40, 0x40, 0x7F, 0x02, 0x1C, 0x02, 0x7F, 0x7F, 0x04, 0x08, 0x10, 0x7F, 0x3E, 0x41, 0x41, 0x41, 0x3E, 0x7F, 0x09, 0x09, 0x09, 0x06, 0x3E, 0x41, 0x51, 0x21, 0x5E, 0x7F, 0x09, 0x19, 0x29, 0x46, 0x26, 0x49, 0x49, 0x49, 0x32, 0x03, 0x01, 0x7F, 0x01, 0x03, 0x3F, 0x40, 0x40, 0x40, 0x3F, 0x1F, 0x20, 0x40, 0x20, 0x1F, 0x3F, 0x40, 0x38, 0x40, 0x3F, 0x63, 0x14, 0x08, 0x14, 0x63, 0x03, 0x04, 0x78, 0x04, 0x03, 0x61, 0x59, 0x49, 0x4D, 0x43, 0x00, 0x7F, 0x41, 0x41, 0x41, 0x02, 0x04, 0x08, 0x10, 0x20, 0x00, 0x41, 0x41, 0x41, 0x7F, 0x04, 0x02, 0x01, 0x02, 0x04, 0x40, 0x40, 0x40, 0x40, 0x40, 0x00, 0x03, 0x07, 0x08, 0x00, 0x20, 0x54, 0x54, 0x78, 0x40, 0x7F, 0x28, 0x44, 0x44, 0x38, 0x38, 0x44, 0x44, 0x44, 0x28, 0x38, 0x44, 0x44, 0x28, 0x7F, 0x38, 0x54, 0x54, 0x54, 0x18, 0x00, 0x08, 0x7E, 0x09, 0x02, 0x18, 0xA4, 0xA4, 0x9C, 0x78, 0x7F, 0x08, 0x04, 0x04, 0x78, 0x00, 0x44, 0x7D, 0x40, 0x00, 0x20, 0x40, 0x40, 0x3D, 0x00, 0x7F, 0x10, 0x28, 0x44, 0x00, 0x00, 0x41, 0x7F, 0x40, 0x00, 0x7C, 0x04, 0x78, 0x04, 0x78, 0x7C, 0x08, 0x04, 0x04, 0x78, 0x38, 0x44, 0x44, 0x44, 0x38, 0xFC, 0x18, 0x24, 0x24, 0x18, 0x18, 0x24, 0x24, 0x18, 0xFC, 0x7C, 0x08, 0x04, 0x04, 0x08, 0x48, 0x54, 0x54, 0x54, 0x24, 0x04, 0x04, 0x3F, 0x44, 0x24, 0x3C, 0x40, 0x40, 0x20, 0x7C, 0x1C, 0x20, 0x40, 0x20, 0x1C, 0x3C, 0x40, 0x30, 0x40, 0x3C, 0x44, 0x28, 0x10, 0x28, 0x44, 0x4C, 0x90, 0x90, 0x90, 0x7C, 0x44, 0x64, 0x54, 0x4C, 0x44, 0x00, 0x08, 0x36, 0x41, 0x00, 0x00, 0x00, 0x77, 0x00, 0x00, 0x00, 0x41, 0x36, 0x08, 0x00, 0x02, 0x01, 0x02, 0x04, 0x02, 0x3C, 0x26, 0x23, 0x26, 0x3C, 0x1E, 0xA1, 0xA1, 0x61, 0x12, 0x3A, 0x40, 0x40, 0x20, 0x7A, 0x38, 0x54, 0x54, 0x55, 0x59, 0x21, 0x55, 0x55, 0x79, 0x41, 0x22, 0x54, 0x54, 0x78, 0x42, 0x21, 0x55, 0x54, 0x78, 0x40, 0x20, 0x54, 0x55, 0x79, 0x40, 0x0C, 0x1E, 0x52, 0x72, 0x12, 0x39, 0x55, 0x55, 0x55, 0x59, 0x39, 0x54, 0x54, 0x54, 0x59, 0x39, 0x55, 0x54, 0x54, 0x58, 0x00, 0x00, 0x45, 0x7C, 0x41, 0x00, 0x02, 0x45, 0x7D, 0x42, 0x00, 0x01, 0x45, 0x7C, 0x40, 0x7D, 0x12, 0x11, 0x12, 0x7D, 0xF0, 0x28, 0x25, 0x28, 0xF0, 0x7C, 0x54, 0x55, 0x45, 0x00, 0x20, 0x54, 0x54, 0x7C, 0x54, 0x7C, 0x0A, 0x09, 0x7F, 0x49, 0x32, 0x49, 0x49, 0x49, 0x32, 0x3A, 0x44, 0x44, 0x44, 0x3A, 0x32, 0x4A, 0x48, 0x48, 0x30, 0x3A, 0x41, 0x41, 0x21, 0x7A, 0x3A, 0x42, 0x40, 0x20, 0x78, 0x00, 0x9D, 0xA0, 0xA0, 0x7D, 0x3D, 0x42, 0x42, 0x42, 0x3D, 0x3D, 0x40, 0x40, 0x40, 0x3D, 0x3C, 0x24, 0xFF, 0x24, 0x24, 0x48, 0x7E, 0x49, 0x43, 0x66, 0x2B, 0x2F, 0xFC, 0x2F, 0x2B, 0xFF, 0x09, 0x29, 0xF6, 0x20, 0xC0, 0x88, 0x7E, 0x09, 0x03, 0x20, 0x54, 0x54, 0x79, 0x41, 0x00, 0x00, 0x44, 0x7D, 0x41, 0x30, 0x48, 0x48, 0x4A, 0x32, 0x38, 0x40, 0x40, 0x22, 0x7A, 0x00, 0x7A, 0x0A, 0x0A, 0x72, 0x7D, 0x0D, 0x19, 0x31, 0x7D, 0x26, 0x29, 0x29, 0x2F, 0x28, 0x26, 0x29, 0x29, 0x29, 0x26, 0x30, 0x48, 0x4D, 0x40, 0x20, 0x38, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x38, 0x2F, 0x10, 0xC8, 0xAC, 0xBA, 0x2F, 0x10, 0x28, 0x34, 0xFA, 0x00, 0x00, 0x7B, 0x00, 0x00, 0x08, 0x14, 0x2A, 0x14, 0x22, 0x22, 0x14, 0x2A, 0x14, 0x08, 0x55, 0x00, 0x55, 0x00, 0x55, 0xAA, 0x55, 0xAA, 0x55, 0xAA, 0xFF, 0x55, 0xFF, 0x55, 0xFF, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x10, 0x10, 0x10, 0xFF, 0x00, 0x14, 0x14, 0x14, 0xFF, 0x00, 0x10, 0x10, 0xFF, 0x00, 0xFF, 0x10, 0x10, 0xF0, 0x10, 0xF0, 0x14, 0x14, 0x14, 0xFC, 0x00, 0x14, 0x14, 0xF7, 0x00, 0xFF, 0x00, 0x00, 0xFF, 0x00, 0xFF, 0x14, 0x14, 0xF4, 0x04, 0xFC, 0x14, 0x14, 0x17, 0x10, 0x1F, 0x10, 0x10, 0x1F, 0x10, 0x1F, 0x14, 0x14, 0x14, 0x1F, 0x00, 0x10, 0x10, 0x10, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x10, 0x10, 0x10, 0x10, 0x1F, 0x10, 0x10, 0x10, 0x10, 0xF0, 0x10, 0x00, 0x00, 0x00, 0xFF, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0xFF, 0x10, 0x00, 0x00, 0x00, 0xFF, 0x14, 0x00, 0x00, 0xFF, 0x00, 0xFF, 0x00, 0x00, 0x1F, 0x10, 0x17, 0x00, 0x00, 0xFC, 0x04, 0xF4, 0x14, 0x14, 0x17, 0x10, 0x17, 0x14, 0x14, 0xF4, 0x04, 0xF4, 0x00, 0x00, 0xFF, 0x00, 0xF7, 0x14, 0x14, 0x14, 0x14, 0x14, 0x14, 0x14, 0xF7, 0x00, 0xF7, 0x14, 0x14, 0x14, 0x17, 0x14, 0x10, 0x10, 0x1F, 0x10, 0x1F, 0x14, 0x14, 0x14, 0xF4, 0x14, 0x10, 0x10, 0xF0, 0x10, 0xF0, 0x00, 0x00, 0x1F, 0x10, 0x1F, 0x00, 0x00, 0x00, 0x1F, 0x14, 0x00, 0x00, 0x00, 0xFC, 0x14, 0x00, 0x00, 0xF0, 0x10, 0xF0, 0x10, 0x10, 0xFF, 0x10, 0xFF, 0x14, 0x14, 0x14, 0xFF, 0x14, 0x10, 0x10, 0x10, 0x1F, 0x00, 0x00, 0x00, 0x00, 0xF0, 0x10, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x38, 0x44, 0x44, 0x38, 0x44, 0xFC, 0x4A, 0x4A, 0x4A, 0x34, 0x7E, 0x02, 0x02, 0x06, 0x06, 0x02, 0x7E, 0x02, 0x7E, 0x02, 0x63, 0x55, 0x49, 0x41, 0x63, 0x38, 0x44, 0x44, 0x3C, 0x04, 0x40, 0x7E, 0x20, 0x1E, 0x20, 0x06, 0x02, 0x7E, 0x02, 0x02, 0x99, 0xA5, 0xE7, 0xA5, 0x99, 0x1C, 0x2A, 0x49, 0x2A, 0x1C, 0x4C, 0x72, 0x01, 0x72, 0x4C, 0x30, 0x4A, 0x4D, 0x4D, 0x30, 0x30, 0x48, 0x78, 0x48, 0x30, 0xBC, 0x62, 0x5A, 0x46, 0x3D, 0x3E, 0x49, 0x49, 0x49, 0x00, 0x7E, 0x01, 0x01, 0x01, 0x7E, 0x2A, 0x2A, 0x2A, 0x2A, 0x2A, 0x44, 0x44, 0x5F, 0x44, 0x44, 0x40, 0x51, 0x4A, 0x44, 0x40, 0x40, 0x44, 0x4A, 0x51, 0x40, 0x00, 0x00, 0xFF, 0x01, 0x03, 0xE0, 0x80, 0xFF, 0x00, 0x00, 0x08, 0x08, 0x6B, 0x6B, 0x08, 0x36, 0x12, 0x36, 0x24, 0x36, 0x06, 0x0F, 0x09, 0x0F, 0x06, 0x00, 0x00, 0x18, 0x18, 0x00, 0x00, 0x00, 0x10, 0x10, 0x00, 0x30, 0x40, 0xFF, 0x01, 0x01, 0x00, 0x1F, 0x01, 0x01, 0x1E, 0x00, 0x19, 0x1D, 0x17, 0x12, 0x00, 0x3C, 0x3C, 0x3C, 0x3C, 0x00, 0x00, 0x00, 0x00, 0x00 };
