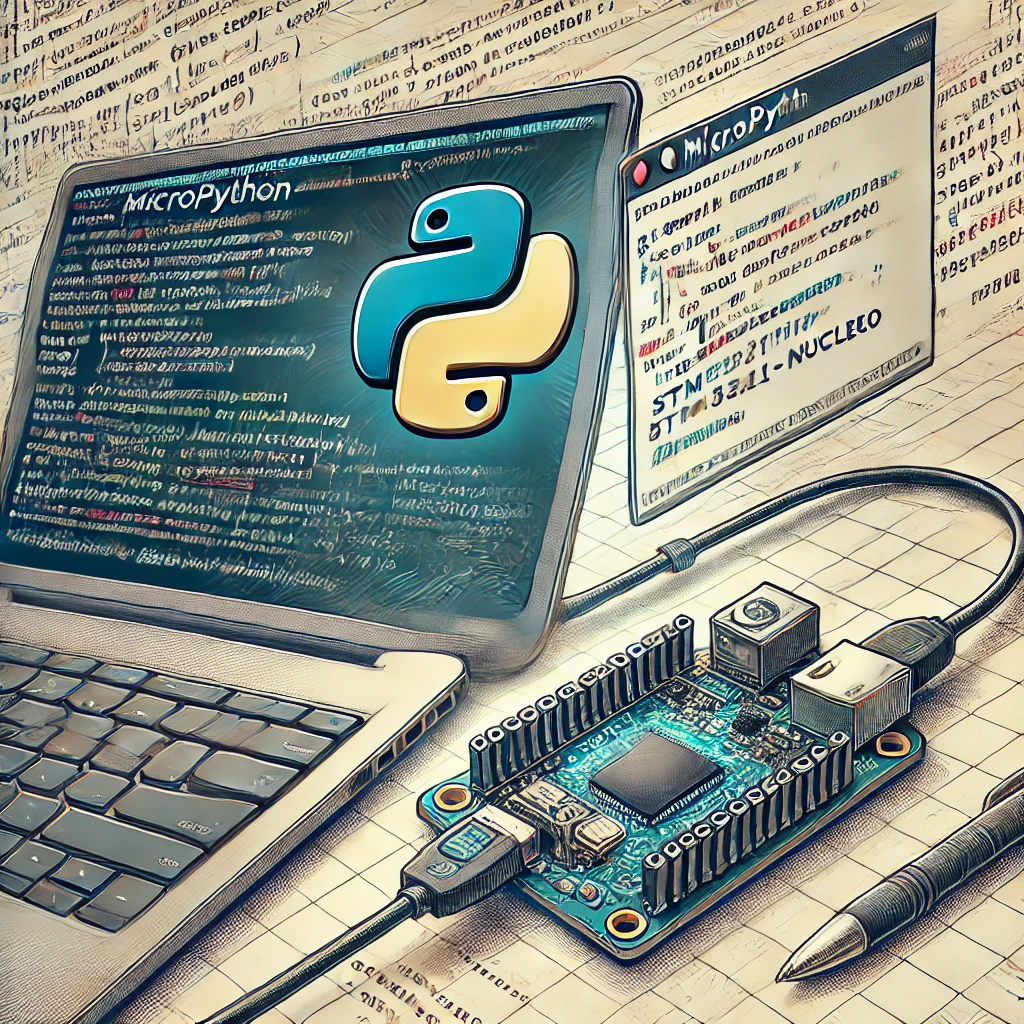
In this guide, we shall see how configure a GPIO as input and read the GPIO pin state and toggle the LED with each press from the push button.
In this guide, we shall cover the following:
- Gathering information.
- Developing the firmware.
- Results.
1. Gathering the Information:
From the user manual of STM32 of Nucleo-64 board, we can find the Push-buttons section, we can find the following:

From the statement, we can see that the user push button (B1) is connected to PC13. Hence, we can configure it as input.
