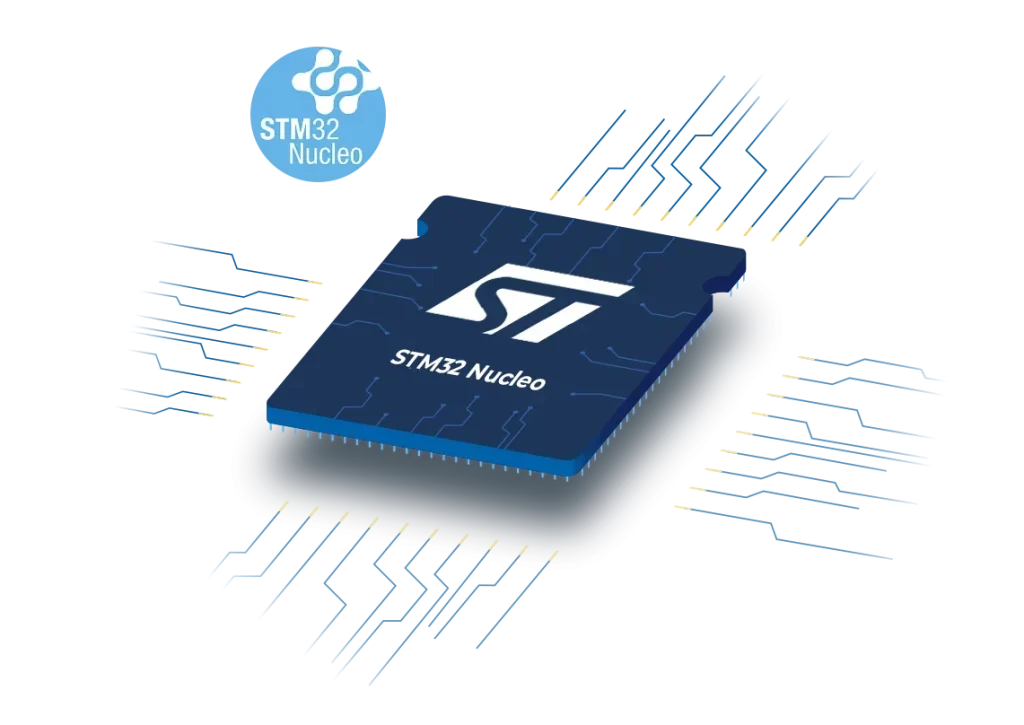
In the previous guide (here), we took a look how to configure DAC and we used polling mode to generate waveform using the DAC. In this guide, we shall use timer and DMA to generate faster waveform while maintaining maximum performance.
In this guide, we shall cover the following:
- STM32CubeMX configuration.
