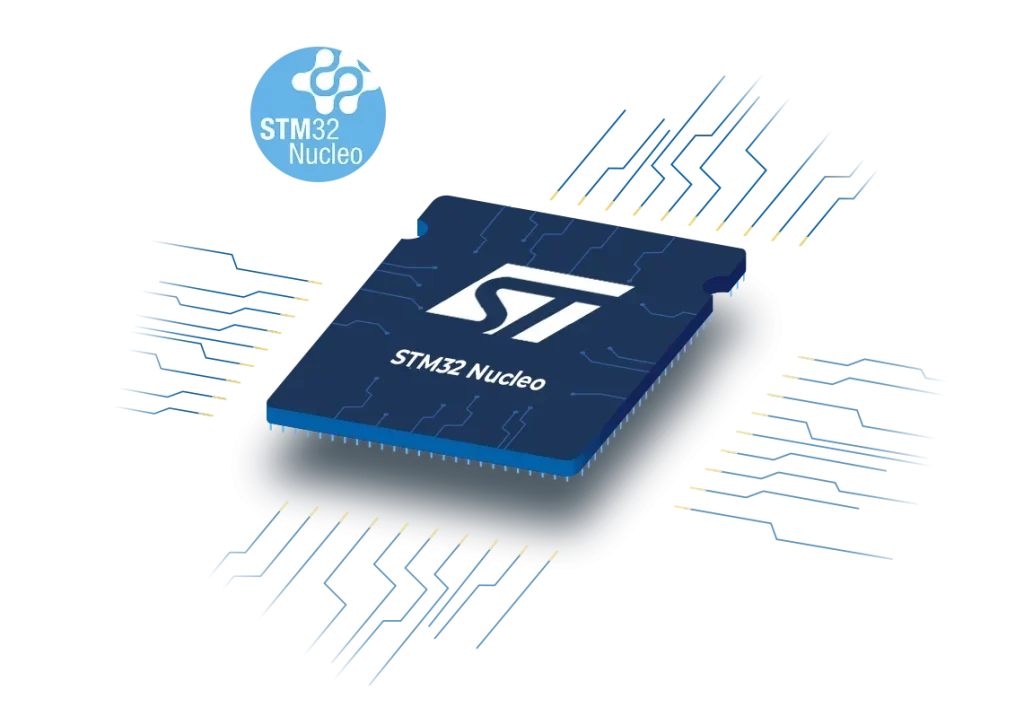
In the second part of timer series on STM32H5, we shall use timer in output compare to blink the LED using hardware level rather than software.
In this guide, we shall cover the following:
- What is Output Compare Mode.
- STM32CubeMX setup.
- Firmware Development.
- Results.
1. What is Output Compare Mode:
The Output Compare concept in STM32 is based on comparing the timer’s counter value with a predefined value (stored in a capture/compare register) to trigger an event or perform an action. It’s used for precise timing, periodic signals, and PWM generation.
Core Concept:
1. Timer Counting:
• A timer counts up, down, or both (depending on the mode).
• The current count value is stored in the Counter Register (CNT).
2. Comparison:
• A predefined value is stored in a Compare Register (CCR).
• The timer continuously compares the CNT value with CCR.
3. Match Event:
• When CNT == CCR, the timer generates a match event.
• The event can trigger an action (e.g., toggling a pin, generating an interrupt, or updating a PWM signal).
Use Cases:
• Generating Periodic Signals: Toggle a GPIO pin at regular intervals.
• PWM Signal Generation: Control duty cycles for motor drivers or LED dimming.
• Input Capture Synchronization: Measure or synchronize external signal timing.
• Event Timing: Trigger specific actions at predefined time intervals.
Output Compare Modes:
1. Toggle Mode: Changes the GPIO pin state (high/low) on each match.
2. Set Mode: Forces the GPIO pin high when a match occurs.
3. Reset Mode: Forces the GPIO pin low when a match occurs.
4. PWM Mode: Outputs a pulse-width-modulated signal, varying the on/off times.
Key Features:
• Resolution: Depends on the timer’s bit width (16-bit or 32-bit).
• Precision: Achieved using the timer’s prescaler to adjust clock speed.
• Flexibility: Multiple channels per timer for simultaneous compare events.
• Interrupts: Can trigger interrupts on compare match for software-driven actions.
Example in Words:
• A timer is configured to count from 0 to 1000 (auto-reload value).
• A compare value of 500 is set in CCR1.
• When the timer reaches 500, it toggles a GPIO pin (or triggers an interrupt).
• The process repeats as the timer resets and counts again.
