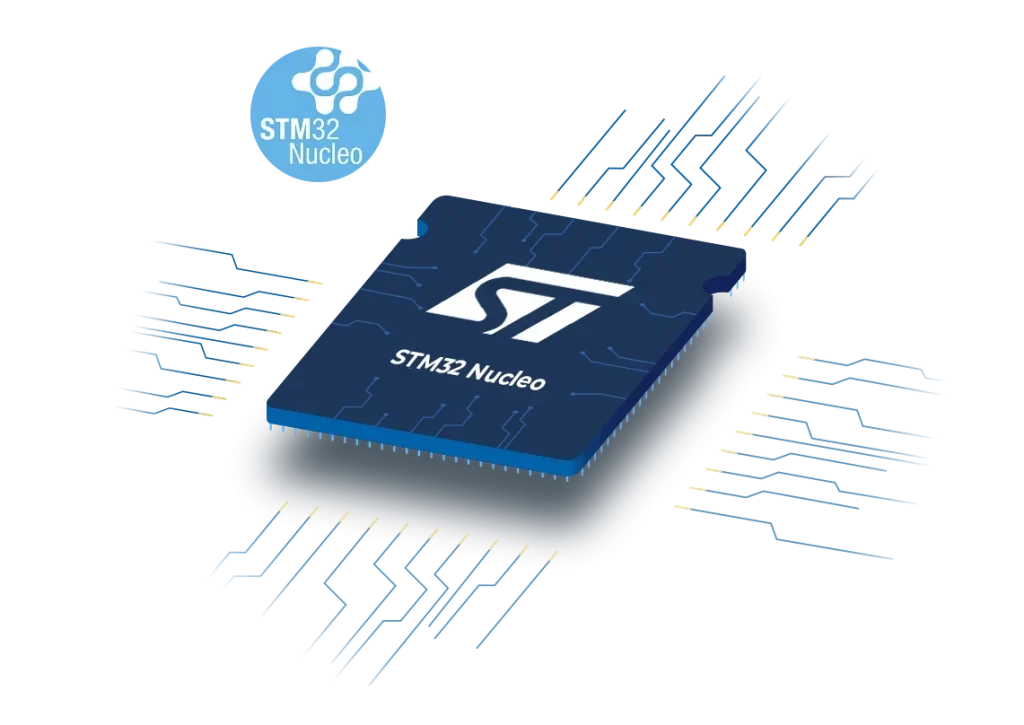
In this guide of STM32H5, we shall receive multiple bytes over UART in interrupt mode and echo back the characters received to our terminal.
In this guide, we shall cover the following:
- CubeMX Configuration.
- Firmware Development.
- Results.
1. CubeMX Configuration:
From Project Explorer, open UART.ioc as following:
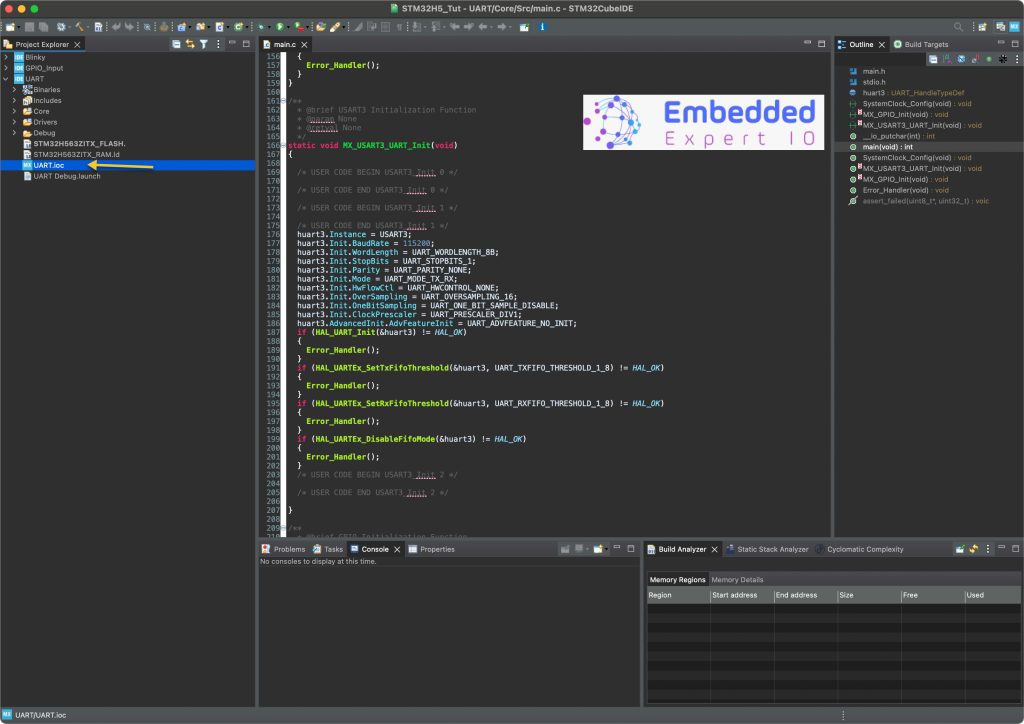
Next, from connectivity, select UART3, then NVIC tab and enable UART3 global interrupt as following:
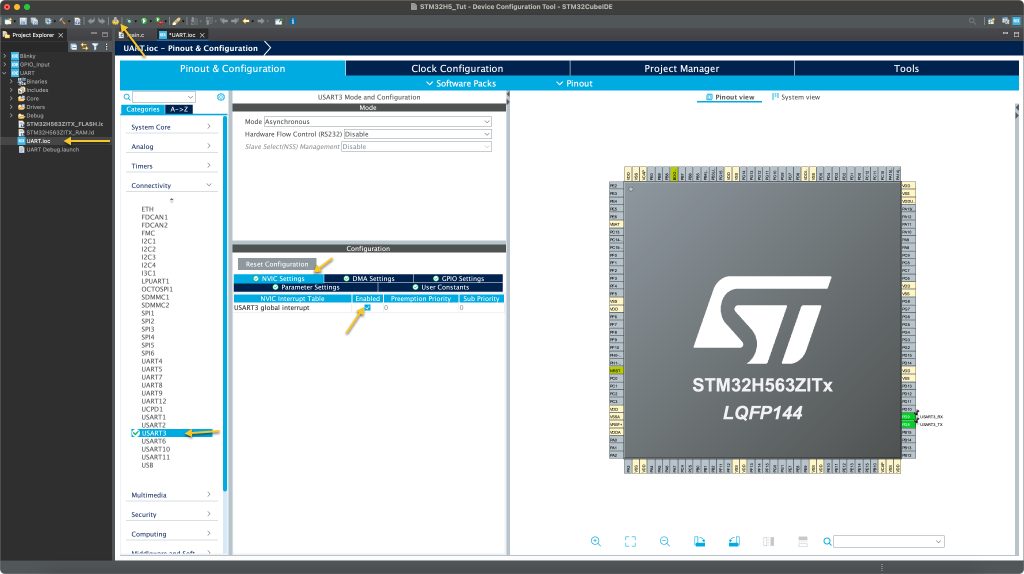
Click on generate to generate the code.
Thats all for the configuration.
2. Firmware development:
First remove everything in while 1 loop from the previous guide since it will be different.
In user code begin PV, declare a buffer to hold the received data as following:
uint8_t RxData[100]={0};
Here, we are allocating 100 bytes (characters) to be received.
Next, also declare the following:
volatile uint8_t RXDone=0;
This will be set in the interrupt callback. Hence, it needs to be volatile and it will signal our application that there is new data to be processed.
Declare a variable to hold the received data as following:
uint16_t size;
In user code begin 2, start the uart reception in interrupt mode:
You have two methods:
First:
HAL_UARTEx_ReceiveToIdle_IT(&huart3, RxData, 100);