- Firmware development.
- Results.
1. STM32CubeMX Configuration:
From the previous guide, open the .ioc file from project explorer as following:
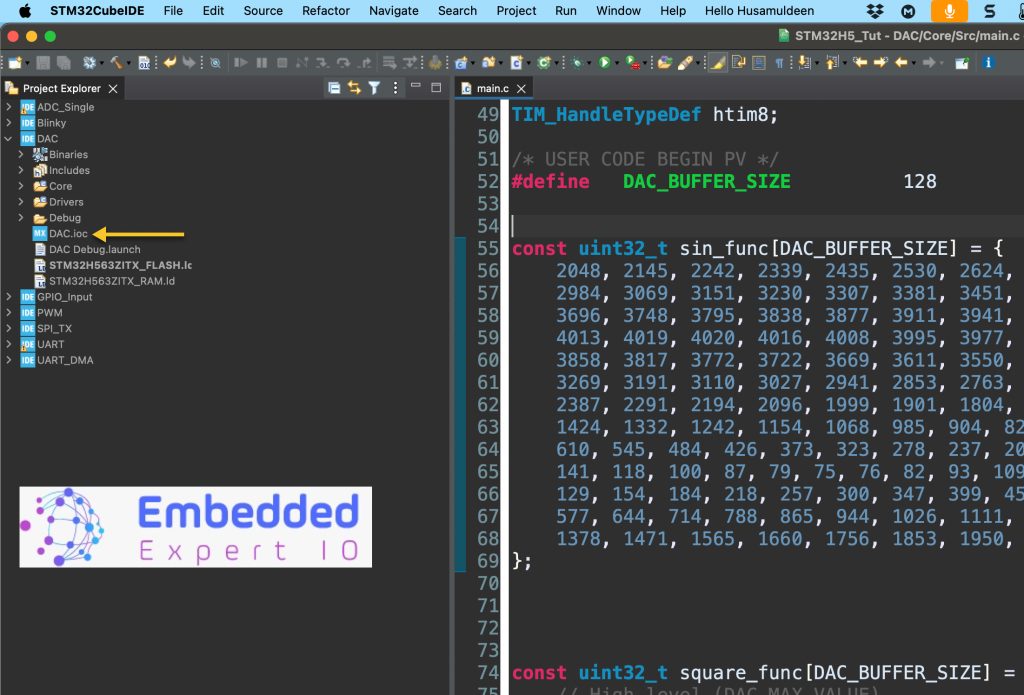
Next, we shall push the MCU to it’s full speed as following:
Select Clock Configuration tab and set HCLK to 250MHz as following:
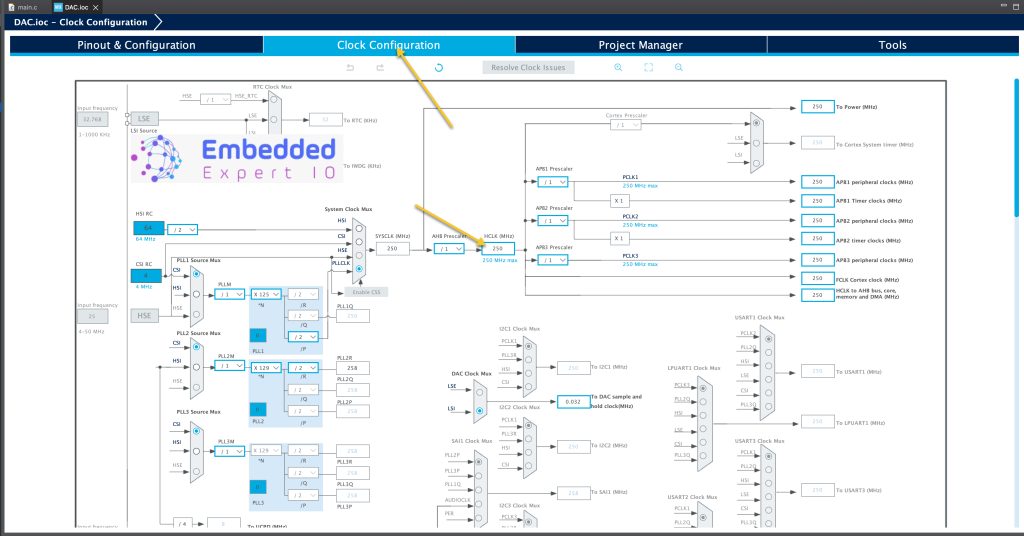
