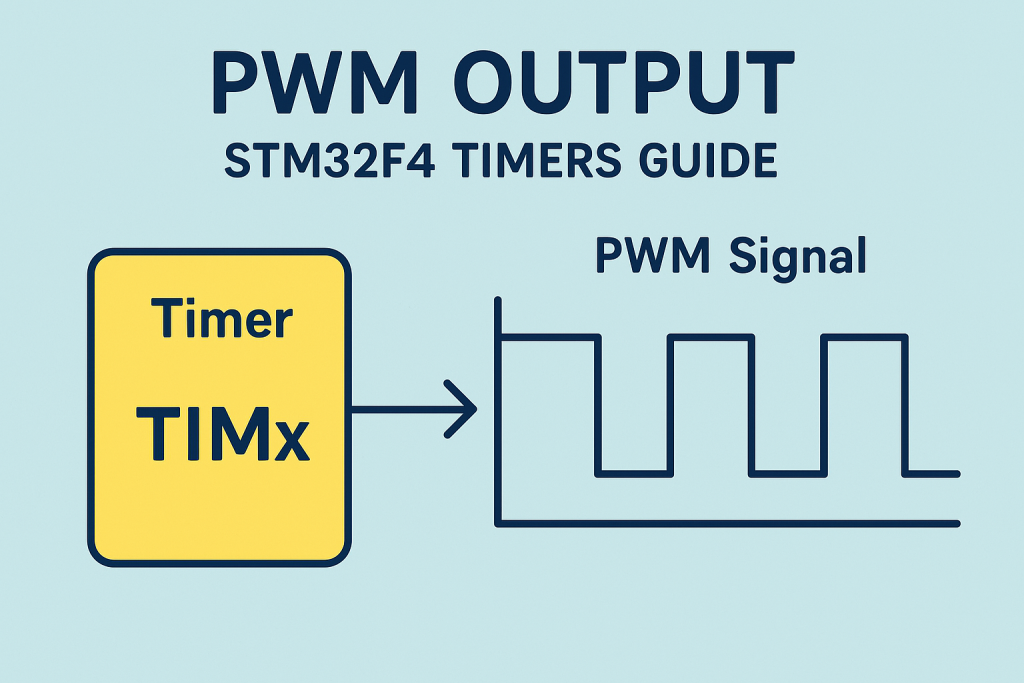
PWM (Pulse-Width Modulation) is a fundamental feature of STM32F4 timers used to generate precise variable-duty cycle signals for controlling devices like motors, LEDs, and buzzers. This guide explains how to configure STM32F4 timers to output PWM signals on GPIO pins.
In this guide, we shall cover the following:
- Introduction.
- STM32CubeIDE setup.
- Firmware Development.
- Results.
1. Introduction:
Introduction to PWM and its Applications
Pulse-Width Modulation (PWM) is one of the most essential techniques in embedded systems and electronics for controlling power, signal levels, or simulating analog behavior using digital hardware. At its core, PWM works by rapidly switching a digital signal between high (ON) and low (OFF) states at a fixed frequency. By adjusting the proportion of time the signal stays high within each period — known as the duty cycle — it is possible to control the average power delivered to a load without the need for complex analog circuitry.
PWM is widely used because of its simplicity, flexibility, and efficiency. Since most microcontrollers, including STM32F4 series devices, operate in a digital domain (on or off), PWM provides an elegant way to interface with devices that require varying levels of power or control.
Why Use PWM?
PWM is used in a broad range of real-world applications across different industries:
- Motor ControlPWM controls the speed and torque of DC motors or position of servos by varying the duty cycle of the driving signal. It’s commonly used in robotics, automotive systems, drones, and industrial automation.
- LED Brightness ControlLEDs respond well to PWM control since human eyes naturally average out the flickering light. By changing the duty cycle, you can create smooth dimming effects without changing the LED’s operating current.
- Audio Signal GenerationPWM can generate sound signals or simple audio tones for buzzers or speakers by varying the frequency of the pulses.
- Power RegulationPWM drives switching power supplies (like buck or boost converters) for efficient voltage regulation.