Functions declarations:
//***** Functions prototypes *****// //1. Write Command to LCD void ILI9341_SendCommand(uint8_t com); //2. Write data to LCD void ILI9341_SendData(uint8_t data); //2.2 Write multiple/DMA void ILI9341_SendData_Multi(uint16_t Colordata, uint32_t size); //3. Set cursor position void ILI9341_SetCursorPosition(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); //4. Initialise function void ILI9341_Init(); //5. Write data to a single pixel void ILI9341_DrawPixel(uint16_t x, uint16_t y, uint16_t color); //Draw single pixel to ILI9341 //6. Fill the entire screen with a background color void ILI9341_Fill(uint16_t color); //Fill entire ILI9341 with color //7. Rectangle drawing functions void ILI9341_Fill_Rect(unsigned int x0, unsigned int y0, unsigned int x1, unsigned int y1, uint16_t color); //8. Circle drawing functions void ILI9341_drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color); static void drawCircleHelper( int16_t x0, int16_t y0, int16_t r, uint8_t cornername, uint16_t color); static void fillCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, int16_t delta, uint16_t color); void ILI9341_fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color); //9. Line drawing functions void ILI9341_drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color); void ILI9341_drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color); void ILI9341_drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); //10. Triangle drawing void ILI9341_drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color); void ILI9341_fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color); //11. Text printing functions //void ILI9341_drawChar(int16_t x, int16_t y, unsigned char c, uint16_t color, uint16_t bg, uint8_t size); //void ILI9341_printText(char text[], int16_t x, int16_t y, uint16_t color, uint16_t bg, uint8_t size); //12. Image print (RGB 565, 2 bytes per pixel) void ILI9341_printImage(uint16_t x, uint16_t y, uint16_t w, uint16_t h, uint8_t *data, uint32_t size); //13. Set screen rotation void ILI9341_setRotation(uint8_t rotate); //14. Get screen rotation uint8_t ILI9341_getRotation(void); //draw a single character void ILI9341_DrawChar(int16_t x, int16_t y, char c, int16_t textColor, int16_t bgColor, uint8_t size); //sensd 16-bit color data uint16_t ILI9341_Color565(uint8_t r, uint8_t g, uint8_t b); //write a string to the lcd uint32_t ILI9341_DrawString(uint16_t x, uint16_t y, char *pt, int16_t textColor,int16_t BG,uint16_t size);
Hence, the entire header file as following:
#ifndef ILI9341_H_ #define ILI9341_H_ //List of includes #include <stdbool.h> #include "delay.h" #include <stdint.h> #include <math.h> //LCD dimensions defines #define ILI9341_WIDTH 240 #define ILI9341_HEIGHT 320 #define ILI9341_PIXEL_COUNT ILI9341_WIDTH * ILI9341_HEIGHT //ILI9341 LCD commands #define ILI9341_RESET 0x01 #define ILI9341_SLEEP_OUT 0x11 #define ILI9341_GAMMA 0x26 #define ILI9341_DISPLAY_OFF 0x28 #define ILI9341_DISPLAY_ON 0x29 #define ILI9341_COLUMN_ADDR 0x2A #define ILI9341_PAGE_ADDR 0x2B #define ILI9341_GRAM 0x2C #define ILI9341_TEARING_OFF 0x34 #define ILI9341_TEARING_ON 0x35 #define ILI9341_DISPLAY_INVERSION 0xb4 #define ILI9341_MAC 0x36 #define ILI9341_PIXEL_FORMAT 0x3A #define ILI9341_WDB 0x51 #define ILI9341_WCD 0x53 #define ILI9341_RGB_INTERFACE 0xB0 #define ILI9341_FRC 0xB1 #define ILI9341_BPC 0xB5 #define ILI9341_DFC 0xB6 #define ILI9341_Entry_Mode_Set 0xB7 #define ILI9341_POWER1 0xC0 #define ILI9341_POWER2 0xC1 #define ILI9341_VCOM1 0xC5 #define ILI9341_VCOM2 0xC7 #define ILI9341_POWERA 0xCB #define ILI9341_POWERB 0xCF #define ILI9341_PGAMMA 0xE0 #define ILI9341_NGAMMA 0xE1 #define ILI9341_DTCA 0xE8 #define ILI9341_DTCB 0xEA #define ILI9341_POWER_SEQ 0xED #define ILI9341_3GAMMA_EN 0xF2 #define ILI9341_INTERFACE 0xF6 #define ILI9341_PRC 0xF7 #define ILI9341_VERTICAL_SCROLL 0x33 #define ILI9341_MEMCONTROL 0x36 #define ILI9341_MADCTL_MY 0x80 #define ILI9341_MADCTL_MX 0x40 #define ILI9341_MADCTL_MV 0x20 #define ILI9341_MADCTL_ML 0x10 #define ILI9341_MADCTL_RGB 0x00 #define ILI9341_MADCTL_BGR 0x08 #define ILI9341_MADCTL_MH 0x04 //List of colors #define COLOR_BLACK 0x0000 #define COLOR_NAVY 0x000F #define COLOR_DGREEN 0x03E0 #define COLOR_DCYAN 0x03EF #define COLOR_MAROON 0x7800 #define COLOR_PURPLE 0x780F #define COLOR_OLIVE 0x7BE0 #define COLOR_LGRAY 0xC618 #define COLOR_DGRAY 0x7BEF #define COLOR_BLUE 0x001F #define COLOR_BLUE2 0x051D #define COLOR_GREEN 0x07E0 #define COLOR_GREEN2 0xB723 #define COLOR_GREEN3 0x8000 #define COLOR_CYAN 0x07FF #define COLOR_RED 0xF800 #define COLOR_MAGENTA 0xF81F #define COLOR_YELLOW 0xFFE0 #define COLOR_WHITE 0xFFFF #define COLOR_ORANGE 0xFD20 #define COLOR_GREENYELLOW 0xAFE5 #define COLOR_BROWN 0XBC40 #define COLOR_BRRED 0XFC07 //Functions defines Macros #define swap(a, b) { int16_t t = a; a = b; b = t; } #define min(a,b) (((a)<(b))?