By using Output Compare, you can control real-time events with high precision, critical in embedded systems like motor control, signal generation, or sensor sampling.
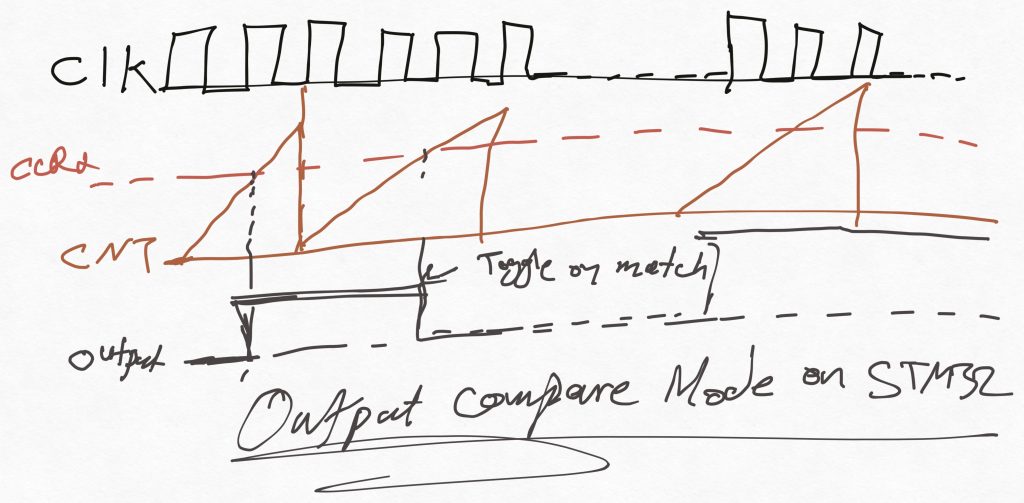
We shall use toggle Mode to blink an LED.
2. STM32CubeMX:
We start off by creating new project with name of OutputCompare. For how to create new project, please refer to this guide.
Since STM32H563Zi Nucleo-144 has arduino pinout, we can use that.
