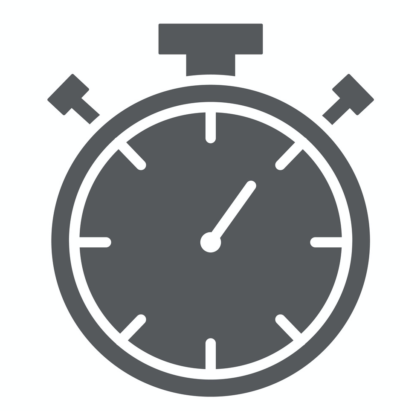
In previous two guides, we discussed how to use timer to generate delay (here) and how to generate PWM signal (here). In this guide, we shall look how to configure TIM2 to generate an interrupt every second and toggle an led every second.
In this guide, we will cover the following
- What is an interrupt
- Configure TIM2 to generate Interrupt
- Code
- Demo
1. What is an interrupt:
Interrupt is a process to notify the cpu that there is a request and it should be handled as soon as possible See figure below.
The CPU will find the current instruction in the main loop (which is empty in our case) and jump to ISR function to toggle PA5 and jump back to main loop.
For more information, please refer to this guide (here)
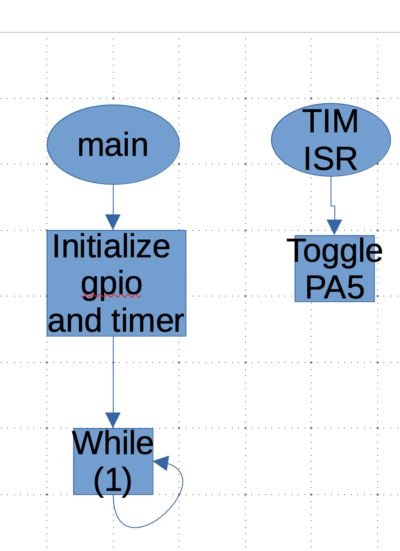
2. Configure TIM2 to generate interrupt
We starting by enabling Clock access to TIM2 as following
RCC->APB1ENR|=RCC_APB1ENR_TIM2EN; //enable TIM2 clock
then select the proper prescaller as following
TIM2->PSC=16000-1;
Then we select the ARR (maximum value the counter shall count)
TIM2->ARR=1000-1;
Enable Update interrupt enable as following
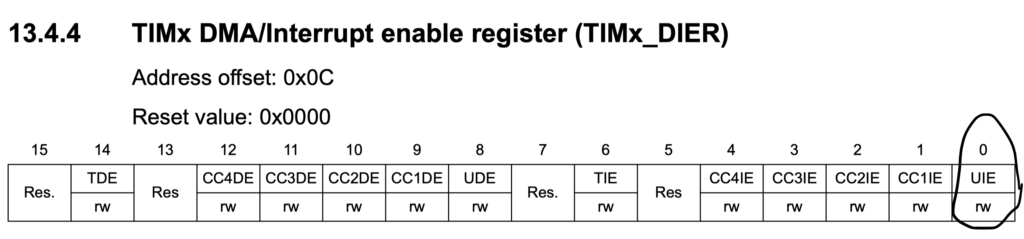
TIM2->DIER|=TIM_DIER_UIE;//enable interrupt
Now we need to enable TIM2 interrupt handler in NVIC as following
NVIC_EnableIRQ(TIM2_IRQn);
Finally, we enable the timer as following
TIM2->CR1|= TIM_CR1_CEN;
After that, the timer interrupt handler as following
void TIM2_IRQHandler(void) { TIM2->SR=0; //clear status register //toggle PA5 GPIOA->ODR^=GPIO_ODR_OD5; }
3. Code
#include "stm32f4xx.h" int main(void) { RCC->APB1ENR|=RCC_APB1ENR_TIM2EN; //enable TIM2 clock RCC->AHB1ENR|=RCC_AHB1ENR_GPIOAEN;//Enable GPIOA clock GPIOA->MODER|=GPIO_MODER_MODE5_0;//set PA5 as output //set timer prescaller and Auto Reload value TIM2->PSC=16000-1; TIM2->ARR=1000-1; TIM2->DIER|=TIM_DIER_UIE;//enable interrupt NVIC_EnableIRQ(TIM2_IRQn); TIM2->CR1|= TIM_CR1_CEN; while(1) { ; } } void TIM2_IRQHandler(void) { TIM2->SR=0; //clear status register //toggle PA5 GPIOA->ODR^=GPIO_ODR_OD5; }
Add Comment