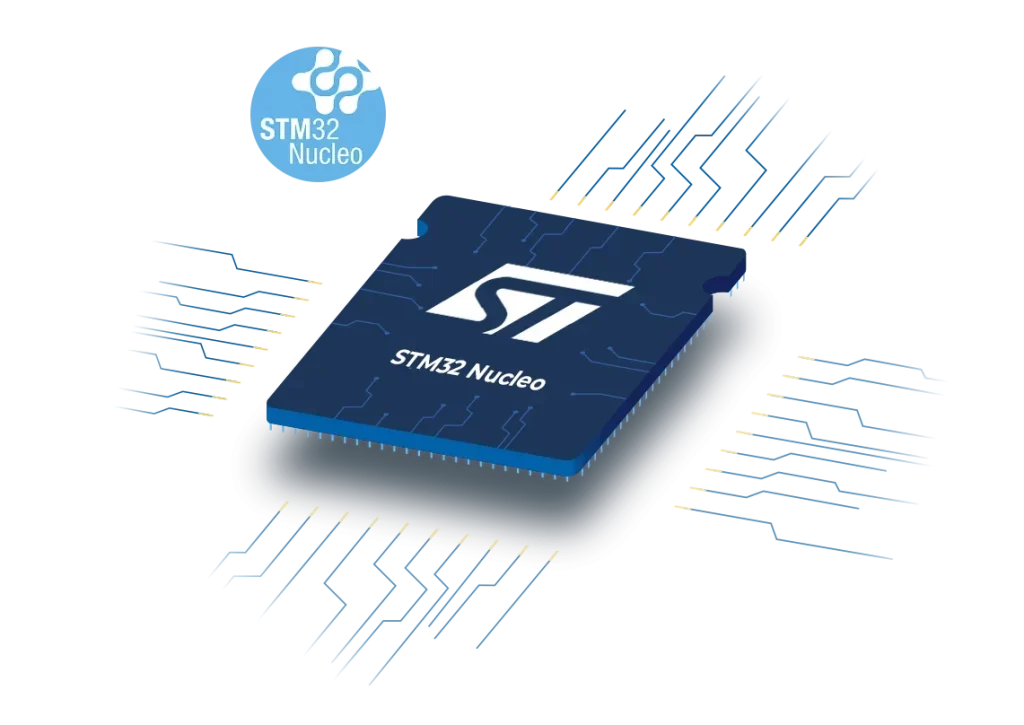
In this guide, we shall use the interrupt of the ADC to acquire the ADC data without waiting for the conversion to be completed.
In this guide, we shall cover the following:
- Modification to STM32CubeMX configuration.
- Modification to the source code.
- Results.
1. Modification to STM32CubeMX:
Open adc_single.ioc file as following:
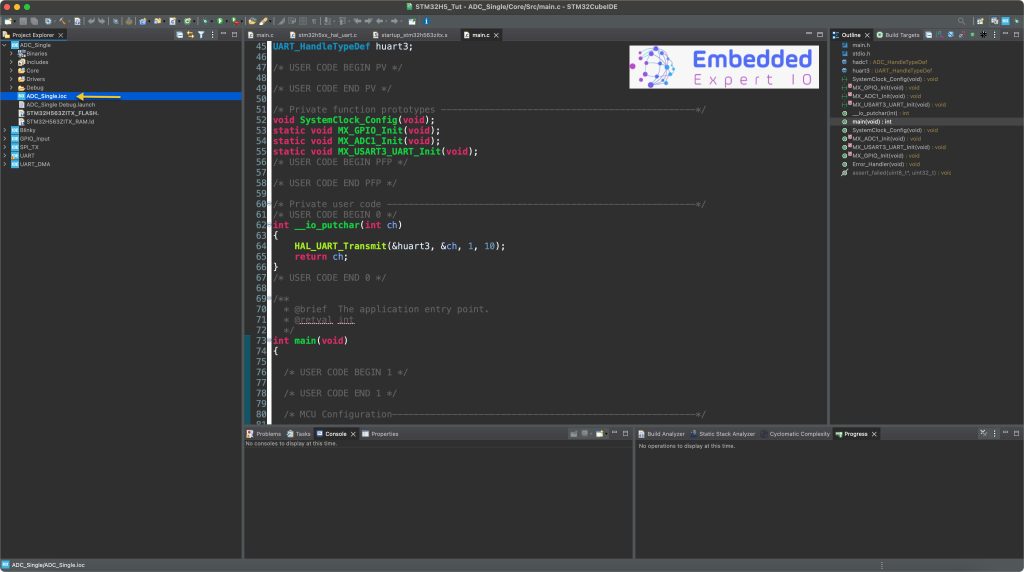
Next, in ADC parameter configuration, set the clock divider to be 16. This will reduce the ADC clock and slow down the interrupt generation significantly. Hence, the CPU shall no spend too much time processing the ADC data.