(a):(b)) //font static //Text simple font array (You can your own font) const unsigned char Font[] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x3E, 0x5B, 0x4F, 0x5B, 0x3E, 0x3E, 0x6B, 0x4F, 0x6B, 0x3E, 0x1C, 0x3E, 0x7C, 0x3E, 0x1C, 0x18, 0x3C, 0x7E, 0x3C, 0x18, 0x1C, 0x57, 0x7D, 0x57, 0x1C, 0x1C, 0x5E, 0x7F, 0x5E, 0x1C, 0x00, 0x18, 0x3C, 0x18, 0x00, 0xFF, 0xE7, 0xC3, 0xE7, 0xFF, 0x00, 0x18, 0x24, 0x18, 0x00, 0xFF, 0xE7, 0xDB, 0xE7, 0xFF, 0x30, 0x48, 0x3A, 0x06, 0x0E, 0x26, 0x29, 0x79, 0x29, 0x26, 0x40, 0x7F, 0x05, 0x05, 0x07, 0x40, 0x7F, 0x05, 0x25, 0x3F, 0x5A, 0x3C, 0xE7, 0x3C, 0x5A, 0x7F, 0x3E, 0x1C, 0x1C, 0x08, 0x08, 0x1C, 0x1C, 0x3E, 0x7F, 0x14, 0x22, 0x7F, 0x22, 0x14, 0x5F, 0x5F, 0x00, 0x5F, 0x5F, 0x06, 0x09, 0x7F, 0x01, 0x7F, 0x00, 0x66, 0x89, 0x95, 0x6A, 0x60, 0x60, 0x60, 0x60, 0x60, 0x94, 0xA2, 0xFF, 0xA2, 0x94, 0x08, 0x04, 0x7E, 0x04, 0x08, 0x10, 0x20, 0x7E, 0x20, 0x10, 0x08, 0x08, 0x2A, 0x1C, 0x08, 0x08, 0x1C, 0x2A, 0x08, 0x08, 0x1E, 0x10, 0x10, 0x10, 0x10, 0x0C, 0x1E, 0x0C, 0x1E, 0x0C, 0x30, 0x38, 0x3E, 0x38, 0x30, 0x06, 0x0E, 0x3E, 0x0E, 0x06, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x5F, 0x00, 0x00, 0x00, 0x07, 0x00, 0x07, 0x00, 0x14, 0x7F, 0x14, 0x7F, 0x14, 0x24, 0x2A, 0x7F, 0x2A, 0x12, 0x23, 0x13, 0x08, 0x64, 0x62, 0x36, 0x49, 0x56, 0x20, 0x50, 0x00, 0x08, 0x07, 0x03, 0x00, 0x00, 0x1C, 0x22, 0x41, 0x00, 0x00, 0x41, 0x22, 0x1C, 0x00, 0x2A, 0x1C, 0x7F, 0x1C, 0x2A, 0x08, 0x08, 0x3E, 0x08, 0x08, 0x00, 0x80, 0x70, 0x30, 0x00, 0x08, 0x08, 0x08, 0x08, 0x08, 0x00, 0x00, 0x60, 0x60, 0x00, 0x20, 0x10, 0x08, 0x04, 0x02, 0x3E, 0x51, 0x49, 0x45, 0x3E, 0x00, 0x42, 0x7F, 0x40, 0x00, 0x72, 0x49, 0x49, 0x49, 0x46, 0x21, 0x41, 0x49, 0x4D, 0x33, 0x18, 0x14, 0x12, 0x7F, 0x10, 0x27, 0x45, 0x45, 0x45, 0x39, 0x3C, 0x4A, 0x49, 0x49, 0x31, 0x41, 0x21, 0x11, 0x09, 0x07, 0x36, 0x49, 0x49, 0x49, 0x36, 0x46, 0x49, 0x49, 0x29, 0x1E, 0x00, 0x00, 0x14, 0x00, 0x00, 0x00, 0x40, 0x34, 0x00, 0x00, 0x00, 0x08, 0x14, 0x22, 0x41, 0x14, 0x14, 0x14, 0x14, 0x14, 0x00, 0x41, 0x22, 0x14, 0x08, 0x02, 0x01, 0x59, 0x09, 0x06, 0x3E, 0x41, 0x5D, 0x59, 0x4E, 0x7C, 0x12, 0x11, 0x12, 0x7C, 0x7F, 0x49, 0x49, 0x49, 0x36, 0x3E, 0x41, 0x41, 0x41, 0x22, 0x7F, 0x41, 0x41, 0x41, 0x3E, 0x7F, 0x49, 0x49, 0x49, 0x41, 0x7F, 0x09, 0x09, 0x09, 0x01, 0x3E, 0x41, 0x41, 0x51, 0x73, 0x7F, 0x08, 0x08, 0x08, 0x7F, 0x00, 0x41, 0x7F, 0x41, 0x00, 0x20, 0x40, 0x41, 0x3F, 0x01, 0x7F, 0x08, 0x14, 0x22, 0x41, 0x7F, 0x40, 0x40, 0x40, 0x40, 0x7F, 0x02, 0x1C, 0x02, 0x7F, 0x7F, 0x04, 0x08, 0x10, 0x7F, 0x3E, 0x41, 0x41, 0x41, 0x3E, 0x7F, 0x09, 0x09, 0x09, 0x06, 0x3E, 0x41, 0x51, 0x21, 0x5E, 0x7F, 0x09, 0x19, 0x29, 0x46, 0x26, 0x49, 0x49, 0x49, 0x32, 0x03, 0x01, 0x7F, 0x01, 0x03, 0x3F, 0x40, 0x40, 0x40, 0x3F, 0x1F, 0x20, 0x40, 0x20, 0x1F, 0x3F, 0x40, 0x38, 0x40, 0x3F, 0x63, 0x14, 0x08, 0x14, 0x63, 0x03, 0x04, 0x78, 0x04, 0x03, 0x61, 0x59, 0x49, 0x4D, 0x43, 0x00, 0x7F, 0x41, 0x41, 0x41, 0x02, 0x04, 0x08, 0x10, 0x20, 0x00, 0x41, 0x41, 0x41, 0x7F, 0x04, 0x02, 0x01, 0x02, 0x04, 0x40, 0x40, 0x40, 0x40, 0x40, 0x00, 0x03, 0x07, 0x08, 0x00, 0x20, 0x54, 0x54, 0x78, 0x40, 0x7F, 0x28, 0x44, 0x44, 0x38, 0x38, 0x44, 0x44, 0x44, 0x28, 0x38, 0x44, 0x44, 0x28, 0x7F, 0x38, 0x54, 0x54, 0x54, 0x18, 0x00, 0x08, 0x7E, 0x09, 0x02, 0x18, 0xA4, 0xA4, 0x9C, 0x78, 0x7F, 0x08, 0x04, 0x04, 0x78, 0x00, 0x44, 0x7D, 0x40, 0x00, 0x20, 0x40, 0x40, 0x3D, 0x00, 0x7F, 0x10, 0x28, 0x44, 0x00, 0x00, 0x41, 0x7F, 0x40, 0x00, 0x7C, 0x04, 0x78, 0x04, 0x78, 0x7C, 0x08, 0x04, 0x04, 0x78, 0x38, 0x44, 0x44, 0x44, 0x38, 0xFC, 0x18, 0x24, 0x24, 0x18, 0x18, 0x24, 0x24, 0x18, 0xFC, 0x7C, 0x08, 0x04, 0x04, 0x08, 0x48, 0x54, 0x54, 0x54, 0x24, 0x04, 0x04, 0x3F, 0x44, 0x24, 0x3C, 0x40, 0x40, 0x20, 0x7C, 0x1C, 0x20, 0x40, 0x20, 0x1C, 0x3C, 0x40, 0x30, 0x40, 0x3C, 0x44, 0x28, 0x10, 0x28, 0x44, 0x4C, 0x90, 0x90, 0x90, 0x7C, 0x44, 0x64, 0x54, 0x4C, 0x44, 0x00, 0x08, 0x36, 0x41, 0x00, 0x00, 0x00, 0x77, 0x00, 0x00, 0x00, 0x41, 0x36, 0x08, 0x00, 0x02, 0x01, 0x02, 0x04, 0x02, 0x3C, 0x26, 0x23, 0x26, 0x3C, 0x1E, 0xA1, 0xA1, 0x61, 0x12, 0x3A, 0x40, 0x40, 0x20, 0x7A, 0x38, 0x54, 0x54, 0x55, 0x59, 0x21, 0x55, 0x55, 0x79, 0x41, 0x22, 0x54, 0x54, 0x78, 0x42, 0x21, 0x55, 0x54, 0x78, 0x40, 0x20, 0x54, 0x55, 0x79, 0x40, 0x0C, 0x1E, 0x52, 0x72, 0x12, 0x39, 0x55, 0x55, 0x55, 0x59, 0x39, 0x54, 0x54, 0x54, 0x59, 0x39, 0x55, 0x54, 0x54, 0x58, 0x00, 0x00, 0x45, 0x7C, 0x41, 0x00, 0x02, 0x45, 0x7D, 0x42, 0x00, 0x01, 0x45, 0x7C, 0x40, 0x7D, 0x12, 0x11, 0x12, 0x7D, 0xF0, 0x28, 0x25, 0x28, 0xF0, 0x7C, 0x54, 0x55, 0x45, 0x00, 0x20, 0x54, 0x54, 0x7C, 0x54, 0x7C, 0x0A, 0x09, 0x7F, 0x49, 0x32, 0x49, 0x49, 0x49, 0x32, 0x3A, 0x44, 0x44, 0x44, 0x3A, 0x32, 0x4A, 0x48, 0x48, 0x30, 0x3A, 0x41, 0x41, 0x21, 0x7A, 0x3A, 0x42, 0x40, 0x20, 0x78, 0x00, 0x9D, 0xA0, 0xA0, 0x7D, 0x3D, 0x42, 0x42, 0x42, 0x3D, 0x3D, 0x40, 0x40, 0x40, 0x3D, 0x3C, 0x24, 0xFF, 0x24, 0x24, 0x48, 0x7E, 0x49, 0x43, 0x66, 0x2B, 0x2F, 0xFC, 0x2F, 0x2B, 0xFF, 0x09, 0x29, 0xF6, 0x20, 0xC0, 0x88, 0x7E, 0x09, 0x03, 0x20, 0x54, 0x54, 0x79, 0x41, 0x00, 0x00, 0x44, 0x7D, 0x41, 0x30, 0x48, 0x48, 0x4A, 0x32, 0x38, 0x40, 0x40, 0x22, 0x7A, 0x00, 0x7A, 0x0A, 0x0A, 0x72, 0x7D, 0x0D, 0x19, 0x31, 0x7D, 0x26, 0x29, 0x29, 0x2F, 0x28, 0x26, 0x29, 0x29, 0x29, 0x26, 