
In the previous guide (here), we took a look at the PWM mode in timer2 of STM32F767. In this guide, we shall take a look at the output compare where the the output will be toggled each time the timer overflow.
In this guide we cover the following:
- What is output compare mode
- Required parts and connection
- Code
- Demo
1. What is output compare mode:
In this mode, the timer shall count to the maximum level (set by the ARR), the following will happen:
- Assigns the corresponding output pin to a programmable value defined by the output compare mode.
- Sets a flag in the interrupt status register.
- Generates an interrupt if the corresponding interrupt mask is set.
- Sends a DMA request if the corresponding enable bit is set.
In our case, we will toggle PA5 (TIM2_CH1) based on out setting.
2. Required parts:
We will need the following:
- STM32F767 Nucleo-64
- LED
- Breadboard
- Hookup wires
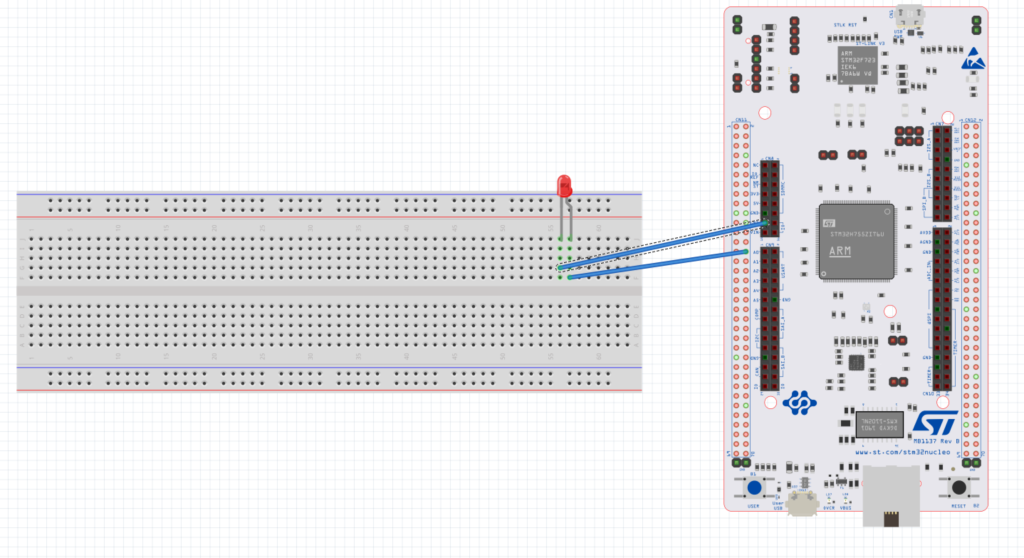
3. Code:
We start of by enabling clock access to GPIOA and set PA0 to alternative function as shown in PWM guide:
#define TIM2_AF 0x01 RCC->AHB1ENR |= RCC_AHB1ENR_GPIOAEN; GPIOA->MODER&=~ GPIO_MODER_MODER0; //reset PA0 and PA1 GPIOA->MODER|= GPIO_MODER_MODER0_1; //set PA0 and PA1 as alternate mode GPIOA->AFR[0] |= (TIM2_AF<<0);
Then enable clock access to timer2 and disable the timer
RCC->APB1ENR |= RCC_APB1ENR_TIM2EN; TIM2->CR1 &=~ TIM_CR1_CEN;
Then we need to set the pin to toggle on match in CCRM1 register:
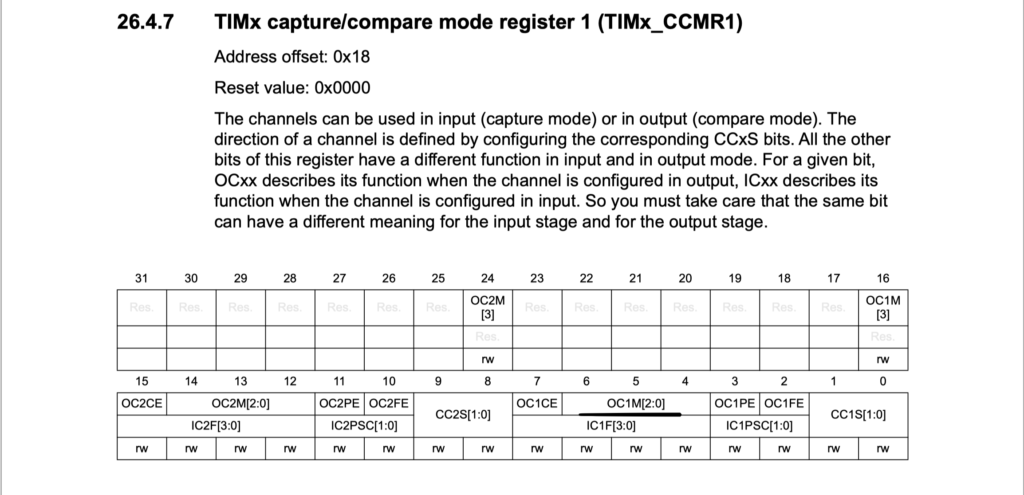
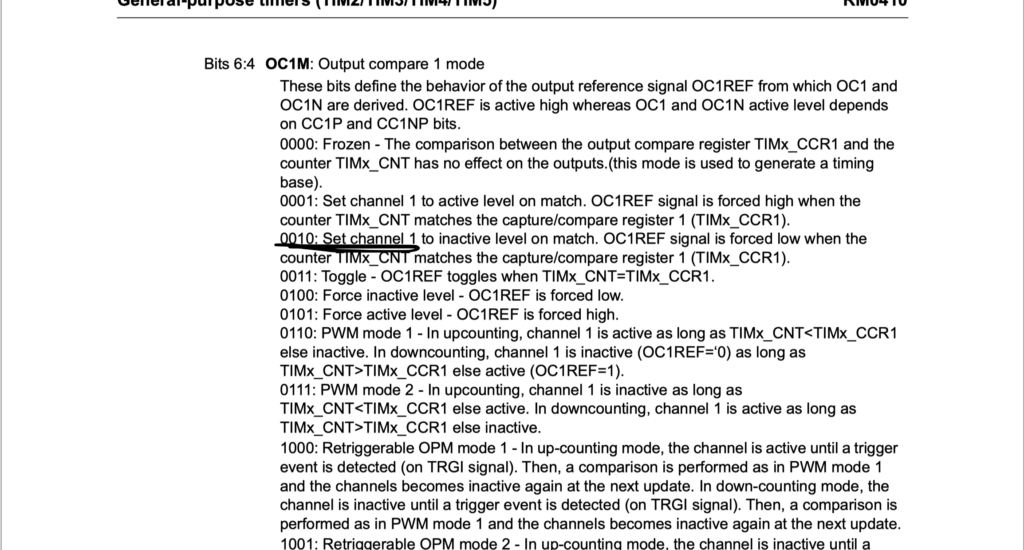
TIM2->CCMR1|=TIM_CCMR1_OC1M_0|TIM_CCMR1_OC1M_1;
Then enable channel1 from capture/compare enable register

TIM2->CCER|=TIM_CCER_CC1E;
Since the MCU is running at 16MHz we can achieve toggle rate of 1Hz by setting the prescaler to 15999 and ARR to 1000 as following:
TIM2->PSC=16000-1; /*16 000 000 / 16 000 =1 000*/ TIM2->ARR=1000-1; /*1 000 / 1 000 = 1HZ*/
Finally enable the timer
TIM2->CR1 |= TIM_CR1_CEN;
4. Demo:
And we are successfully blinked the LED with rate of 1Hz using the hardware level rather than forcing the MCU to wait or generating an interrupt
Happy coding 🙂
Add Comment