The System tick timer is present in all arm cortex-m microcontrollers. Irrespective of whether it is STM32,LPC, Tiva C etc.
The System tick timer allows the system to initiate an action on a periodic basis. This action is performed internally at a fixed rate without external signal. For example, in a given application we can use SysTick to read a sensor every 200 msec. SysTick is used widely by operating systems so that the system software may interrupt the application software periodically (often 10 ms interval) to monitor and control the system operations. The SysTick is a 24-bit down counter driven by either the system clock or the internal oscillator. It counts down from an initial value to 0. When it reaches 0, in the next clock, it underflows and it raises a flag called COUNT and reloads the initial value and starts all over.
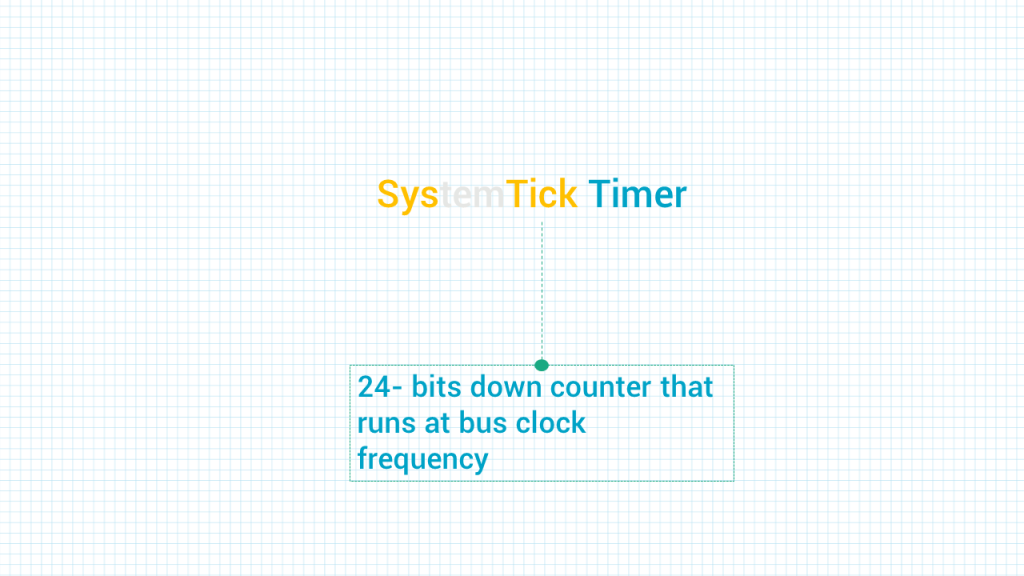
Systick Register
Lets see the systick registers. There are three registers in the SysTick module: SysTick Control and Status register, SysTick Reload Value register, and SysTick Current Value register.
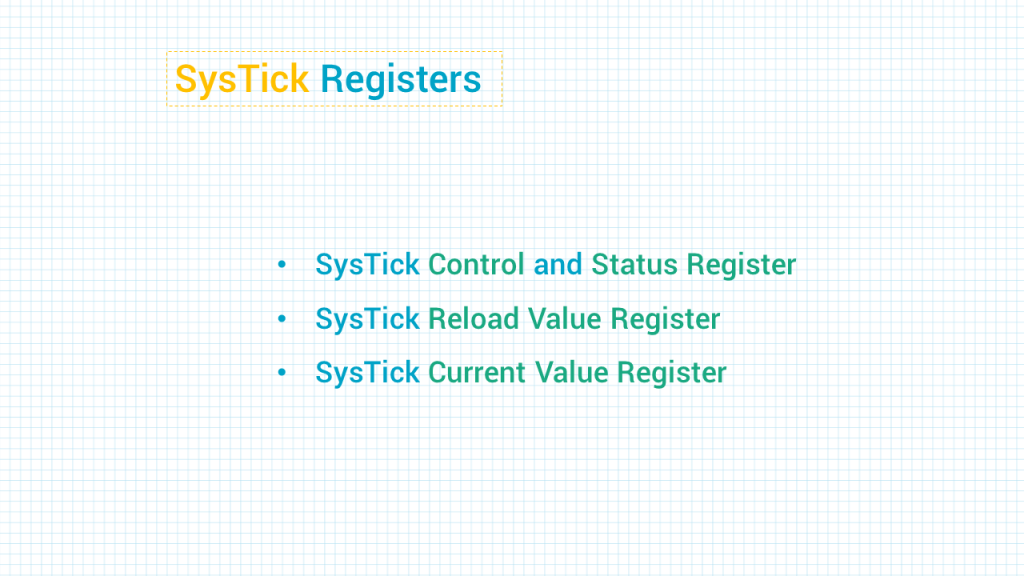
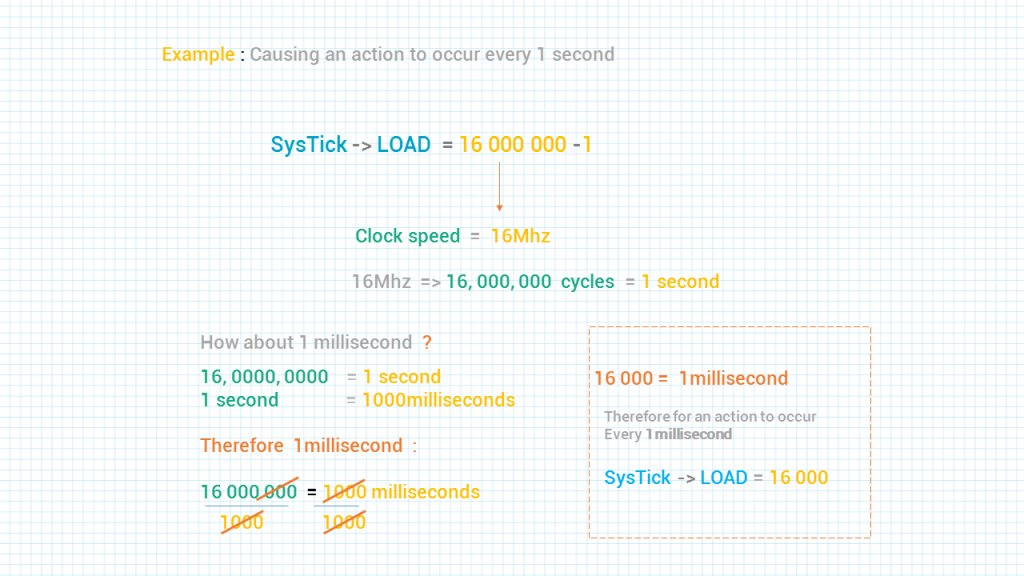
SYSTICK IMPLEMENTATION
/* Configure SysTick to generate 500 ms delay to * toggle the board LED. */ #include "stm32f4xx.h" int main(void) { RCC->AHB1ENR |= 1; /* enable GPIOA clock */ GPIOA->MODER &= ~0x00000C00; /* clear pin mode */ GPIOA->MODER |= 0x00000400; /* set pin to output mode */ /* Configure SysTick */ SysTick->LOAD = 3200000 - 1; /* reload with number of clocks per second */ SysTick->VAL = 0; SysTick->CTRL = 5; /* enable it, no interrupt, use system clock */ while (1) { if (SysTick->CTRL & 0x10000) { /* if COUNT flag is set */ GPIOA->ODR ^= 0x00000020; /* toggle green LED */ } } }
1 Comment
good one
Add Comment