- Communication SystemsCertain communication protocols or infrared transmitters also use PWM-like techniques to encode data.
STM32 Timers for PWM Generation
STM32F4 microcontrollers come equipped with advanced timer peripherals that support various PWM modes. These hardware timers can operate autonomously, generating highly accurate PWM signals with very little CPU overhead. The timers can be configured to output PWM signals on specific GPIO pins, enabling the microcontroller to control external devices directly.
Each timer in the STM32F4 family is highly configurable — supporting different counting modes, prescalers, auto-reload values, and output compare features. This allows developers to fine-tune the frequency and resolution of the PWM signal to match application requirements.
Why Learn PWM on STM32F4?
Understanding how to configure and use PWM output on STM32F4 microcontrollers is a fundamental skill for embedded developers. Whether you’re building a motor driver, an LED lighting system, or a power supply controller, mastering PWM will allow you to unlock the full potential of the STM32 hardware.
This guide will take you step-by-step through the process of setting up PWM output using STM32F4 timers. It will cover the key concepts, timer configuration registers, GPIO setup, and practical examples — enabling you to confidently generate PWM signals for your embedded projects.
WM Generation — Block Diagram and Theory (STM32F4 Timers)
Before jumping into code and configuration, it’s important to understand how the STM32F4 timers work internally to generate PWM signals. STM32 timers are flexible and powerful hardware peripherals designed to handle time-based operations like counting, delays, and signal generation — including PWM.
Basic PWM Generation Principle