0x30, 0x48, 0x4D, 0x40, 0x20, 0x38, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x38, 0x2F, 0x10, 0xC8, 0xAC, 0xBA, 0x2F, 0x10, 0x28, 0x34, 0xFA, 0x00, 0x00, 0x7B, 0x00, 0x00, 0x08, 0x14, 0x2A, 0x14, 0x22, 0x22, 0x14, 0x2A, 0x14, 0x08, 0x55, 0x00, 0x55, 0x00, 0x55, 0xAA, 0x55, 0xAA, 0x55, 0xAA, 0xFF, 0x55, 0xFF, 0x55, 0xFF, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x10, 0x10, 0x10, 0xFF, 0x00, 0x14, 0x14, 0x14, 0xFF, 0x00, 0x10, 0x10, 0xFF, 0x00, 0xFF, 0x10, 0x10, 0xF0, 0x10, 0xF0, 0x14, 0x14, 0x14, 0xFC, 0x00, 0x14, 0x14, 0xF7, 0x00, 0xFF, 0x00, 0x00, 0xFF, 0x00, 0xFF, 0x14, 0x14, 0xF4, 0x04, 0xFC, 0x14, 0x14, 0x17, 0x10, 0x1F, 0x10, 0x10, 0x1F, 0x10, 0x1F, 0x14, 0x14, 0x14, 0x1F, 0x00, 0x10, 0x10, 0x10, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x10, 0x10, 0x10, 0x10, 0x1F, 0x10, 0x10, 0x10, 0x10, 0xF0, 0x10, 0x00, 0x00, 0x00, 0xFF, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0xFF, 0x10, 0x00, 0x00, 0x00, 0xFF, 0x14, 0x00, 0x00, 0xFF, 0x00, 0xFF, 0x00, 0x00, 0x1F, 0x10, 0x17, 0x00, 0x00, 0xFC, 0x04, 0xF4, 0x14, 0x14, 0x17, 0x10, 0x17, 0x14, 0x14, 0xF4, 0x04, 0xF4, 0x00, 0x00, 0xFF, 0x00, 0xF7, 0x14, 0x14, 0x14, 0x14, 0x14, 0x14, 0x14, 0xF7, 0x00, 0xF7, 0x14, 0x14, 0x14, 0x17, 0x14, 0x10, 0x10, 0x1F, 0x10, 0x1F, 0x14, 0x14, 0x14, 0xF4, 0x14, 0x10, 0x10, 0xF0, 0x10, 0xF0, 0x00, 0x00, 0x1F, 0x10, 0x1F, 0x00, 0x00, 0x00, 0x1F, 0x14, 0x00, 0x00, 0x00, 0xFC, 0x14, 0x00, 0x00, 0xF0, 0x10, 0xF0, 0x10, 0x10, 0xFF, 0x10, 0xFF, 0x14, 0x14, 0x14, 0xFF, 0x14, 0x10, 0x10, 0x10, 0x1F, 0x00, 0x00, 0x00, 0x00, 0xF0, 0x10, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x38, 0x44, 0x44, 0x38, 0x44, 0xFC, 0x4A, 0x4A, 0x4A, 0x34, 0x7E, 0x02, 0x02, 0x06, 0x06, 0x02, 0x7E, 0x02, 0x7E, 0x02, 0x63, 0x55, 0x49, 0x41, 0x63, 0x38, 0x44, 0x44, 0x3C, 0x04, 0x40, 0x7E, 0x20, 0x1E, 0x20, 0x06, 0x02, 0x7E, 0x02, 0x02, 0x99, 0xA5, 0xE7, 0xA5, 0x99, 0x1C, 0x2A, 0x49, 0x2A, 0x1C, 0x4C, 0x72, 0x01, 0x72, 0x4C, 0x30, 0x4A, 0x4D, 0x4D, 0x30, 0x30, 0x48, 0x78, 0x48, 0x30, 0xBC, 0x62, 0x5A, 0x46, 0x3D, 0x3E, 0x49, 0x49, 0x49, 0x00, 0x7E, 0x01, 0x01, 0x01, 0x7E, 0x2A, 0x2A, 0x2A, 0x2A, 0x2A, 0x44, 0x44, 0x5F, 0x44, 0x44, 0x40, 0x51, 0x4A, 0x44, 0x40, 0x40, 0x44, 0x4A, 0x51, 0x40, 0x00, 0x00, 0xFF, 0x01, 0x03, 0xE0, 0x80, 0xFF, 0x00, 0x00, 0x08, 0x08, 0x6B, 0x6B, 0x08, 0x36, 0x12, 0x36, 0x24, 0x36, 0x06, 0x0F, 0x09, 0x0F, 0x06, 0x00, 0x00, 0x18, 0x18, 0x00, 0x00, 0x00, 0x10, 0x10, 0x00, 0x30, 0x40, 0xFF, 0x01, 0x01, 0x00, 0x1F, 0x01, 0x01, 0x1E, 0x00, 0x19, 0x1D, 0x17, 0x12, 0x00, 0x3C, 0x3C, 0x3C, 0x3C, 0x00, 0x00, 0x00, 0x00, 0x00 }; //***** Functions prototypes *****// //1. Write Command to LCD void ILI9341_SendCommand(uint8_t com); //2. Write data to LCD void ILI9341_SendData(uint8_t data); //2.2 Write multiple/DMA void ILI9341_SendData_Multi(uint16_t Colordata, uint32_t size); //3. Set cursor position void ILI9341_SetCursorPosition(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); //4. Initialise function void ILI9341_Init(); //5. Write data to a single pixel void ILI9341_DrawPixel(uint16_t x, uint16_t y, uint16_t color); //Draw single pixel to ILI9341 //6. Fill the entire screen with a background color void ILI9341_Fill(uint16_t color); //Fill entire ILI9341 with color //7. Rectangle drawing functions void ILI9341_Fill_Rect(unsigned int x0, unsigned int y0, unsigned int x1, unsigned int y1, uint16_t color); //8. Circle drawing functions void ILI9341_drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color); static void drawCircleHelper( int16_t x0, int16_t y0, int16_t r, uint8_t cornername, uint16_t color); static void fillCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, int16_t delta, uint16_t color); void ILI9341_fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color); //9. Line drawing functions void ILI9341_drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color); void ILI9341_drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color); void ILI9341_drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); //10. Triangle drawing void ILI9341_drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color); void ILI9341_fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color); //11. Text printing functions //void ILI9341_drawChar(int16_t x, int16_t y, unsigned char c, uint16_t color, uint16_t bg, uint8_t size); //void ILI9341_printText(char text[], int16_t x, int16_t y, uint16_t color, uint16_t bg, uint8_t size); //12. Image print (RGB 565, 2 bytes per pixel) void ILI9341_printImage(uint16_t x, uint16_t y, uint16_t w, uint16_t h, uint8_t *data, uint32_t size); //13. Set screen rotation void ILI9341_setRotation(uint8_t rotate); //14. Get screen rotation uint8_t ILI9341_getRotation(void); //draw a single character void ILI9341_DrawChar(int16_t x, int16_t y, char c, int16_t textColor, int16_t bgColor, uint8_t size); //sensd 16-bit color data uint16_t ILI9341_Color565(uint8_t r, uint8_t g, uint8_t b); //write a string to the lcd uint32_t ILI9341_DrawString(uint16_t x, uint16_t y, char *pt, int16_t textColor,int16_t BG,uint16_t size); #endif
Now, create new source file with name of ILI9341.c.