This will receive data until the IDLE Line has been detected or 100 characters which one is first.
The IDLE Line means there was no new character reception for 1 character time.
Second:
HAL_UART_Receive_IT(&huart3, RxData, 100);
This will trigger the interrupt handler until all the 100 data has been received.
In this guide, we shall focus on the IDLE line.
Hence, in the user code begin 2 start the reception with IDLE line as following:
HAL_UARTEx_ReceiveToIdle_IT(&huart3, RxData, 100);
In user code begin 4 ,declare the following function:
void HAL_UARTEx_RxEventCallback(UART_HandleTypeDef *huart, uint16_t Size)
This function shall be called once either all the 100 characters has been received or IDLE line is detected.
Within the function, we shall set RXDone to 1 as following:
RXDone=1;
Get the size of received data as following:
size=Size;
In user code begin 3 in while 1 loop, first, check if the flag is set as following:
if(RXDone==1)
If there is new data, process it as following:
Print the received characters:
for (int i=0;i<size;i++) { HAL_UART_Transmit(&huart3, &RxData[i], 1, 10); }
Set the RXDone to zero:
RXDone=0;
Clear the buffer:
for (int i=0;i<100;i++) { RxData[i]=0; }
Restart the reception:
HAL_UARTEx_ReceiveToIdle_IT(&huart3, RxData, 100);
Save the project, build it and run it on your STM32H563Zi board.

3. Results:
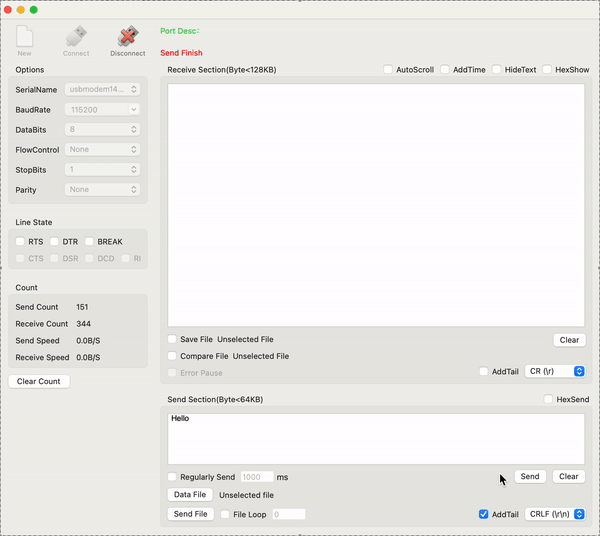
Happy coding 😉
Add Comment