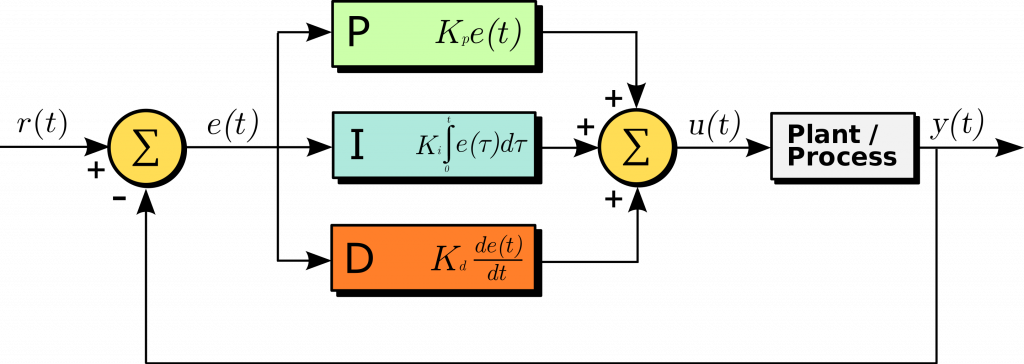
In the previous guide (here), we took a look at how to implement the PID controller programmatically. In this guide, we shall use simple case study to control the speed of a DC motor.
In this guide, we shall cover the following:
- What is speed control.
- Code implementation.
- Demo.
1. What is speed control:
Often we want to control the speed of a DC motor on demand. This intentional change of drive speed is known as speed control of a DC motor.
We can control the speed of DC motor manually or through an automatic control device. This is different to speed regulation – where the speed can regulate against the natural change in speed due to a change in the load on the shaft.
The speed of a DC motor (N) is equal to
N = K (V – IaRa)/ ø Where, K is a constant.
This implies three things:
- Speed of the motor is directly proportional to supply voltage.
- The Speed of the motor is inversely proportional to armature voltage drop.
- The motor speed is inversely proportional to the flux due to the field findings
Thus, the speed of a DC motor can control in three ways:
- By varying the flux, and by varying the current through field winding
- By varying the armature voltage, and the armature resistance
- Through the supply voltage
1. Flux Control Method
Due to the field winding, the magnetic flux varies in order to vary the speed of the motor. As the magnetic flux depends on the current flowing through the field winding, it changes by varying the current through the field winding. This can achieve using a variable resistor in a series with the field winding resistor.
Initially, when the variable resistor keeps at its minimum position, the rated current flows through the field winding due to a rated supply voltage, and as a result, the speed is kept normal. When the resistance increases gradually, the current through the field winding decreases. This in turn decreases the flux produced. Thus, the speed of the motor increases beyond its normal value.
2. Armature Control Method
The controlling of armature resistance controls the voltage drop across the armature. With this method, the speed of the DC motor can control. This method also uses a variable resistor in series with the armature.
When the variable resistor reaches its minimum value, the armature resistance is at normal one. Therefore, the armature voltage drops. When the resistance value gradually increases, the voltage across the armature decreases. This in turn leads to decrease in the speed of the motor. In this way, this method achieves the speed of the motor below its normal range.
3. Voltage Control Method
Both the above mentioned methods cannot provide speed control in the desirable range. Moreover, the flux control method can affect commutation. Whereas the armature control method involves huge power loss due to its usage of resistor in series with the armature. Therefore, a different method is often desirable – the one that controls the supply voltage to control the motor speed.
In such a method, the field winding receives a fixed voltage, and the armature gets a variable voltage. One such technique of voltage control method involves the use of a switch gear mechanism to provide a variable voltage to the armature. Another one uses an AC motor driven Generator to provide variable voltage to the armature (named as Ward-Leonard System).
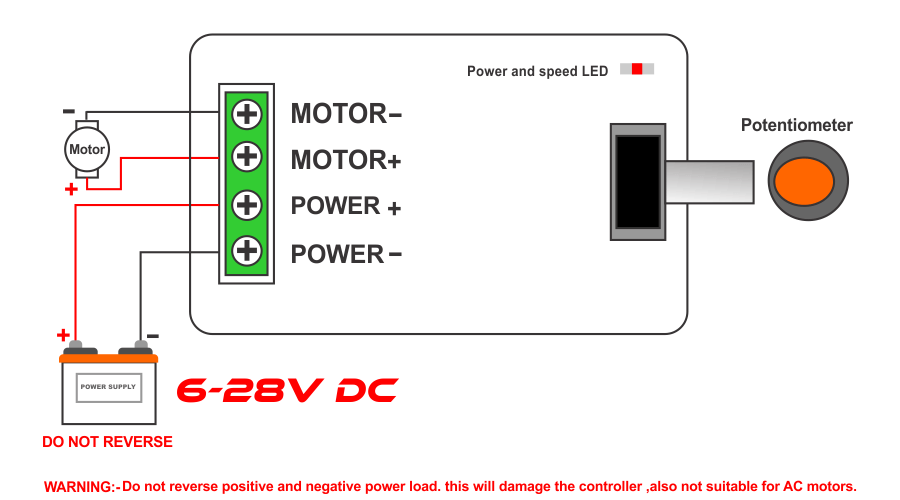
Apart from these two techniques, the most widely used technique is the use of pulse width modulation to achieve speed control of a DC motor. PWM involves application of varying width of pulses to the motor driver to control the voltage applied to the motor. This method proves to be very efficient as the power loss keeps at minimum, and it doesn’t involve the use of any complex equipment.
PWM is achieved by varying the pulses applied to the enable pin of the motor driver IC to control the applied voltage of the motor. The variation of pulses is done by the micro controller, with the input signal from the push buttons.
We hope that we covered all the details and relevant description on DC motor speed control.
2. Code Implementation:
First, include the header file of PID control
#include "pid.h"
Declare the structure of the PID:
PID_Param_t pid_par;
variable to store current rpm and output:
float rpm; uint32_t out;
in the main function:
Set the parameters of PID:
pid_par.Kp=1.4; pid_par.Ki=0.001; pid_par.Kd=1; pid_par.Ts=1; pid_par.Anti_windup=Anti_windup_disabled; pid_par.Anti_windup_error=10; pid_par.Set_point=4000; pid_par.Outmin=100; pid_par.Outmax=1000;
Initialize PID controller:
PID_init(&pid_par);
In the while loop:
rpm=get_rpm(); if(millis()%(uint64_t)pid_par.Ts==0){ out=PID_Calculation(rpm); set_duty_cycle(out); } if(millis()%10==0){ printf("%0.1f\t%0.1f\r\n",(float)pid_par.Set_point,rpm);}
You may download the source code from here:
3. Demo:
Note: the parameters are not tuned at all.
Happy coding 🙂
Add Comment