At a high level, a timer generates PWM using the following method:
- The timer counts up from zero to a configured maximum value (Auto-Reload Register, ARR).
- The counting happens at a frequency derived from the main system clock, adjusted by a prescaler.
- A Compare Value (stored in the Capture/Compare Register, CCR) determines when the output signal switches from high to low (or low to high), effectively controlling the duty cycle.
- The timer continuously repeats this process, creating a stable PWM waveform on the output pin.
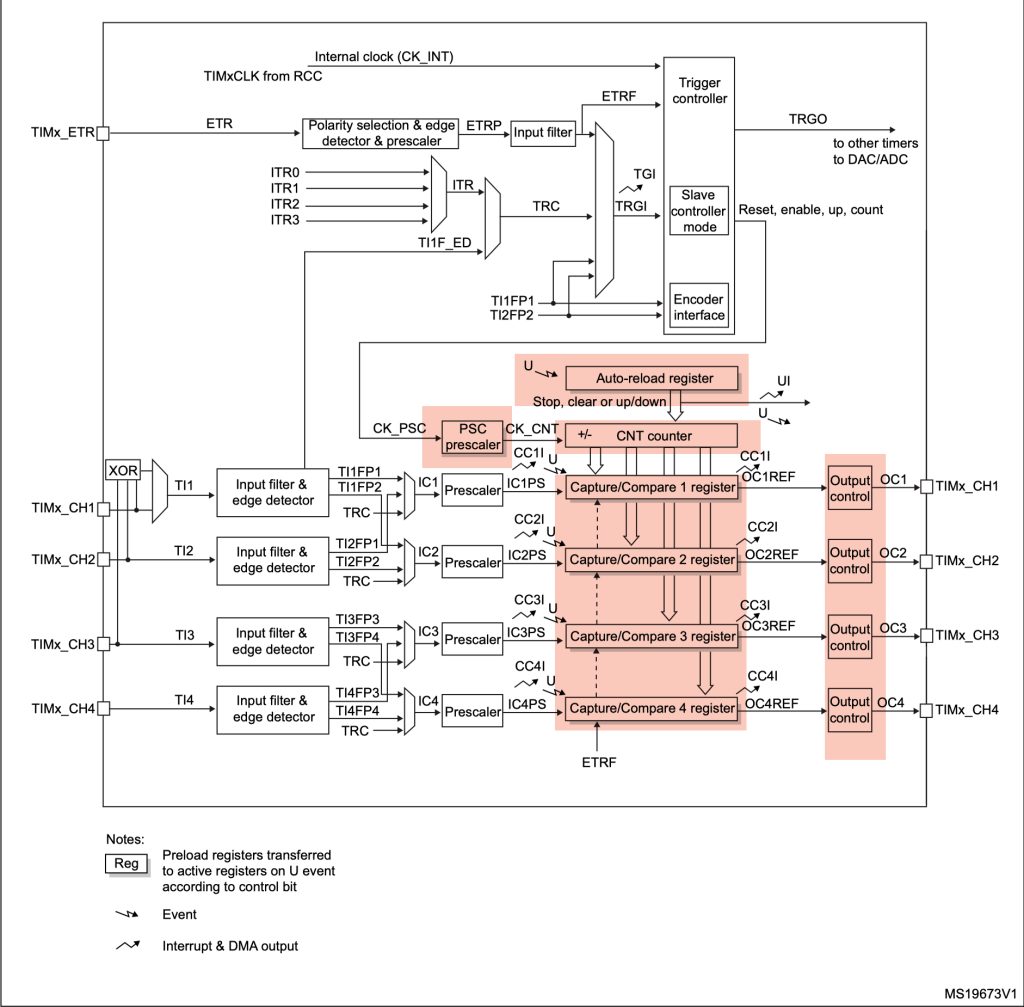
2. STM32CubeIDE Setup:
pen STM32CubeIDE after selecting the workspace and create new project as following:
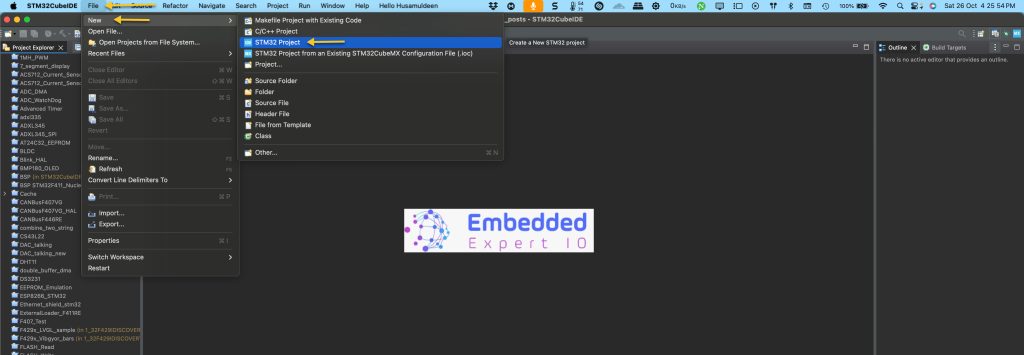
Select the MCU:
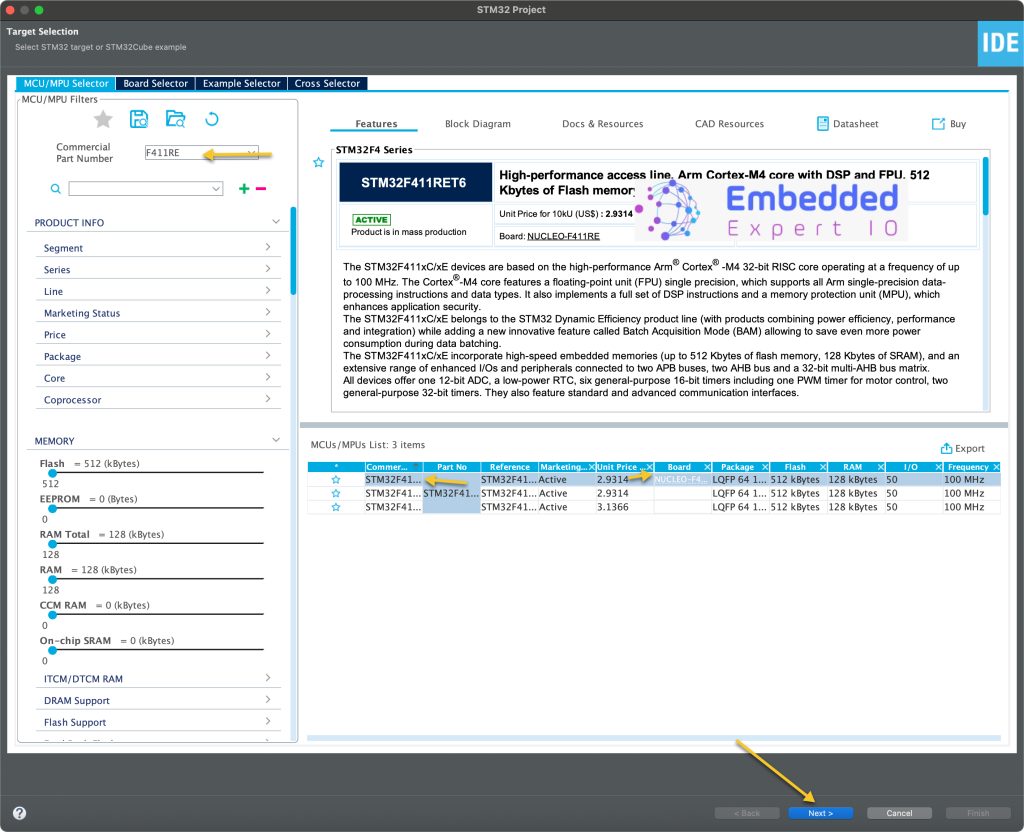
Give the project a name:
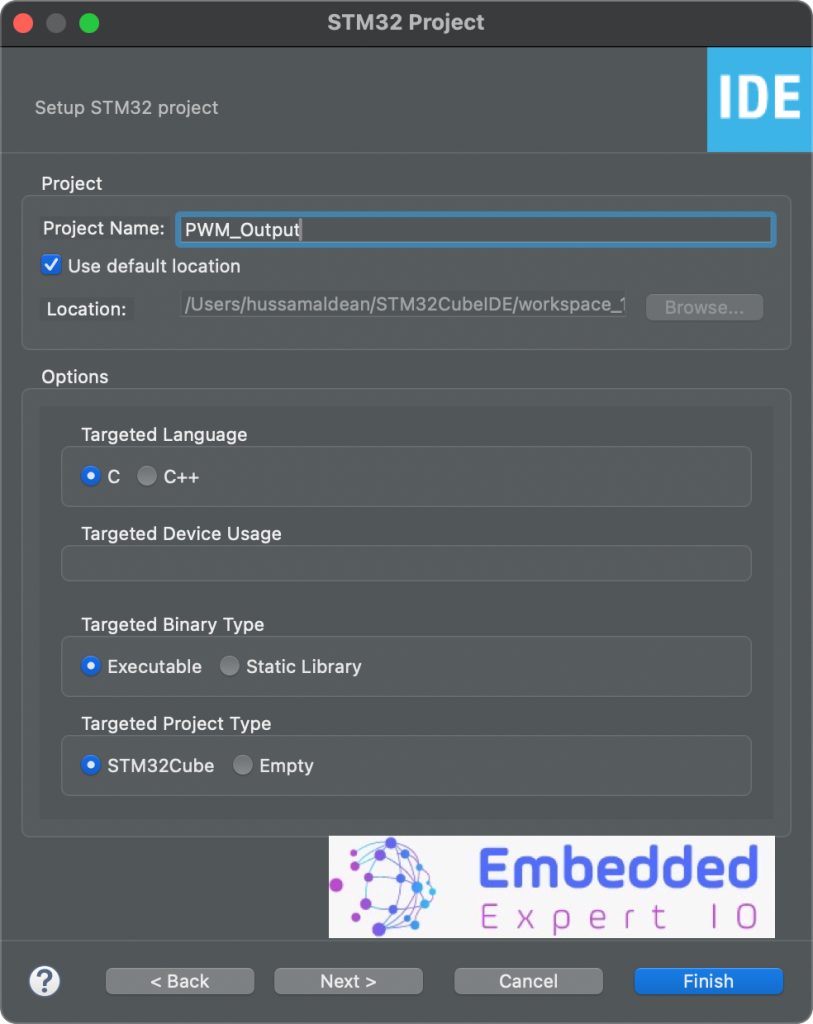
Next, STM32CubeMX Window will appear.