From the user guide of STM32H563ZI Nucleo-144 we can find that A0 of Arduino pin is connected to PA6 as following:
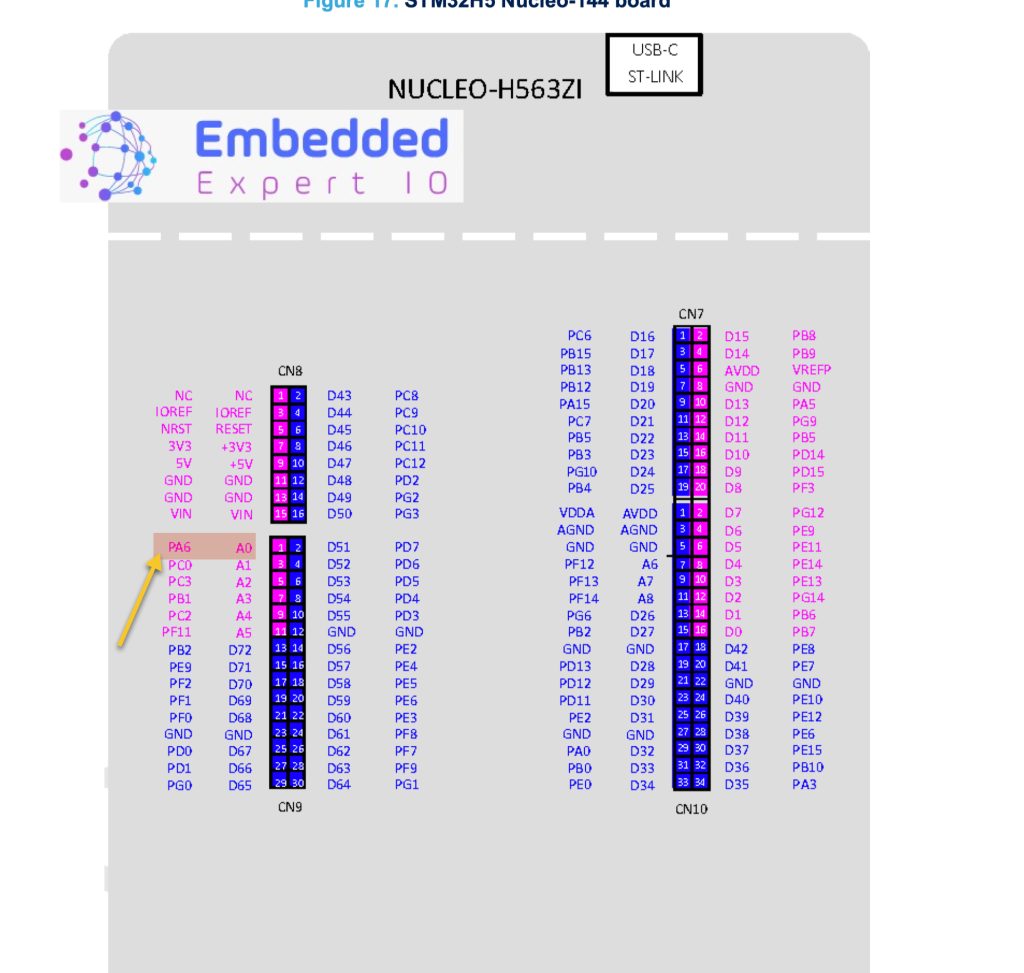
Hence we shall connect external LED through 220Ohm resistor to limit the current as following:
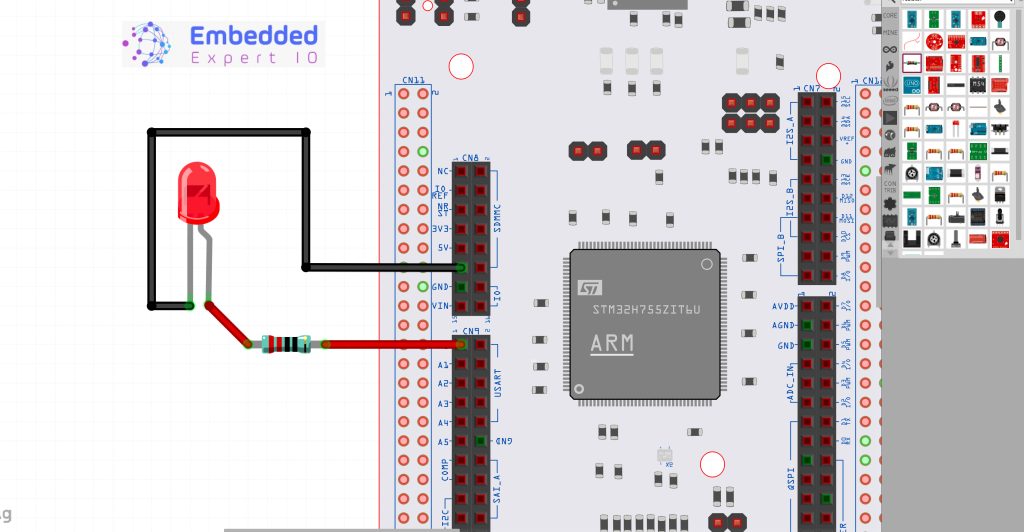
From STM32CubeIDE enable PA6 as TIM3_CH1 as following:
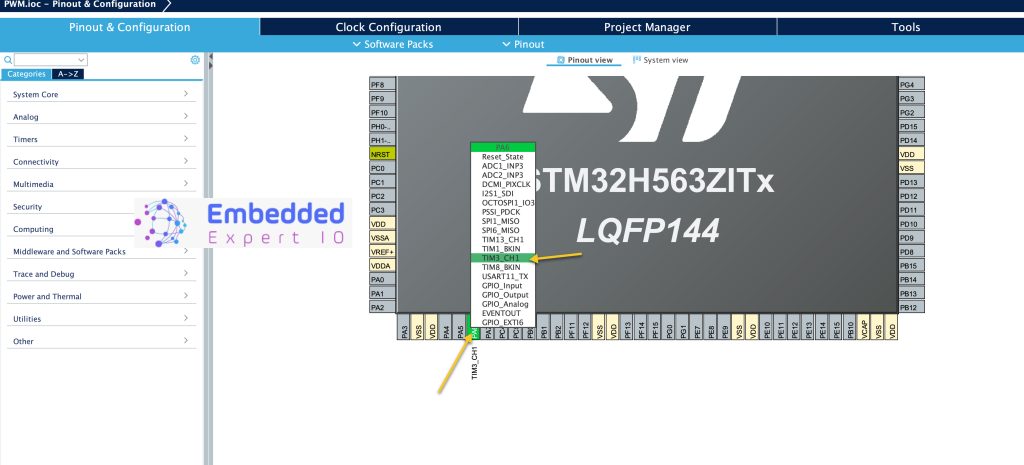
Next, from timers section, enable TIM3 as following:
- Set the clock source to be internal.