Set the ADC clock divider as following:
Set the prescaller to Asynchronous clock mode divided by 16.
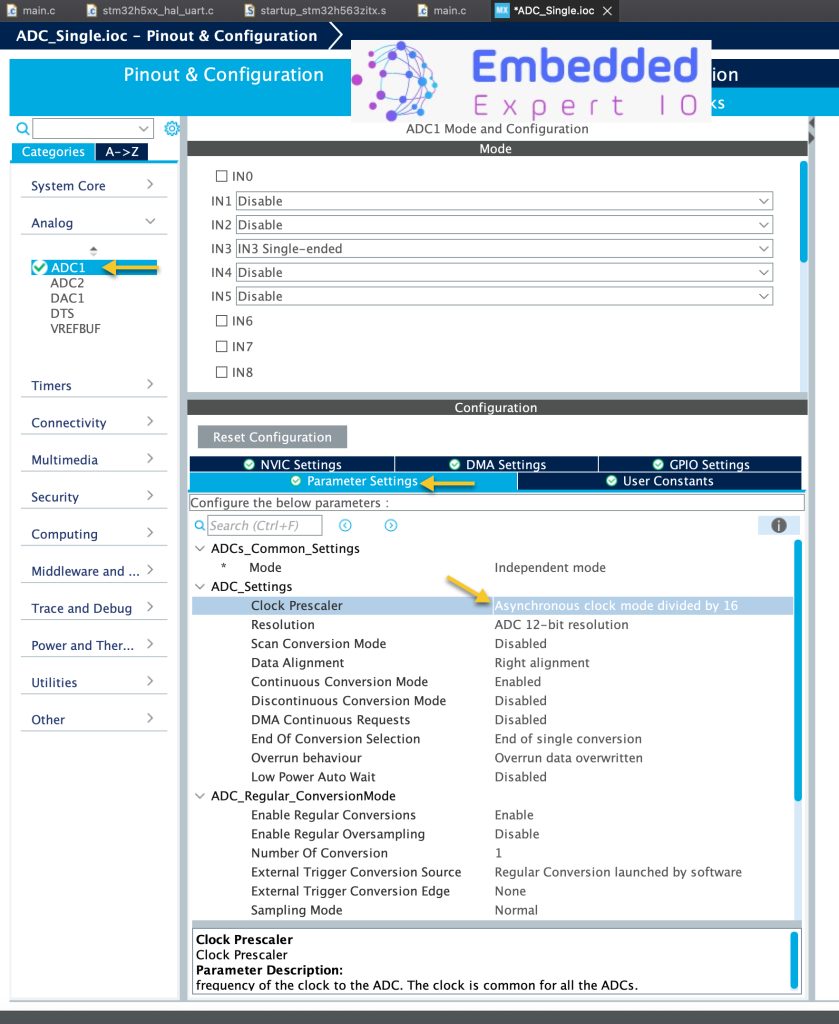
Next, from NVIC settings, enable the ADC interrupt as following (make sure it is marked):
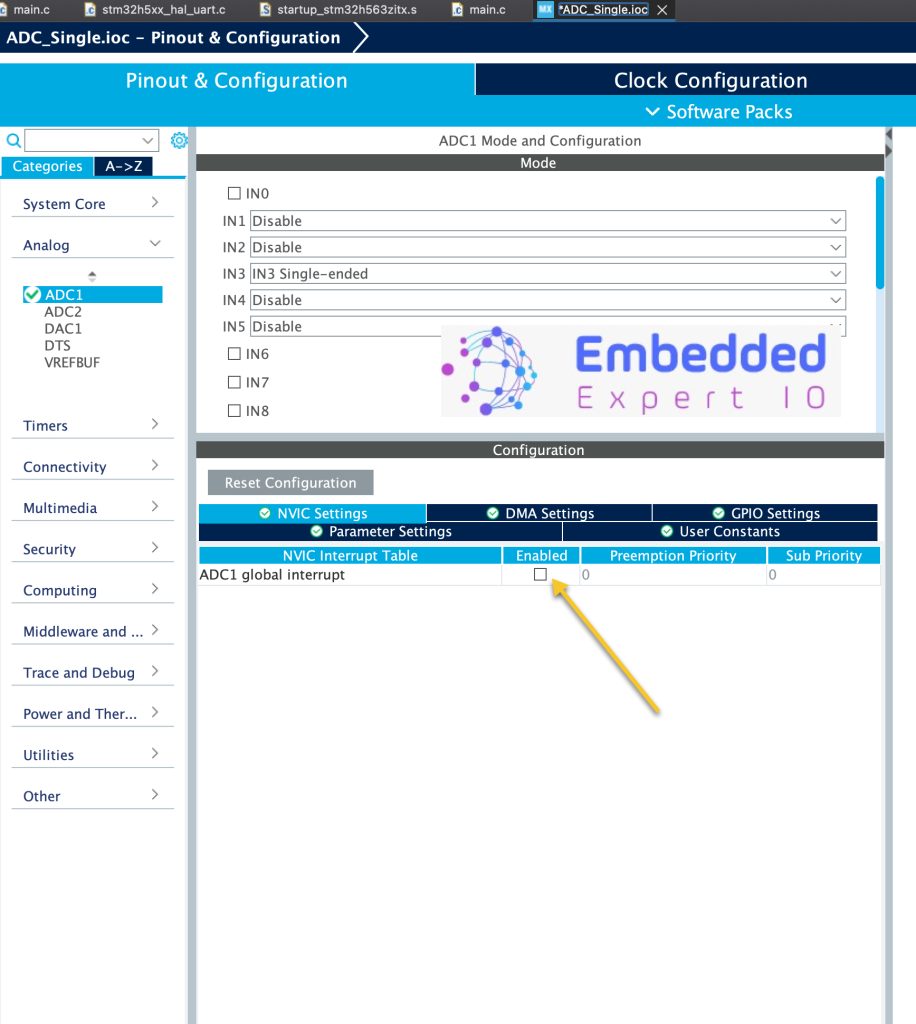
Thats all for the configuration. Save the project and that will generate the new configuration.
2. Modification to the Source Code:
In user code begin PV part of the main declare the following variable:
uint16_t adc_value;
The variable to store the ADC shall be global since it will be modified outside the main loop.
Next, in the user code begin 2 of the main function, start the ADC in the interrupt mode as following:
HAL_ADC_Start_IT(&hadc1);
In the while 1 loop:
We shall simply print the value and delay by 500ms between each printed data as following:
printf("ADC Value =%d\r\n",adc_value); HAL_Delay(500);
As you can see, no need to poll for end of conversion like in the previous guide since the ADC will generate an interrupt each time the ADC has finished the conversion.
In user code begin 4 (in the bottom of the main.c file), we shall handle the ADC interrupt.
In STM32H5xx_hal_adc.c source file, there is a function that can be called each time the ADC generate interrupt as following:
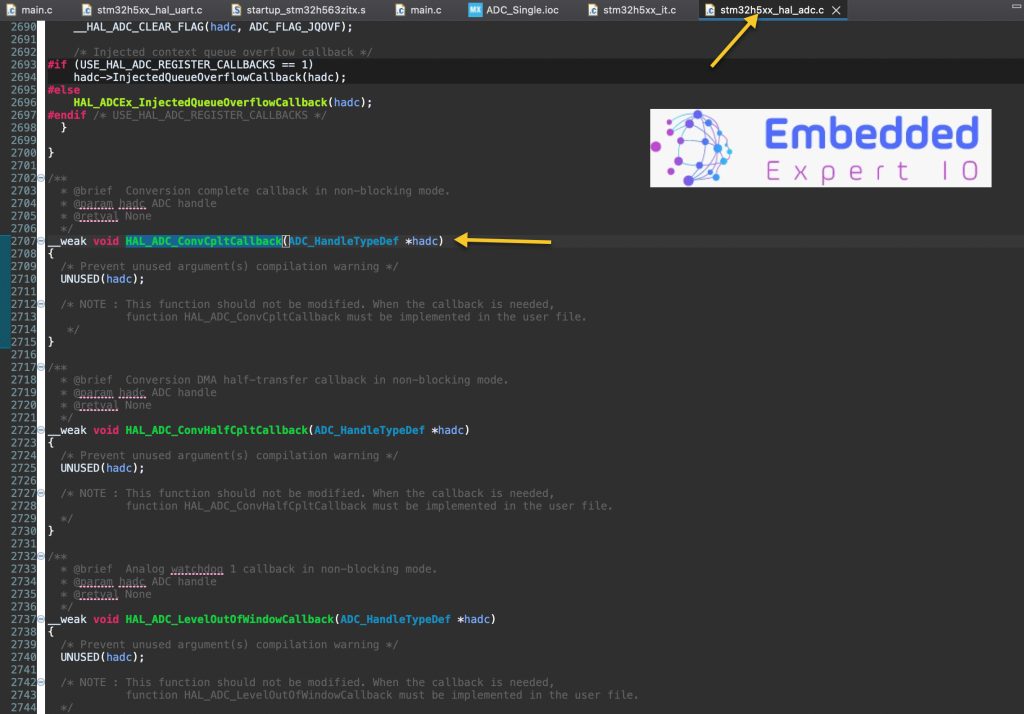
The function called:
void HAL_ADC_ConvCpltCallback(ADC_HandleTypeDef *hadc)
Since it is defined as weak function, the user shall implement it in his firmware.
Hence, in user code begin 4 of the main.c file:
void HAL_ADC_ConvCpltCallback(ADC_HandleTypeDef *hadc) { adc_value=HAL_ADC_GetValue(&hadc1); }
The function shall store the ADC value in the adc_value variable which has been defined globally.
That all for the guide.
Save, build and run the project on your STM32H563Zi Nucleo-144 as following:

3. Results:
Open your serial terminal application, set the baud rate 115200 and you should get the following:
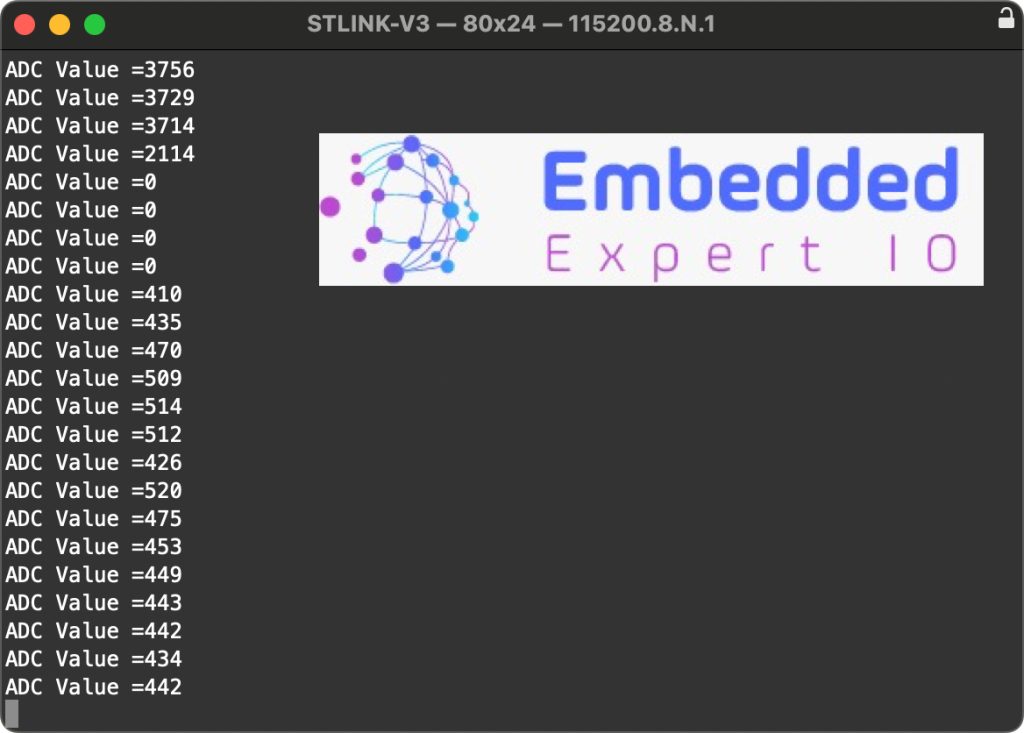
Happy coding 😉
Add Comment