From the window, select Clock Configuration as following:
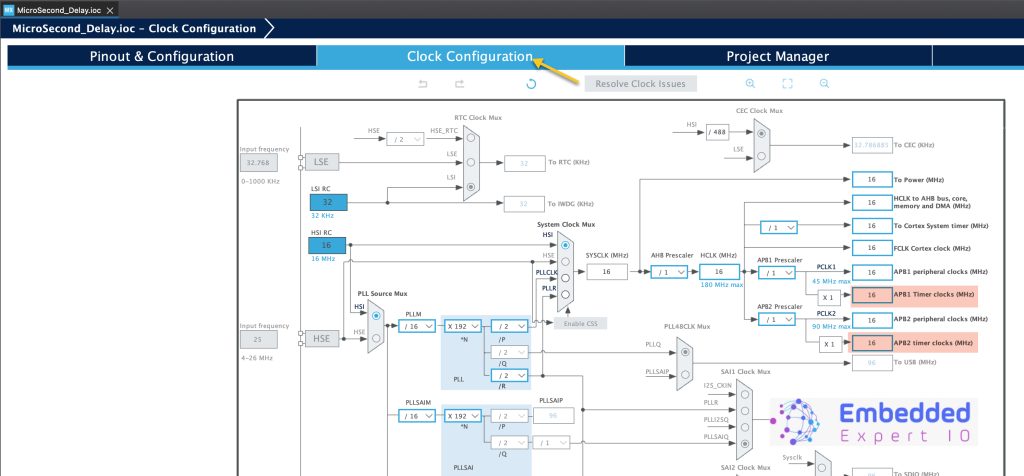
Notice timers clock. Since STM32F4 has default frequency of 16MHz, hence timers frequency is 16MHz.
Next, from Pinout and Configuration, enable TIM2 and configure it as following:
- Clock Source to Internal Clock
- Channel 1 to PWM Generation CH1.
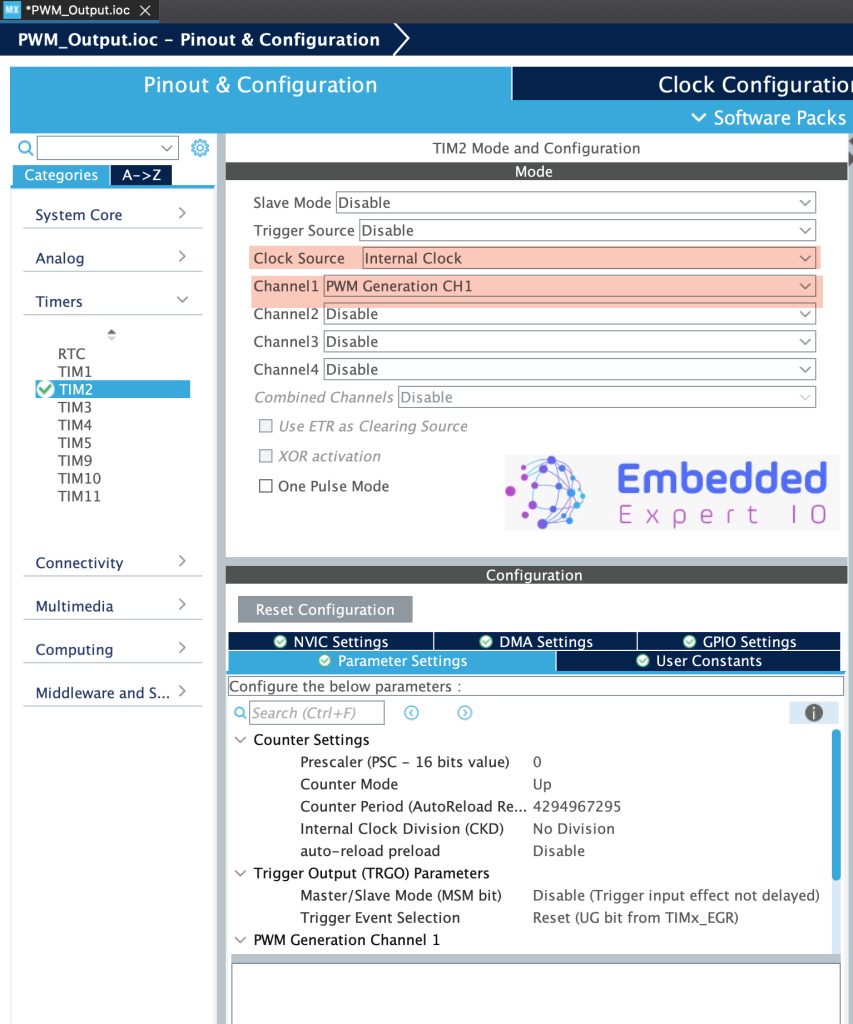
This will activate PA0 as PWM pin.