- Enable Channel 1 to be in PWM mode as following:
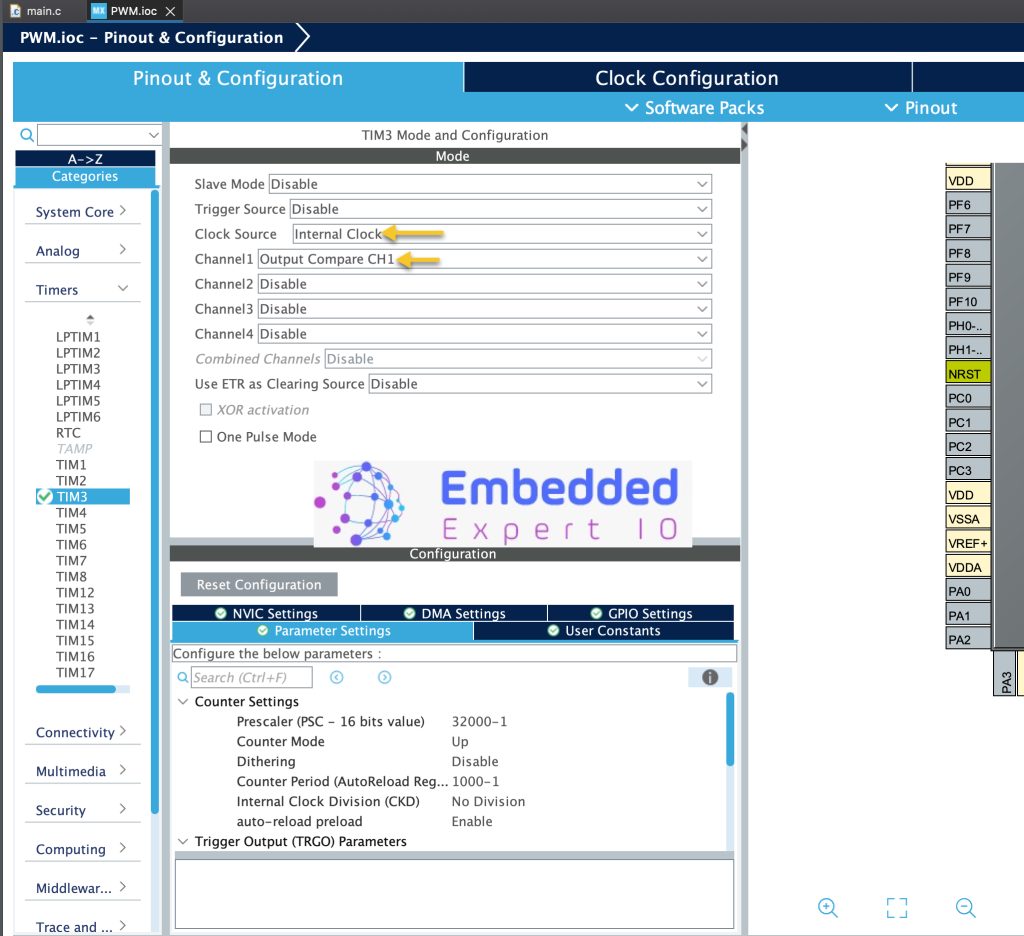
Next from clock configuration, we need to find the frequencies of the timers:
By default, STM32H563Zi is running at 32MHz. Hence, the timers are 32MHz in speed.
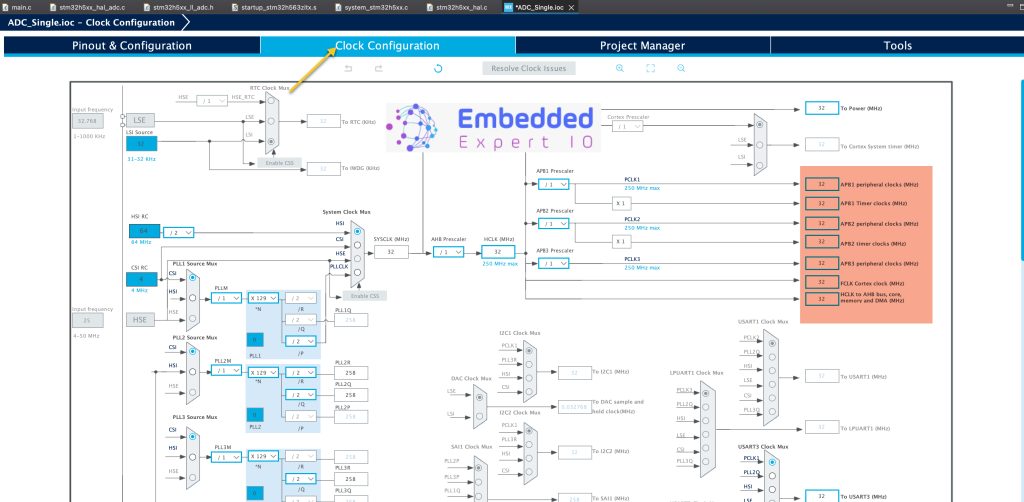
Hence, we can configure the timer with the following parameters:
- Prescaler to 32000-1 which this will reduce the timer frequency to 1KHz.