Within the source file, include the following:
//most of the functions has been documented in the interface file (ILI9341.h) #include "ILI9341.h" #include "LCD_Pins.h"
Variables needed for calculations:
static uint8_t rotationNum=1; static uint8_t ColStart, RowStart;
For the initialization function:
//initialize the tft void ILI9341_Init(void) { LCD_Write_Cmd (ILI9341_DISPLAY_OFF); // display off //------------power control------------------------------ LCD_Write_Cmd (ILI9341_POWER1); // power control LCD_Write_Data (0x26); // GVDD = 4.75v LCD_Write_Cmd (ILI9341_POWER2); // power control LCD_Write_Data (0x11); // AVDD=VCIx2, VGH=VCIx7, VGL=-VCIx3 //--------------VCOM------------------------------------- LCD_Write_Cmd (ILI9341_VCOM1); // vcom control LCD_Write_Data (0x35); // Set the VCOMH voltage (0x35 = 4.025v) LCD_Write_Data (0x3e); // Set the VCOML voltage (0x3E = -0.950v) LCD_Write_Cmd (ILI9341_VCOM2); // vcom control LCD_Write_Data (0xbe); //------------memory access control------------------------ LCD_Write_Cmd (ILI9341_MAC); // memory access control LCD_Write_Data(0x48); LCD_Write_Cmd (ILI9341_PIXEL_FORMAT); // pixel format set LCD_Write_Data (0x55); // 16bit /pixel LCD_Write_Cmd(ILI9341_FRC); LCD_Write_Data(0); LCD_Write_Data(0x1F); //-------------ddram ---------------------------- LCD_Write_Cmd (ILI9341_COLUMN_ADDR); // column set LCD_Write_Data (0x00); // x0_HIGH---0 LCD_Write_Data (0x00); // x0_LOW----0 LCD_Write_Data (0x00); // x1_HIGH---240 LCD_Write_Data (0x1D); // x1_LOW----240 LCD_Write_Cmd (ILI9341_PAGE_ADDR); // page address set LCD_Write_Data (0x00); // y0_HIGH---0 LCD_Write_Data (0x00); // y0_LOW----0 LCD_Write_Data (0x00); // y1_HIGH---320 LCD_Write_Data (0x27); // y1_LOW----320 LCD_Write_Cmd (ILI9341_TEARING_OFF); // tearing effect off //LCD_write_cmd(ILI9341_TEARING_ON); // tearing effect on //LCD_write_cmd(ILI9341_DISPLAY_INVERSION); // display inversion LCD_Write_Cmd (ILI9341_Entry_Mode_Set); // entry mode set // Deep Standby Mode: OFF // Set the output level of gate driver G1-G320: Normal display // Low voltage detection: Disable LCD_Write_Data (0x07); //-----------------display------------------------ LCD_Write_Cmd (ILI9341_DFC); // display function control //Set the scan mode in non-display area //Determine source/VCOM output in a non-display area in the partial display mode LCD_Write_Data (0x0a); //Select whether the liquid crystal type is normally white type or normally black type //Sets the direction of scan by the gate driver in the range determined by SCN and NL //Select the shift direction of outputs from the source driver //Sets the gate driver pin arrangement in combination with the GS bit to select the optimal scan mode for the module //Specify the scan cycle interval of gate driver in non-display area when PTG to select interval scan LCD_Write_Data (0x82); // Sets the number of lines to drive the LCD at an interval of 8 lines LCD_Write_Data (0x27); LCD_Write_Data (0x00); // clock divisor LCD_Write_Cmd (ILI9341_SLEEP_OUT); // sleep out delay(100); LCD_Write_Cmd (ILI9341_DISPLAY_ON); // display on delay(100); LCD_Write_Cmd (ILI9341_GRAM); // memory write delay(5); }
3. Supporting functions:
//set the address of the pixel in the memory void static setAddrWindow(uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1) { LCD_Write_Cmd(0x2A); // Column addr set LCD_Write_Data(0x00); LCD_Write_Data(x0+ColStart); // XSTART LCD_Write_Data(0x00); LCD_Write_Data(x1+ColStart); // XEND LCD_Write_Cmd(0x2B); // Row addr set LCD_Write_Data(0x00); LCD_Write_Data(y0+RowStart); // YSTART LCD_Write_Data(0x00); LCD_Write_Data(y1+RowStart); // YEND LCD_Write_Cmd(0x2C); // write to RAM } //internally needed function to push 16-bit color as 2 8-bit data void static pushColor(uint16_t color) { LCD_Write_Data((uint8_t)(color >> 8)); LCD_Write_Data((uint8_t)color); } //set cursor function void ILI9341_SetCursorPosition(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) { LCD_Write_Cmd (ILI9341_COLUMN_ADDR); LCD_Write_Data(x1>>8); LCD_Write_Data(x1 & 0xFF); LCD_Write_Data(x2>>8); LCD_Write_Data(x2 & 0xFF); LCD_Write_Cmd (ILI9341_PAGE_ADDR); LCD_Write_Data(y1>>8); LCD_Write_Data(y1 & 0xFF); LCD_Write_Data(y2>>8); LCD_Write_Data(y2 & 0xFF); LCD_Write_Cmd (ILI9341_GRAM); } void ILI9341_DrawPixel(uint16_t x, uint16_t y, uint16_t color) { ILI9341_SetCursorPosition(x, y, x, y); LCD_Write_Data(color>>8); LCD_Write_Data(color&0xFF); } void ILI9341_Fill(uint16_t color) { uint32_t n = ILI9341_PIXEL_COUNT; uint16_t myColor = 0xFF; if(rotationNum==1 || rotationNum==3) { ILI9341_SetCursorPosition(0, 0, ILI9341_WIDTH -1, ILI9341_HEIGHT -1); } else if(rotationNum==2 || rotationNum==4) { ILI9341_SetCursorPosition(0, 0, ILI9341_HEIGHT -1, ILI9341_WIDTH -1); } while (n) { n--; LCD_Write_Data(color>>8); LCD_Write_Data(color&0xff); } } void ILI9341_Fill_Rect(unsigned int x0,unsigned int y0, unsigned int x1,unsigned int y1, uint16_t color) { uint32_t n = ((x1+1)-x0)*((y1+1)-y0); if (n>ILI9341_PIXEL_COUNT) n=ILI9341_PIXEL_COUNT; ILI9341_SetCursorPosition(x0, y0, x1, y1); while (n) { n--; LCD_Write_Data(color>>8); LCD_Write_Data(color&0xff); } } void ILI9341_drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color) { int16_t f = 1 - r; int16_t ddF_x = 1; int16_t ddF_y = -2 * r; int16_t x = 0; int16_t y = r; ILI9341_DrawPixel(x0 , y0+r, color); ILI9341_DrawPixel(x0 , y0-r, color); ILI9341_DrawPixel(x0+r, y0 , color); ILI9341_DrawPixel(x0-r, y0 , color); while (x<y) { if (f >= 0) { y--; ddF_y += 2; f += ddF_y; } x++; ddF_x += 2; f += ddF_x; ILI9341_DrawPixel(x0 + x, y0 + y, color); ILI9341_DrawPixel(x0 - x, y0 + y, color); ILI9341_DrawPixel(x0 + x, y0 - y, color); ILI9341_DrawPixel(x0 - x, y0 - y, color); ILI9341_DrawPixel(x0 + y, y0 + x, color); ILI9341_DrawPixel(x0 - y, y0 + x, color); ILI9341_DrawPixel(x0 + y, y0 - x, color); ILI9341_DrawPixel(x0 - y, y0 - x, color); } } static void drawCircleHelper( int16_t x0, int16_t y0, int16_t r, uint8_t cornername, uint16_t color) { int16_t f = 1 - r; int16_t ddF_x = 1; int16_t ddF_y = -2 * r; int16_t x = 