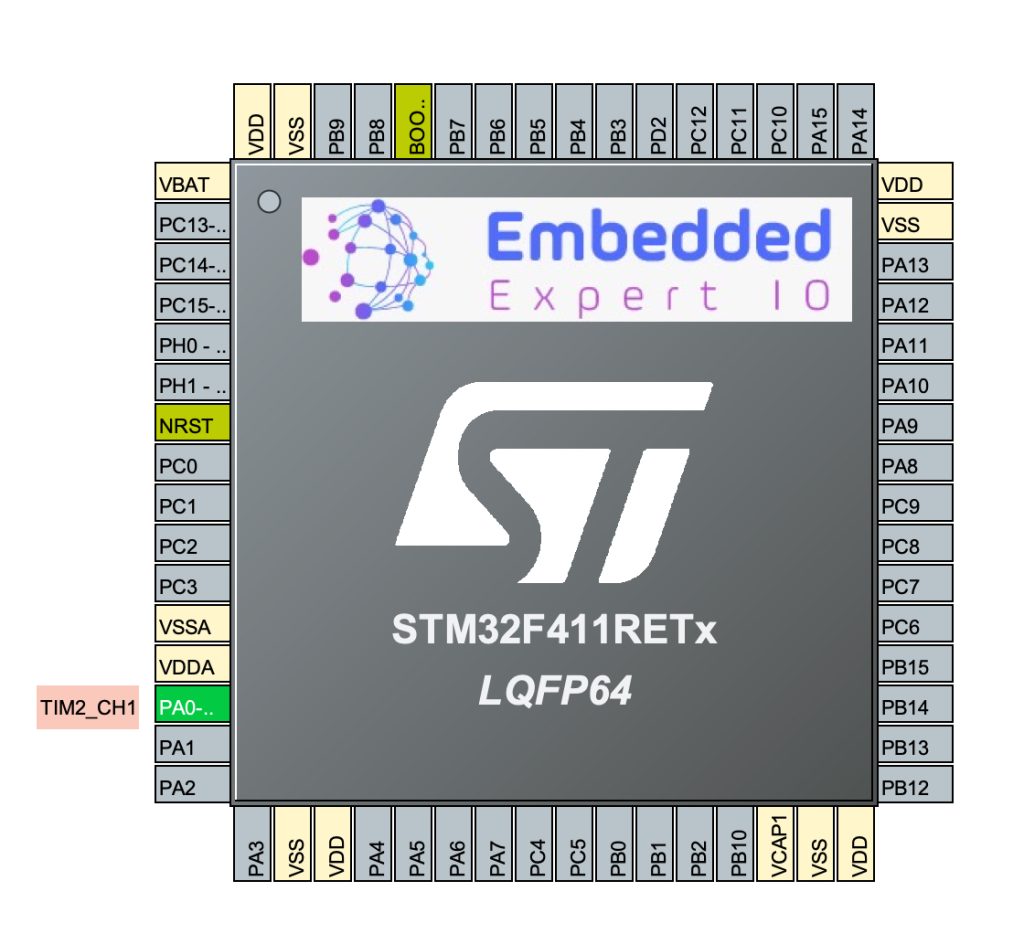
Next, we shall configure the PSC and ARR (Counter Period).
