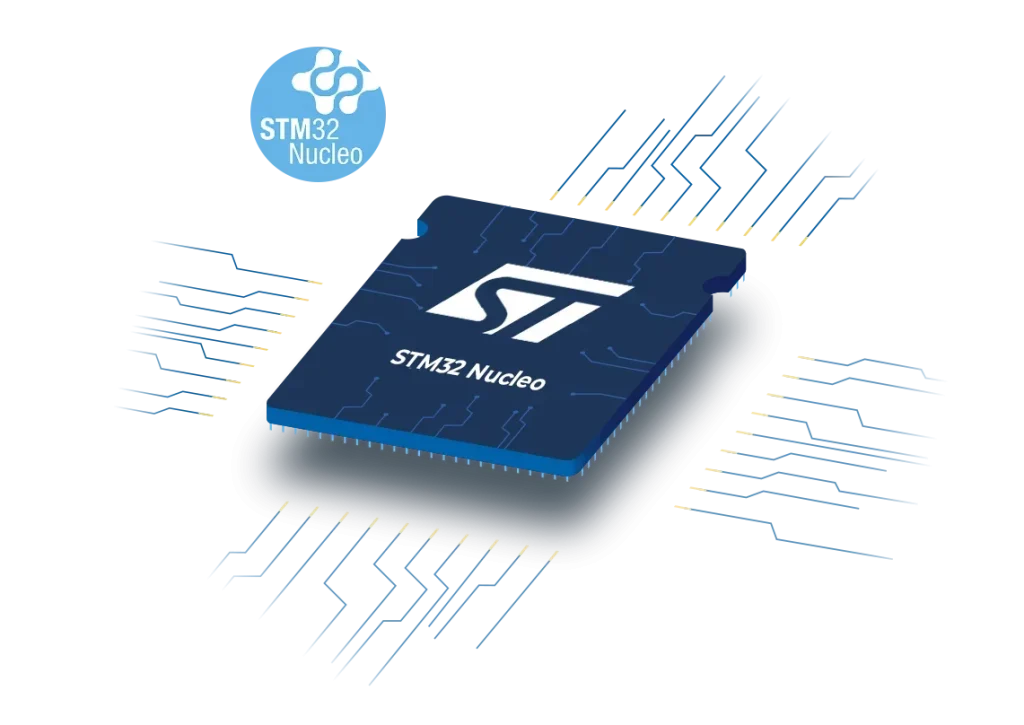
In this guide, we shall use DMA to offload the CPU from acquiring the data from ADC and improve the performance of the MCU.
In this guide, we shall cover the following:
- STM32CubeMX Modification.
- Firmware development.
- Results.
1. STM32CubeMX Modification:
Open adc_single.ioc file as following:
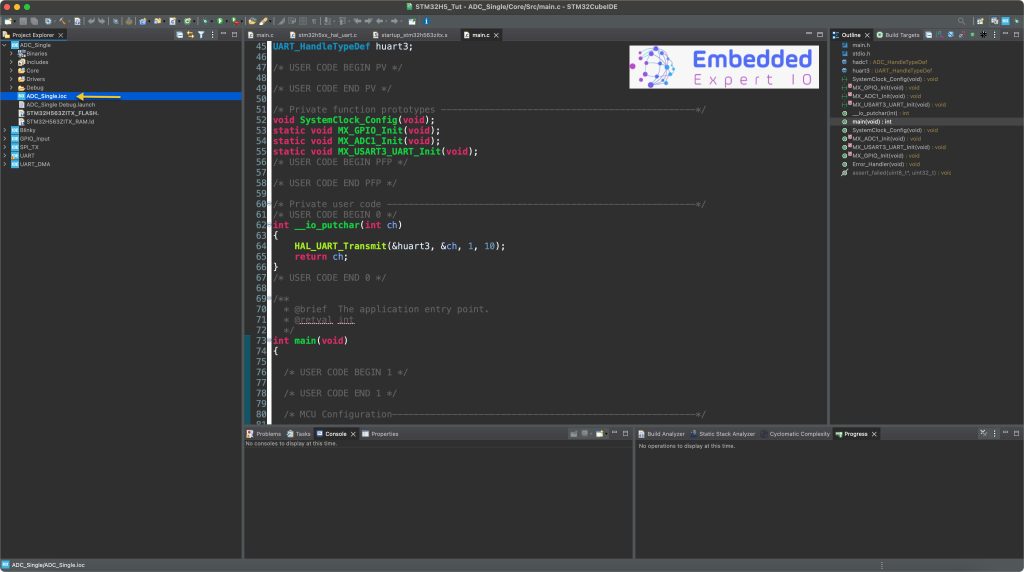
Next, from ADC section, configure the ADC with the following configuration:
- Set the prescaler to be divided by 1 (Doesn’t matter).
- Make sure the continuous mode is enabled.
- Make sure to enable DMA Continuous Request.