0; int16_t y = r; while (x<y) { if (f >= 0) { y--; ddF_y += 2; f += ddF_y; } x++; ddF_x += 2; f+= ddF_x; if (cornername & 0x4) { ILI9341_DrawPixel(x0 + x, y0 + y, color); ILI9341_DrawPixel(x0 + y, y0 + x, color); } if (cornername & 0x2) { ILI9341_DrawPixel(x0 + x, y0 - y, color); ILI9341_DrawPixel(x0 + y, y0 - x, color); } if (cornername & 0x8) { ILI9341_DrawPixel(x0 - y, y0 + x, color); ILI9341_DrawPixel(x0 - x, y0 + y, color); } if (cornername & 0x1) { ILI9341_DrawPixel(x0 - y, y0 - x, color); ILI9341_DrawPixel(x0 - x, y0 - y, color); } } } static void fillCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, int16_t delta, uint16_t color) { int16_t f = 1 - r; int16_t ddF_x = 1; int16_t ddF_y = -2 * r; int16_t x = 0; int16_t y = r; while (x<y) { if (f >= 0) { y--; ddF_y += 2; f+= ddF_y; } x++; ddF_x += 2; f += ddF_x; if (cornername & 0x1) { ILI9341_drawFastVLine(x0+x, y0-y, 2*y+1+delta, color); ILI9341_drawFastVLine(x0+y, y0-x, 2*x+1+delta, color); } if (cornername & 0x2) { ILI9341_drawFastVLine(x0-x, y0-y, 2*y+1+delta, color); ILI9341_drawFastVLine(x0-y, y0-x, 2*x+1+delta, color); } } } void ILI9341_fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color) { ILI9341_drawFastVLine(x0, y0-r, 2*r+1, color); fillCircleHelper(x0, y0, r, 3, 0, color); } void ILI9341_drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color) { int16_t steep = abs(y1 - y0) > abs(x1 - x0); if (steep) { swap(x0, y0); swap(x1, y1); } if (x0 > x1) { swap(x0, x1); swap(y0, y1); } int16_t dx, dy; dx = x1 - x0; dy = abs(y1 - y0); int16_t err = dx / 2; int16_t ystep; if (y0 < y1) { ystep = 1; } else { ystep = -1; } for (; x0<=x1; x0++) { if (steep) { ILI9341_DrawPixel(y0, x0, color); } else { ILI9341_DrawPixel(x0, y0, color); } err -= dy; if (err < 0) { y0 += ystep; err += dx; } } } void ILI9341_drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color) { ILI9341_drawLine(x, y, x+w-1, y, color); } void ILI9341_drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color) { ILI9341_drawLine(x, y, x, y+h-1, color); } //10. Triangle drawing void ILI9341_drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color) { ILI9341_drawLine(x0, y0, x1, y1, color); ILI9341_drawLine(x1, y1, x2, y2, color); ILI9341_drawLine(x2, y2, x0, y0, color); } void ILI9341_fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color) { int16_t a, b, y, last; // Sort coordinates by Y order (y2 >= y1 >= y0) if (y0 > y1) { swap(y0, y1); swap(x0, x1); } if (y1 > y2) { swap(y2, y1); swap(x2, x1); } if (y0 > y1) { swap(y0, y1); swap(x0, x1); } if(y0 == y2) { // Handle awkward all-on-same-line case as its own thing a = b = x0; if(x1 < a) a = x1; else if(x1 > b) b = x1; if(x2 < a) a = x2; else if(x2 > b) b = x2; ILI9341_drawFastHLine(a, y0, b-a+1, color); return; } int16_t dx01 = x1 - x0, dy01 = y1 - y0, dx02 = x2 - x0, dy02 = y2 - y0, dx12 = x2 - x1, dy12 = y2 - y1, sa = 0, sb = 0; // For upper part of triangle, find scanline crossings for segments // 0-1 and 0-2. If y1=y2 (flat-bottomed triangle), the scanline y1 // is included here (and second loop will be skipped, avoiding a /0 // error there), otherwise scanline y1 is skipped here and handled // in the second loop...which also avoids a /0 error here if y0=y1 // (flat-topped triangle). if(y1 == y2) last = y1; // Include y1 scanline else last = y1-1; // Skip it for(y=y0; y<=last; y++) { a = x0 + sa / dy01; b = x0 + sb / dy02; sa += dx01; sb += dx02; if(a > b) swap(a,b); ILI9341_drawFastHLine(a, y, b-a+1, color); } // For lower part of triangle, find scanline crossings for segments // 0-2 and 1-2. This loop is skipped if y1=y2. sa = dx12 * (y - y1); sb = dx02 * (y - y0); for(; y<=y2; y++) { a = x1 + sa / dy12; b = x0 + sb / dy02; sa += dx12; sb += dx02; if(a > b) swap(a,b); ILI9341_drawFastHLine(a, y, b-a+1, color); } } void ILI9341_setRotation(uint8_t rotate) { switch(rotate) { case 1: rotationNum = 1; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MY | ILI9341_MADCTL_BGR); break; case 2: rotationNum = 2; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MV | ILI9341_MADCTL_BGR); break; case 3: rotationNum = 3; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MX | ILI9341_MADCTL_BGR); break; case 4: rotationNum = 4; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MX | ILI9341_MADCTL_MY | ILI9341_MADCTL_MV | ILI9341_MADCTL_BGR); break; default: rotationNum = 1; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MY | ILI9341_MADCTL_BGR); break; } } void ILI9341_DrawChar(int16_t x, int16_t y, char c, int16_t textColor, int16_t bgColor, uint8_t size){ uint8_t line; // horizontal row of pixels of character int32_t col, row, i, j;// loop indices if(((x + 5*size - 1) >= ILI9341_WIDTH) || // Clip right ((y + 8*size - 1) >= ILI9341_HEIGHT) || // Clip bottom ((x + 5*size - 1) < 0) || // Clip left ((y + 8*size - 1) < 0)){ // Clip top return; } setAddrWindow(x, y, x+6*size-1, y+8*size-1); line = 0x01; // print the top row first // print the rows, starting at the top for(row=0; row<8; row=row+1){ for(i=0; i<size; i=i+1){ // print the columns, starting on the left for(col=0; col<5; col=col+1){ if(Font[(c*5)+col]&line){ // bit is set in Font, print pixel(s) in text color for(j=0; j<size; j=j+1){ pushColor(textColor); } } else{ // bit is cleared in Font, print pixel(s) in background color for(j=0; j<size; j=j+1){ pushColor(bgColor); } } } // print blank column(s) to the right of character for(j=0; j<size; j=j+1){ pushColor(bgColor); } } line = line<<1; // move up to the next row } } uint16_t ILI9341_Color565(uint8_t r, uint8_t g, uint8_t b) { return ((b & 0xF8) << 8) | ((g & 0xFC) << 3) | (r >> 3); } uint32_t ILI9341_DrawString(uint16_t x, uint16_t y, char *pt, int16_t textColor, int16_t BG,uint16_t size){ uint32_t count = 0; while(*pt!='\0') { ILI9341_DrawChar(x*6*size, y*10*size, *pt, textColor, BG, size); pt++; x = x+1; count++; } return count; // number of characters printed }
Hence, the entire source code as following:
//most of the functions has been documented in the interface file (ILI9341.h) #include "ILI9341.h" #include "LCD_Pins.h" static uint8_t rotationNum=1; static uint8_t ColStart, RowStart; //initialize the tft void ILI9341_Init(void) { LCD_Write_Cmd (ILI9341_DISPLAY_OFF); // display off //------------power control------------------------------ LCD_Write_Cmd (ILI9341_POWER1); // power control LCD_Write_Data (0x26); // GVDD = 4.75v LCD_Write_Cmd (ILI9341_POWER2); // power control LCD_Write_Data (0x11); // AVDD=VCIx2, VGH=VCIx7, VGL=-VCIx3 //--------------VCOM------------------------------------- LCD_Write_Cmd (ILI9341_VCOM1); // vcom control LCD_Write_Data (0x35); // Set the VCOMH voltage (0x35 = 4.025v) LCD_Write_Data (0x3e); // Set the VCOML voltage (0x3E = -0.950v) LCD_Write_Cmd (ILI9341_VCOM2); // vcom control LCD_Write_Data (0xbe); //------------memory access control------------------------ LCD_Write_Cmd (ILI9341_MAC); // memory access control LCD_Write_Data(0x48); LCD_Write_Cmd (ILI9341_PIXEL_FORMAT); // pixel format set LCD_Write_Data (0x55); // 16bit /pixel LCD_Write_Cmd(ILI9341_FRC); LCD_Write_Data(0); LCD_Write_Data(0x1F); //-------------ddram ---------------------------- LCD_Write_Cmd (ILI9341_COLUMN_ADDR); // column set LCD_Write_Data (0x00); // x0_HIGH---0 LCD_Write_Data (0x00); // x0_LOW----0 LCD_Write_Data (0x00); // x1_HIGH---240 LCD_Write_Data (0x1D); // x1_LOW----240 LCD_Write_Cmd (ILI9341_PAGE_ADDR); // page address set LCD_Write_Data (0x00); // y0_HIGH---0 LCD_Write_Data (0x00); // y0_LOW----0 LCD_Write_Data (0x00); // y1_HIGH---320 LCD_Write_Data (0x27); // y1_LOW----320 LCD_Write_Cmd (ILI9341_TEARING_OFF); // tearing effect off //LCD_write_cmd(ILI9341_TEARING_ON); // tearing effect on //LCD_write_cmd(ILI9341_DISPLAY_INVERSION); // display inversion LCD_Write_Cmd (ILI9341_Entry_Mode_Set); // entry mode set // Deep Standby Mode: OFF // Set the output level of gate driver G1-G320: Normal display // Low voltage detection: Disable LCD_Write_Data (0x07); //-----------------display------------------------ LCD_Write_Cmd (ILI9341_DFC); // display function control //Set the scan mode in non-display area //Determine source/VCOM output in a non-display area in the partial display mode LCD_Write_Data (0x0a); //Select whether the liquid crystal type is normally white type or normally black type //Sets the direction of scan by the gate driver in the range determined by SCN and NL //Select the shift direction of outputs from the source driver //Sets the gate driver pin arrangement in combination with the GS bit to select the optimal scan mode for the module //Specify the scan cycle interval of gate driver in non-display area when PTG to select interval scan LCD_Write_Data (0x82); // Sets the number of lines to drive the LCD at an interval of 8 lines LCD_Write_Data (0x27); LCD_Write_Data (0x00); // clock divisor LCD_Write_Cmd (ILI9341_SLEEP_OUT); // sleep out delay(100); LCD_Write_Cmd (ILI9341_DISPLAY_ON); // display on delay(100); LCD_Write_Cmd (ILI9341_GRAM); // memory write delay(5); } //set the address of the pixel in the memory void static setAddrWindow(uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1) { LCD_Write_Cmd(0x2A); // Column addr set LCD_Write_Data(0x00); LCD_Write_Data(x0+ColStart); // XSTART LCD_Write_Data(0x00); LCD_Write_Data(x1+ColStart); // XEND LCD_Write_Cmd(0x2B); // Row addr set LCD_Write_Data(0x00); LCD_Write_Data(y0+RowStart); // YSTART LCD_Write_Data(0x00); LCD_Write_Data(y1+RowStart); // YEND LCD_Write_Cmd(0x2C); // write to RAM } //internally needed function to push 16-bit color as 2 8-bit data void static pushColor(uint16_t color) { LCD_Write_Data((uint8_t)(color >> 8)); LCD_Write_Data((uint8_t)color); } //set cursor function void ILI9341_SetCursorPosition(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) { LCD_Write_Cmd (ILI9341_COLUMN_ADDR); LCD_Write_Data(x1>>8); LCD_Write_Data(x1 & 0xFF); LCD_Write_Data(x2>>8); LCD_Write_Data(x2 & 0xFF); LCD_Write_Cmd (ILI9341_PAGE_ADDR); LCD_Write_Data(y1>>8); LCD_Write_Data(y1 & 0xFF); LCD_Write_Data(y2>>8); LCD_Write_Data(y2 & 0xFF); LCD_Write_Cmd (ILI9341_GRAM); } void ILI9341_DrawPixel(uint16_t x, uint16_t y, uint16_t color) { ILI9341_SetCursorPosition(x, y, x, y); LCD_Write_Data(color>>8); LCD_Write_Data(color&0xFF); } void ILI9341_Fill(uint16_t color) { uint32_t n = ILI9341_PIXEL_COUNT; uint16_t myColor = 0xFF; if(rotationNum==1 || rotationNum==3) { ILI9341_SetCursorPosition(0, 0, ILI9341_WIDTH -1, ILI9341_HEIGHT -1); } else if(rotationNum==2 || rotationNum==4) { ILI9341_SetCursorPosition(0, 0, ILI9341_HEIGHT -1, ILI9341_WIDTH -1); } while (n) { n--; LCD_Write_Data(color>>8); LCD_Write_Data(color&0xff); } } void ILI9341_Fill_Rect(unsigned int x0,unsigned int y0, unsigned int x1,unsigned int y1, uint16_t color) { uint32_t n = ((x1+1)-x0)*((y1+1)-y0); if (n>ILI9341_PIXEL_COUNT) n=ILI9341_PIXEL_COUNT; ILI9341_SetCursorPosition(x0, y0, x1, y1); while (n) { n--; LCD_Write_Data(color>>8); LCD_Write_Data(color&0xff); } } void ILI9341_drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color) { int16_t f = 1 - r; int16_t ddF_x = 1; int16_t ddF_y = -2 * r; int16_t x = 0; int16_t y = r; ILI9341_DrawPixel(x0 , y0+r, color); ILI9341_DrawPixel(x0 , y0-r, color); ILI9341_DrawPixel(x0+r, y0 , color); ILI9341_DrawPixel(x0-r, y0 , color); while (x<y) { if (f >= 0) { y--; ddF_y += 2; f += ddF_y; } x++; ddF_x += 2; f += ddF_x; ILI9341_DrawPixel(x0 + x, y0 + y, color); ILI9341_DrawPixel(x0 - x, y0 + y, color); ILI9341_DrawPixel(x0 + x, y0 - y, color); ILI9341_DrawPixel(x0 - x, y0 - y, color); ILI9341_DrawPixel(x0 + y, y0 + x, color); ILI9341_DrawPixel(x0 - y, y0 + x, color); ILI9341_DrawPixel(x0 + y, y0 - x, color); ILI9341_DrawPixel(x0 - y, y0 - x, color); } } static void drawCircleHelper( int16_t x0, int16_t y0, int16_t r, uint8_t cornername, uint16_t color) { int16_t f = 1 - r; int16_t ddF_x = 1; int16_t ddF_y = -2 * r; int16_t x = 0; int16_t y = r; while (x<y) { if (f >= 0) { y--; ddF_y += 2; f += ddF_y; } x++; ddF_x += 2; f+= ddF_x; if (cornername & 0x4) { ILI9341_DrawPixel(x0 + x, y0 + y, color); ILI9341_DrawPixel(x0 + y, y0 + x, color); } if (cornername & 0x2) { ILI9341_DrawPixel(x0 + x, y0 - y, color); ILI9341_DrawPixel(x0 + y, y0 - x, color); } if (cornername & 0x8) { ILI9341_DrawPixel(x0 - y, y0 + x, color); ILI9341_DrawPixel(x0 - x, y0 + y, color); } if (cornername & 0x1) { ILI9341_DrawPixel(x0 - y, y0 - x, color); ILI9341_DrawPixel(x0 - x, y0 - y, color); } } } static void fillCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, int16_t delta, uint16_t color) { int16_t f = 1 - r; int16_t ddF_x = 1; int16_t ddF_y = -2 * r; int16_t x = 0; int16_t y = r; while (x<y) { if (f >= 0) { y--; ddF_y += 2; f+= ddF_y; } x++; ddF_x += 2; f += ddF_x; if (cornername & 0x1) { ILI9341_drawFastVLine(x0+x, y0-y, 2*y+1+delta, color); ILI9341_drawFastVLine(x0+y, y0-x, 2*x+1+delta, color); } if (cornername & 0x2) { ILI9341_drawFastVLine(x0-x, y0-y, 2*y+1+delta, color); ILI9341_drawFastVLine(x0-y, y0-x, 2*x+1+delta, color); } } } void ILI9341_fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color) { ILI9341_drawFastVLine(x0, y0-r, 2*r+1, color); fillCircleHelper(x0, y0, r, 3, 0, color); } void ILI9341_drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color) { int16_t steep = abs(y1 - y0) > abs(x1 - x0); if (steep) { swap(x0, y0); swap(x1, y1); } if (x0 > x1) { swap(x0, x1); swap(y0, y1); } int16_t dx, dy; dx = x1 - x0; dy = abs(y1 - y0); int16_t err = dx / 2; int16_t ystep; if (y0 < y1) { ystep = 1; } else { ystep = -1; } for (; x0<=x1; x0++) { if (steep) { ILI9341_DrawPixel(y0, x0, color); } else { ILI9341_DrawPixel(x0, y0, color); } err -= dy; if (err < 0) { y0 += ystep; err += dx; } } } void ILI9341_drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color) { ILI9341_drawLine(x, y, x+w-1, y, color); } void ILI9341_drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color) { ILI9341_drawLine(x, y, x, y+h-1, color); } //10. Triangle drawing void ILI9341_drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color) { ILI9341_drawLine(x0, y0, x1, y1, color); ILI9341_drawLine(x1, y1, x2, y2, color); ILI9341_drawLine(x2, y2, x0, y0, color); } void ILI9341_fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color) { int16_t a, b, y, last; // Sort coordinates by Y order (y2 >= y1 >= y0) if (y0 > y1) { swap(y0, y1); swap(x0, x1); } if (y1 > y2) { swap(y2, y1); swap(x2, x1); } if (y0 > y1) { swap(y0, y1); swap(x0, x1); } if(y0 == y2) { // Handle awkward all-on-same-line case as its own thing a = b = x0; if(x1 < a) a = x1; else if(x1 > b) b = x1; if(x2 < a) a = x2; else if(x2 > b) b = x2; ILI9341_drawFastHLine(a, y0, b-a+1, color); return; } int16_t dx01 = x1 - x0, dy01 = y1 - y0, dx02 = x2 - x0, dy02 = y2 - y0, dx12 = x2 - x1, dy12 = y2 - y1, sa = 0, sb = 0; // For upper part of triangle, find scanline crossings for segments // 0-1 and 0-2. If y1=y2 (flat-bottomed triangle), the scanline y1 // is included here (and second loop will be skipped, avoiding a /0 // error there), otherwise scanline y1 is skipped here and handled // in the second loop...which also avoids a /0 error here if y0=y1 // (flat-topped triangle). if(y1 == y2) last = y1; // Include y1 scanline else last = y1-1; // Skip it for(y=y0; y<=last; y++) { a = x0 + sa / dy01; b = x0 + sb / dy02; sa += dx01; sb += dx02; if(a > b) swap(a,b); ILI9341_drawFastHLine(a, y, b-a+1, color); } // For lower part of triangle, find scanline crossings for segments // 0-2 and 1-2. This loop is skipped if y1=y2. sa = dx12 * (y - y1); sb = dx02 * (y - y0); for(; y<=y2; y++) { a = x1 + sa / dy12; b = x0 + sb / dy02; sa += dx12; sb += dx02; if(a > b) swap(a,b); ILI9341_drawFastHLine(a, y, b-a+1, color); } } void ILI9341_setRotation(uint8_t rotate) { switch(rotate) { case 1: rotationNum = 1; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MY | ILI9341_MADCTL_BGR); break; case 2: rotationNum = 2; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MV | ILI9341_MADCTL_BGR); break; case 3: rotationNum = 3; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MX | ILI9341_MADCTL_BGR); break; case 4: rotationNum = 4; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MX | ILI9341_MADCTL_MY | ILI9341_MADCTL_MV | ILI9341_MADCTL_BGR); break; default: rotationNum = 1; LCD_Write_Cmd(ILI9341_MEMCONTROL); LCD_Write_Data(ILI9341_MADCTL_MY | ILI9341_MADCTL_BGR); break; } } void ILI9341_DrawChar(int16_t x, int16_t y, char c, int16_t textColor, int16_t bgColor, uint8_t size){ uint8_t line; // horizontal row of pixels of character int32_t col, row, i, j;// loop indices if(((x + 5*size - 1) >= ILI9341_WIDTH) || // Clip right ((y + 8*size - 1) >= ILI9341_HEIGHT) || // Clip bottom ((x + 5*size - 1) < 0) || // Clip left ((y + 8*size - 1) < 0)){ // Clip top return; } setAddrWindow(x, y, x+6*size-1, y+8*size-1); line = 0x01; // print the top row first // print the rows, starting at the top for(row=0; row<8; row=row+1){ for(i=0; i<size; i=i+1){ // print the columns, starting on the left for(col=0; col<5; col=col+1){ if(Font[(c*5)+col]&line){ // bit is set in Font, print pixel(s) in text color for(j=0; j<size; j=j+1){ pushColor(textColor); } } else{ // bit is cleared in Font, print pixel(s) in background color for(j=0; j<size; j=j+1){ pushColor(bgColor); } } } // print blank column(s) to the right of character for(j=0; j<size; j=j+1){ pushColor(bgColor); } } line = line<<1; // move up to the next row } } uint16_t ILI9341_Color565(uint8_t r, uint8_t g, uint8_t b) { return ((b & 0xF8) << 8) | ((g & 0xFC) << 3) | (r >> 3); } uint32_t ILI9341_DrawString(uint16_t x, uint16_t y, char *pt, int16_t textColor, int16_t BG,uint16_t size){ uint32_t count = 0; while(*pt!='\0') { ILI9341_DrawChar(x*6*size, y*10*size, *pt, textColor, BG, size); pt++; x = x+1; count++; } return count; // number of characters printed }
4. Code:
You may download the source code from here:
5. Demo:
In main.c:
#include "LCD_Pins.h" #include "ILI9341.h" int main(void) { delay_init(180000000); LCD_Pin_Init(); LCD_SPI_Init(); ILI9341_Init(); ILI9341_setRotation(2); ILI9341_Fill(COLOR_RED); while(1) { ILI9341_Fill(COLOR_YELLOW); ILI9341_Fill(COLOR_PURPLE); } }
Upload the code and you should get the following:
Next, touch interface.
Happy coding 🙂
Add Comment