Since we already know that timer frequency is 16MHz, we can use prescaler to 16 to get 1MHz as following:
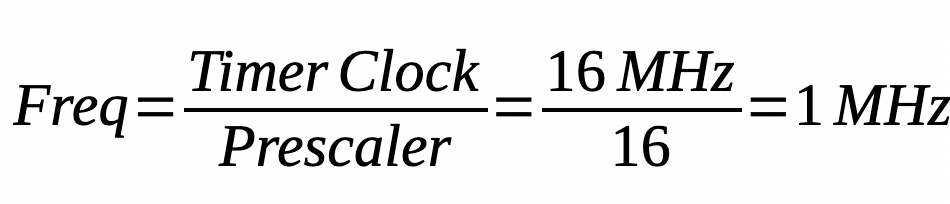
With Counter Period of 100, this will further reduce the speed from 1MHz to 10KHz as following:
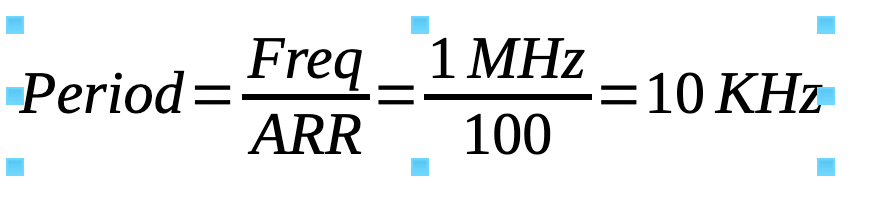
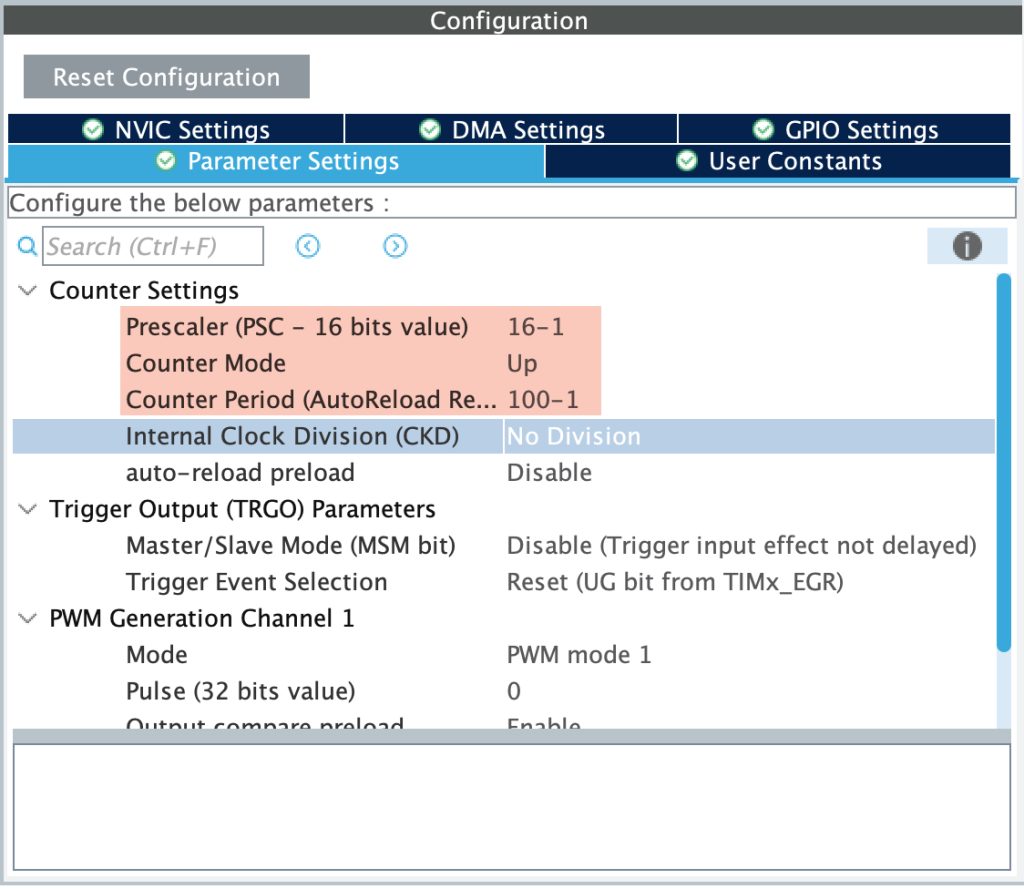
The reason behind the -1 since MCU start counts from 0 rather than 1, hence it needs to be included.