Next from DAC section, set the trigger to be timer8 trigger out event as following:
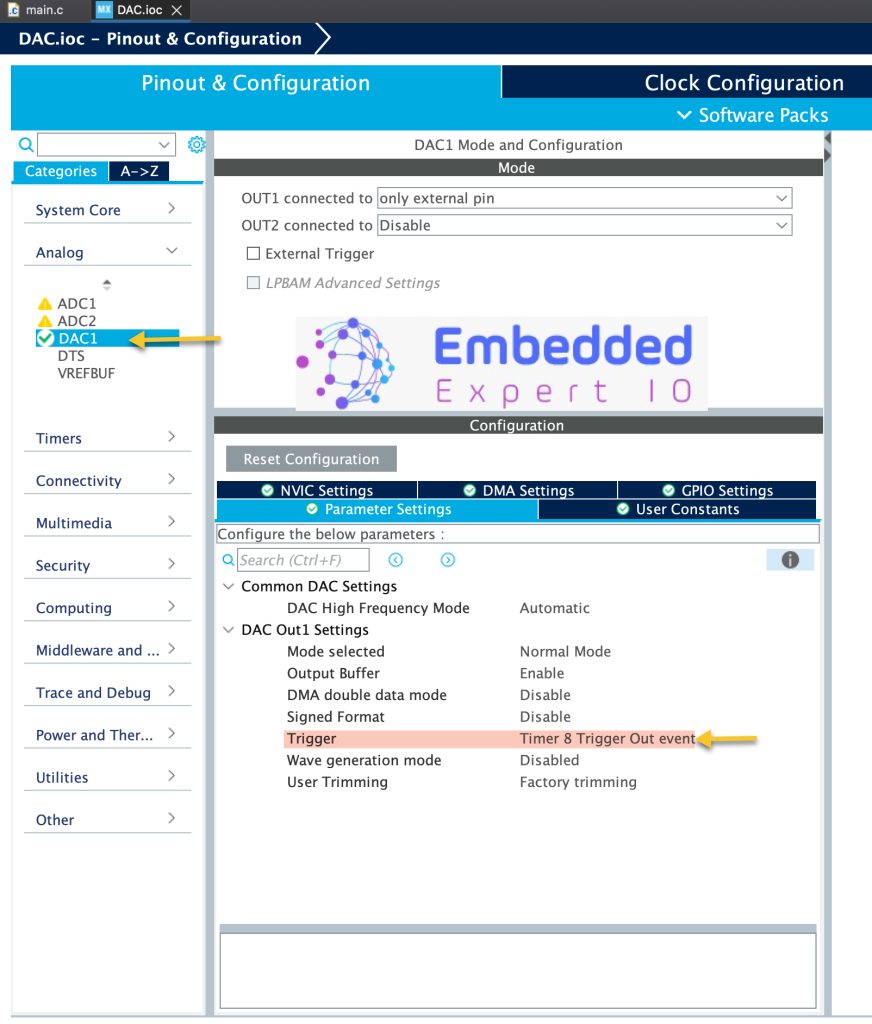
Next, from timer section:
- Set clock source to Internal Clock.
- Set PSC to 1 (No prescaller)
- Set period to 9 (10-1)
- Auto-reload Preload to enable.
- TRGO to Update Event.
As following:
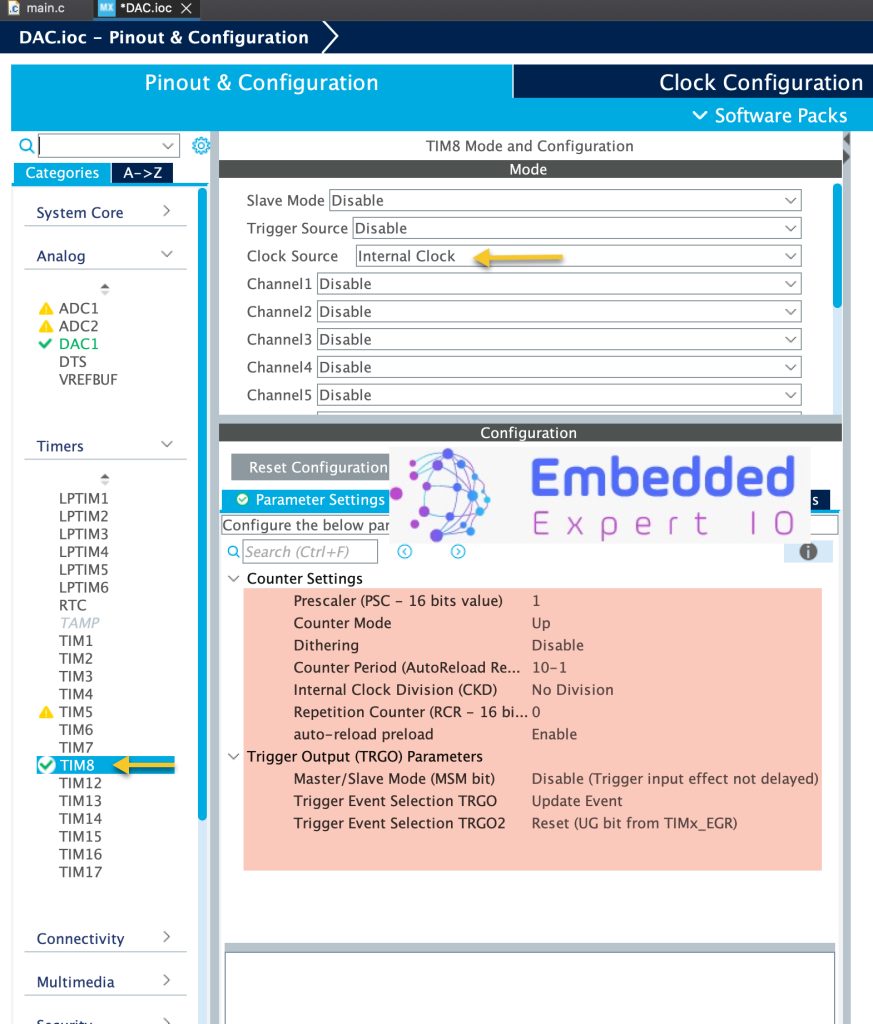