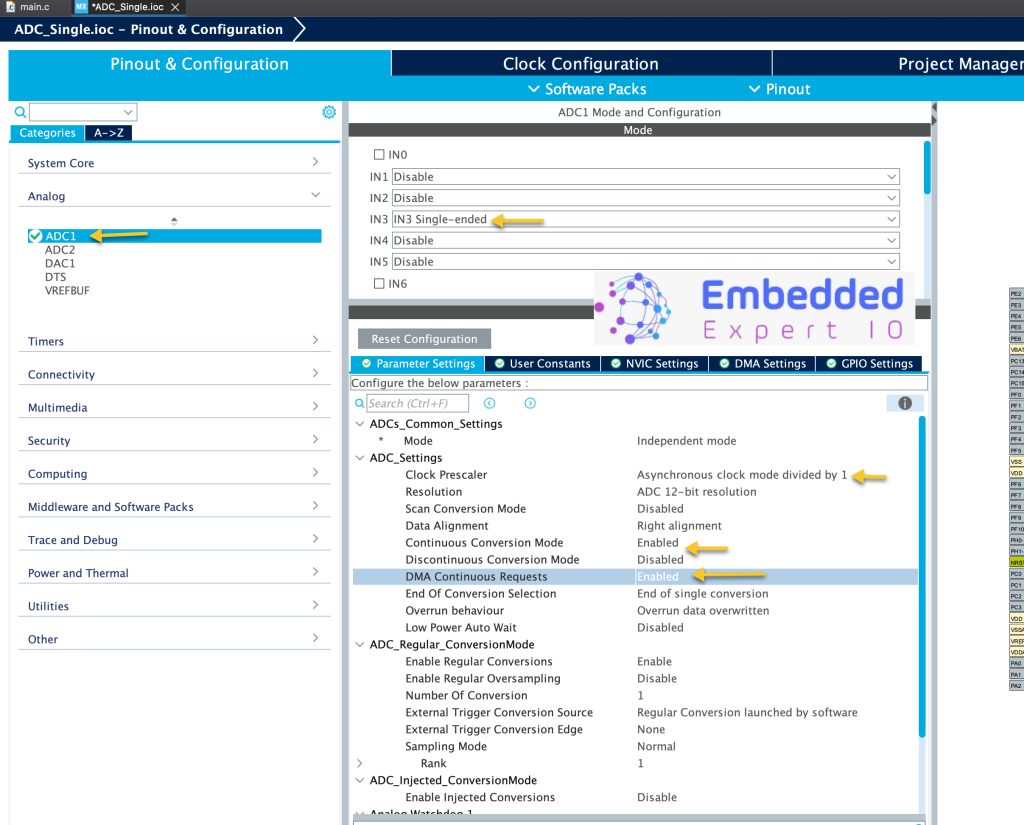
Next from System Core, click either on GPDMA1 or GPDMA2 then enable any channel as following (This guide shall use Channel0 of GPDMA1).
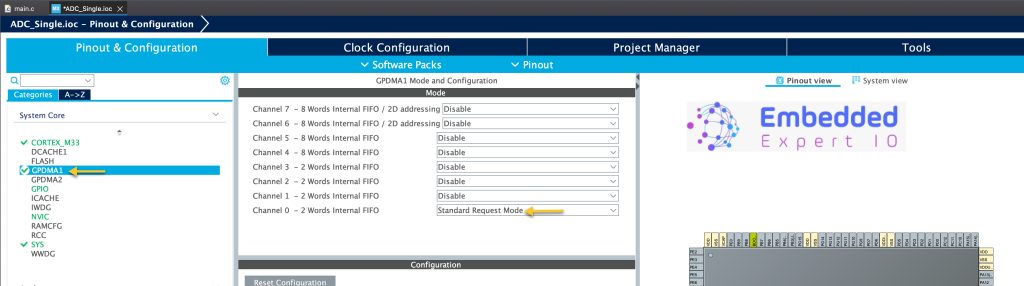
Then select the enabled channel and configured it as following:
- Enable Circular mode.
- Set the request to be ADC1.
- Direction must be Peripheral to Memory.
- Set the data width of source and destination to be half world since the ADC is 12-bit.