Thats all for the configuration. Save the project and this will generate the project.
3. Firmware Development:
After the code has been generated, in main.c we shall start the timer in PWM mode.
In user code begin 2 of the main function, start the timer in PWM mode as following:
HAL_TIM_PWM_Start(&htim2, TIM_CHANNEL_1);
The function takes the following parameters:
- Instant to the timer, htim2 in this case.
- Timer channel which is channel1 in this case.
Next, we shall configure the duty cycle.
HAL offers API to update the duty cycle of the PWM as following:
__HAL_TIM_SET_COMPARE(&htim2, TIM_CHANNEL_1, 50);
The macro takes the following:
- Instant to the timer (htim2).
- Channel (channel1).
- New value (50).
This will set timer2 channel1 to generate PWM signal with frequency of 10KHz and duty cycle of 50%.
Save the project, build and run it on your board as following:

4. Results:
Using Oscilloscope, we can see the following:
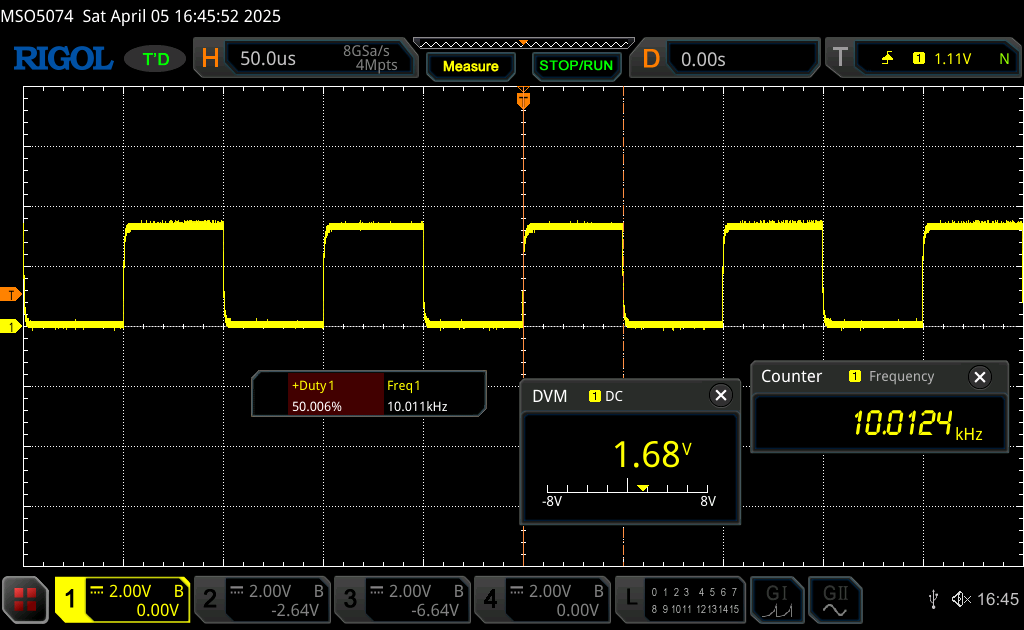
- +duty which is 50% as set in the code.
- Frequency of 10KHz as expected according to our setup.
- Vdc shows as 1.68 which is 3.3V/2 which is the half of the maximum voltage of the IO which is 3.3V.
Now, you are able to drive PWM related hardware with ease.
Happy coding 😉
Add Comment