Next, in GPDMA1, enable Ch0 in standard request mode as following:
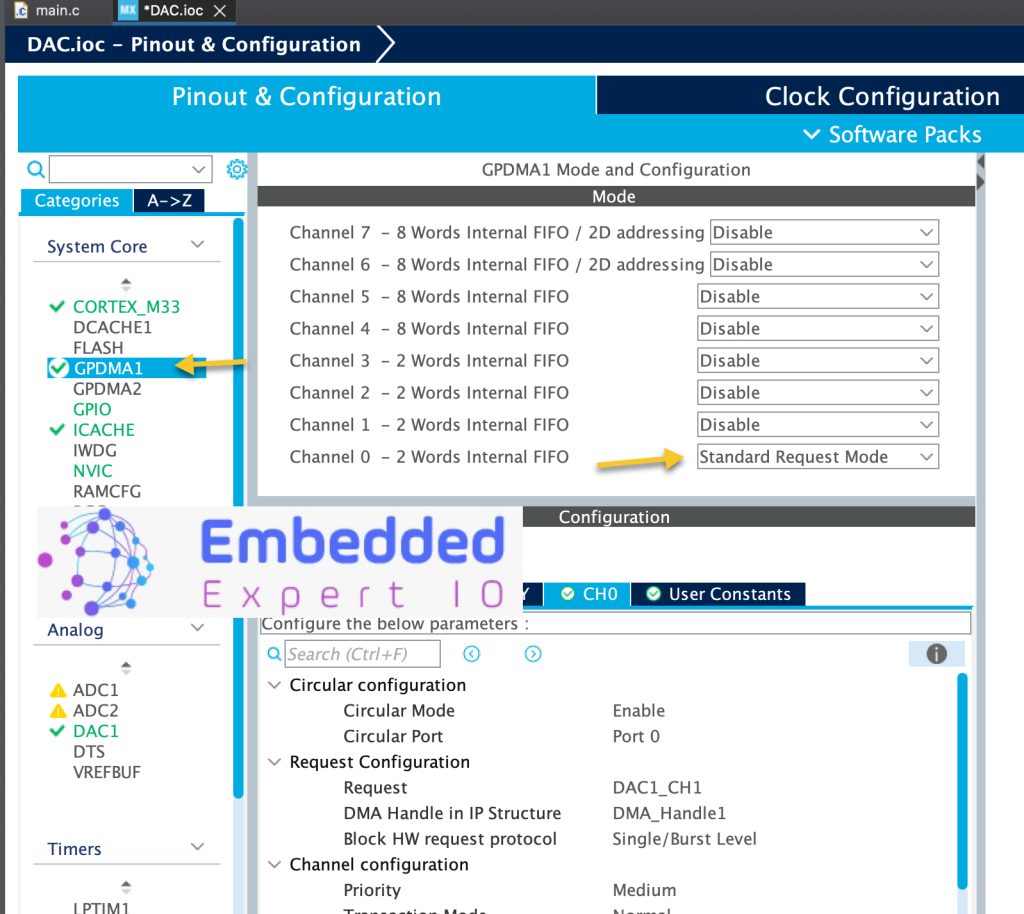
Then configure CH0 as following:
- Enable circular mode.
- Priority to normal.
- Direction Memory to peripheral.
For source Data setting:
- Address increment to enabled.
- Data width to word.
For destination Data:
- Set only data width to word size.