From the schematic of the board:
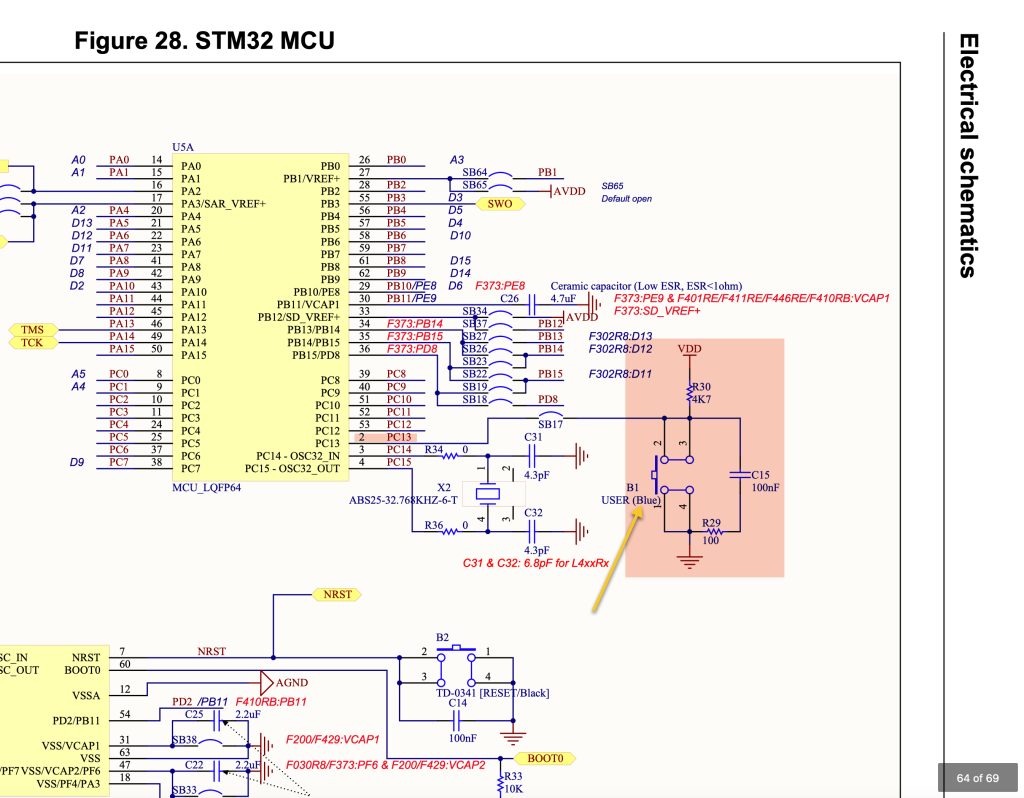
We can find that push button has external push button, hence no need to enable the internal push button.
Thats all for this section.
2. Firmware Development:
Start a new thonny project and configure the interpreter. For more details how to configure it, refer to this guide here.
After the configuration in area where you can write the script we start by import pin from machine as following:
from machine import Pin
Also, import the time as following:
import time
Next, declare a button as following:
In case you want to use the internal pull up:
# Configure the push button (PC13) as input with pull-up button = Pin("PC13", Pin.IN, Pin.PULL_UP)
In case you want to use external one:
# Configure the push button (PC13) as input (no internal pull-up) button = Pin("PC13", Pin.IN)
In both cases, when using either internal or external pullup resistor, when the push button is pressed, the pin will have low state.
Declare the LED as output:
# Configure the onboard LED (PA5) as output led = Pin("PA5", Pin.OUT)
Declare a variable to hold the LED state:
# Store the LED state led_state = False
This will allow us to change the state off the LED connected to PA5.
Declare a while loop as following:
while True: if button.value() == 0: # Button is pressed (PC13 is active low) led_state = not led_state # Toggle LED state led.value(led_state) time.sleep(0.3) # Debounce delay to prevent multiple toggles
First, check if the button state is low as following:
if button.value() == 0: # Button is pressed (PC13 is active low)
If yes, flip the state of the LED state.
Write the new state to the led pin.
Wait for 0.3 second for debouncing.
Click on run as following:
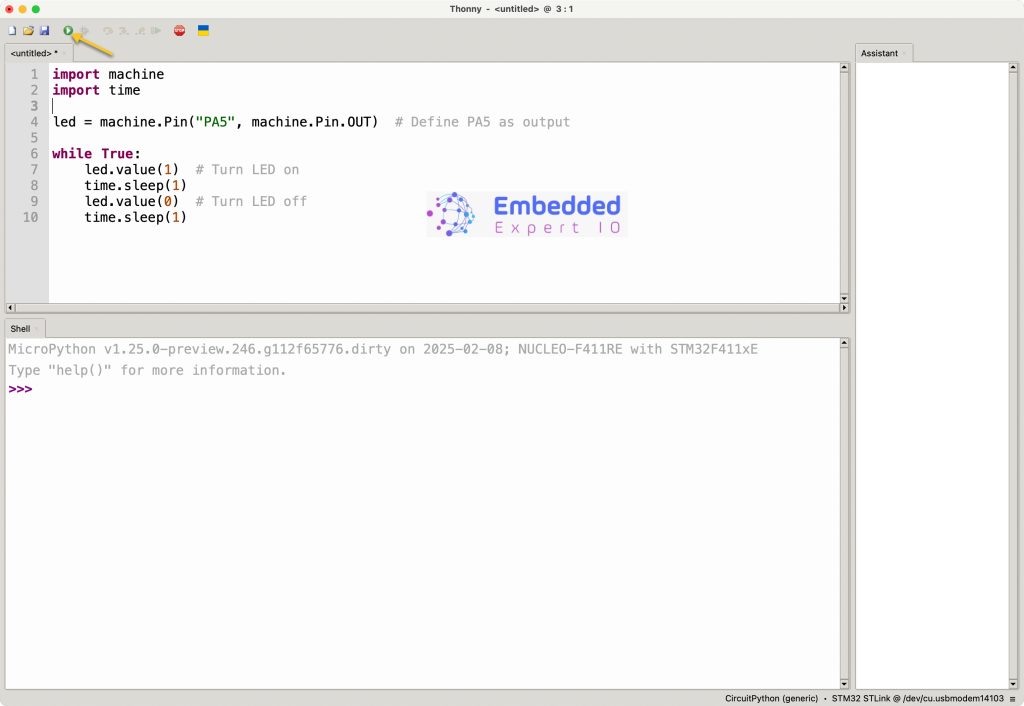
3. Results:
Happy coding 😉
Add Comment