- ARR to 1000-1, this will let timer counts to 1000 (0-999) which will provide 1Hz output.
- Set the mode to toggle on match for the output compare channel 1.
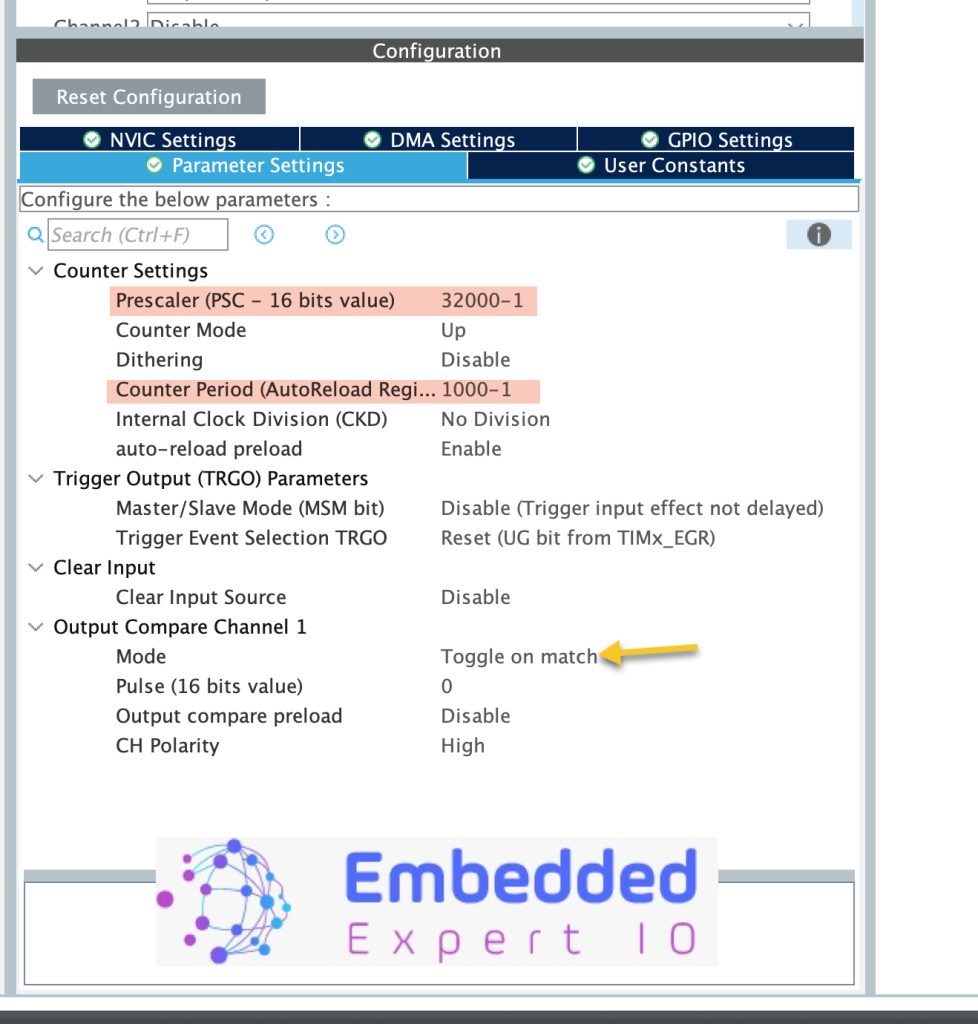
Thats all for STM32CubeMX configuration.
Save the project and this shall generate the project.
3. Firmware Development:
After the code has been generated, the main.c file shall be open.
In user code begin 2 of the main function, we shall start the timer in the output compare mode as following:
HAL_TIM_OC_Start(&htim3, TIM_CHANNEL_1);
HAL_TIM_OC_Start takes the following parameters:
- Instant to the timer which is timer3 in this case.
- Timer channel which is channel1 in this case.
Thats all for the firmware.
Save the project, build it and run it on your STM32H563Zi board.

4. Results:
After the running the code, you should get the following:
Happy coding 😉
Add Comment