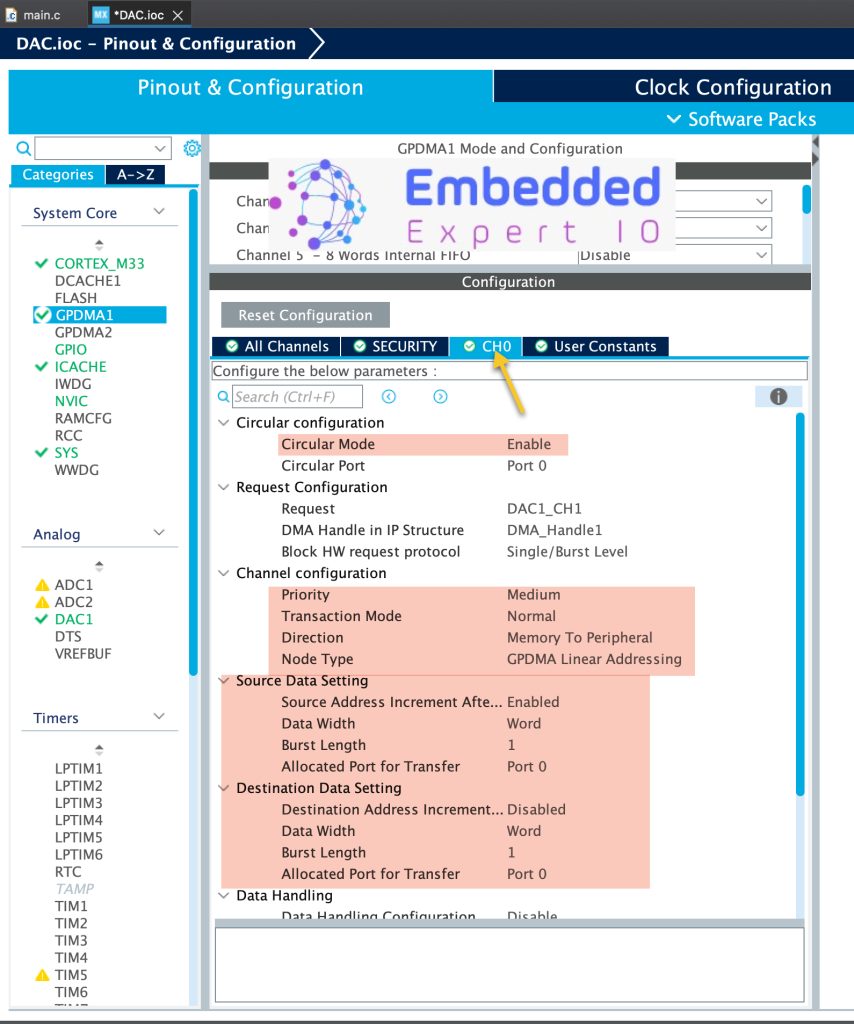
Thats all for the configuration. Save the project and this will generate the project.
2. Firmware Development:
In main.c file, in user code begin PV, declare the following:
#define DAC_BUFFER_SIZE 128 const uint32_t sin_func[DAC_BUFFER_SIZE] = { 2048, 2145, 2242, 2339, 2435, 2530, 2624, 2717, 2808, 2897, 2984, 3069, 3151, 3230, 3307, 3381, 3451, 3518, 3581, 3640, 3696, 3748, 3795, 3838, 3877, 3911, 3941, 3966, 3986, 4002, 4013, 4019, 4020, 4016, 4008, 3995, 3977, 3954, 3926, 3894, 3858, 3817, 3772, 3722, 3669, 3611, 3550, 3485, 3416, 3344, 3269, 3191, 3110, 3027, 2941, 2853, 2763, 2671, 2578, 2483, 2387, 2291, 2194, 2096, 1999, 1901, 1804, 1708, 1612, 1517, 1424, 1332, 1242, 1154, 1068, 985, 904, 826, 751, 679, 610, 545, 484, 426, 373, 323, 278, 237, 201, 169, 141, 118, 100, 87, 79, 75, 76, 82, 93, 109, 129, 154, 184, 218, 257, 300, 347, 399, 455, 514, 577, 644, 714, 788, 865, 944, 1026, 1111, 1198, 1287, 1378, 1471, 1565, 1660, 1756, 1853, 1950, 2047 };
This is a sinewave lookup table.
In user code begin 2, start the timer in the base mode as following:
HAL_TIM_Base_Start(&htim8);
Next, start the DAC in DMA mode as following:
HAL_DAC_Start_DMA(&hdac1, DAC_CHANNEL_1, (uint32_t *)SawTooth_func, DAC_BUFFER_SIZE, DAC_ALIGN_12B_R);
The function shall take the following:
- Instant to the DAC which is hdac1 in this case.
- DAC channel, which is channel 1 in our case.
- Pointer to the data to be written to the DAC.
- Size of the data.
- The alignment which is 12Bit and right alignment.
Thats all for the firmware development.
Save the project, build it and run it on your STM32H563Zi board.

3. Results:
Probe PA4 and you should get the following:

Notice the frequency, it is above 100KHz and yet, the main loop is empty. Which means we are using hardware level to generate the waveform.
Happy coding 😉
Add Comment