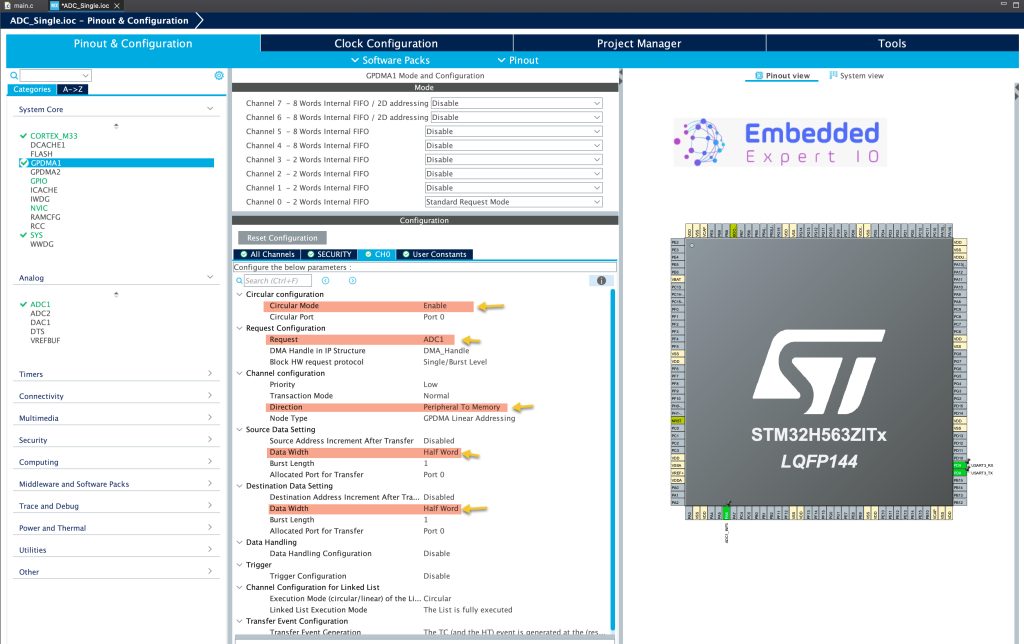
Thats all for the STM32CubeMX configuration. Save the project and this will generate the code.
2. Firmware Development:
After the code is generated, in main.c file, we shall declare the following variable to store the ADC value as following:
uint16_t adc_value;
uint16_t is 16-bit variable since the ADC is 12-bit.
Next, in user code begin 2 in main function, start the ADC in DMA mode as following:
HAL_ADC_Start_DMA(&hadc1, &adc_value, 1);
The function requires three parameters:
- Pointer to the ADC, which is ADC1.
- Address of the variable to store the ADC size.
- Size of the data to be collected, 1 in this case.
Note: If you want to store 10 values of the ADC, change adc_value variable to array as following:
#define adc_data_size 10 uint16_t adc_value[adc_data_size]={};
In launching ADC in DMA mode:
HAL_ADC_Start_DMA(&hadc1, &adc_value, adc_data_size);
In user code begin 3 in while loop, you could print the adc values each half second as following:
printf("ADC Value =%d\r\n",adc_value); HAL_Delay(500);
Save the project, build it and run it on you STM32H563Zi Nucleo-144 board as following:

3. Results:
Open your serial terminal application, set the baud rate 115200 and you should get the following:
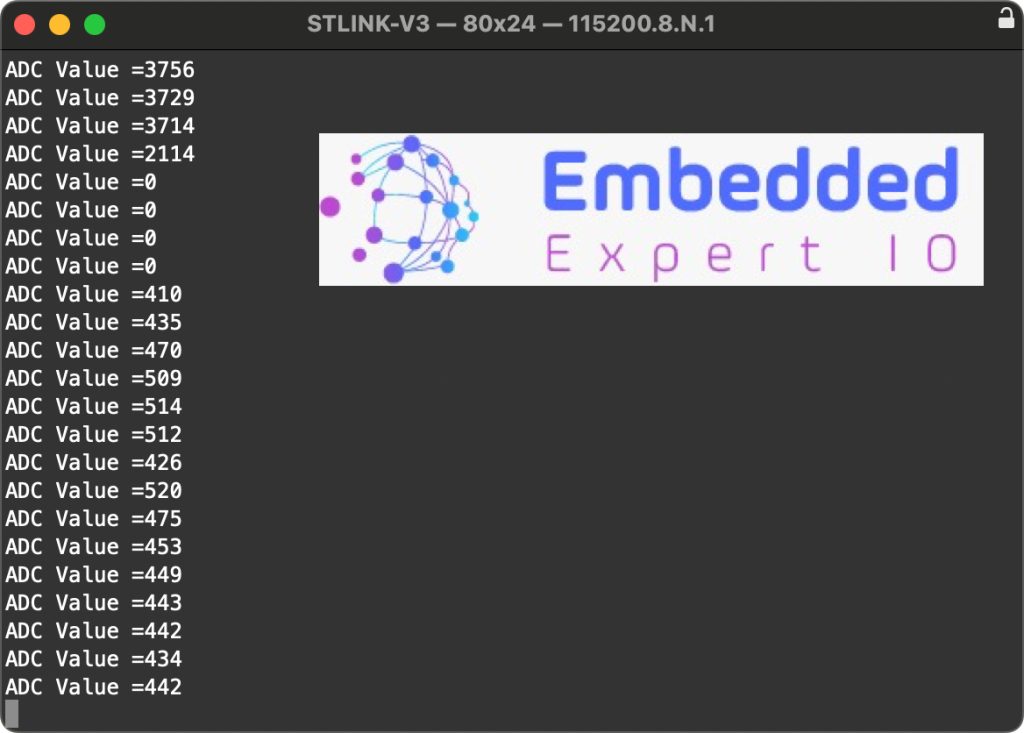
Happy coding 😉
Add Comment