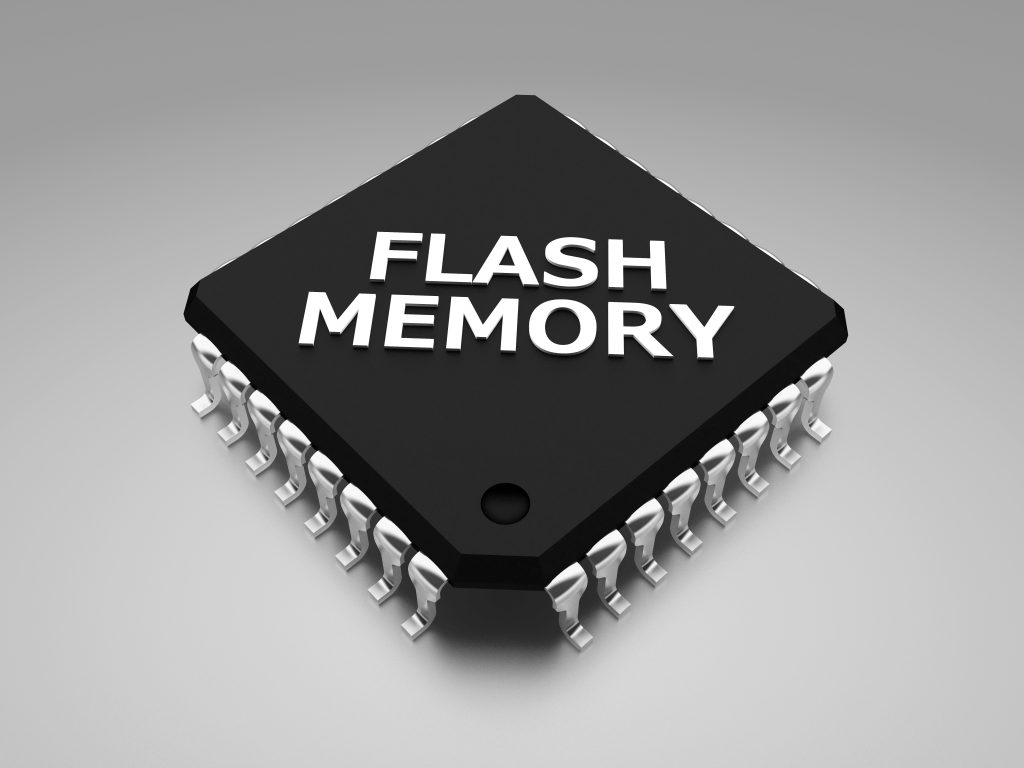
In this guide, we shall see how to read data from the internal flash storage of STM32Fxxx (tested on STM32F1 and F4).
In this guide, we shall cover the following:
- Internal Flash Storage of STM32F411.
- Develop the driver.
- Code.
- Results.
1. Internal Flash Storage of STM32F411:
The Flash memory interface manages CPU AHB I-Code and D-Code accesses to the Flash memory. It implements the erase and program Flash memory operations and the read and write protection mechanisms.
The Flash memory interface accelerates code execution with a system of instruction prefetch and cache lines.
Main features
• Flash memory read operations
• Flash memory program/erase operations
• Read / write protections
• Prefetch on I-Code
• 64 cache lines of 128 bits on I-Code
• 8 cache lines of 128 bits on D-Code
Figure below shows the Flash memory interface connection inside the system architecture.
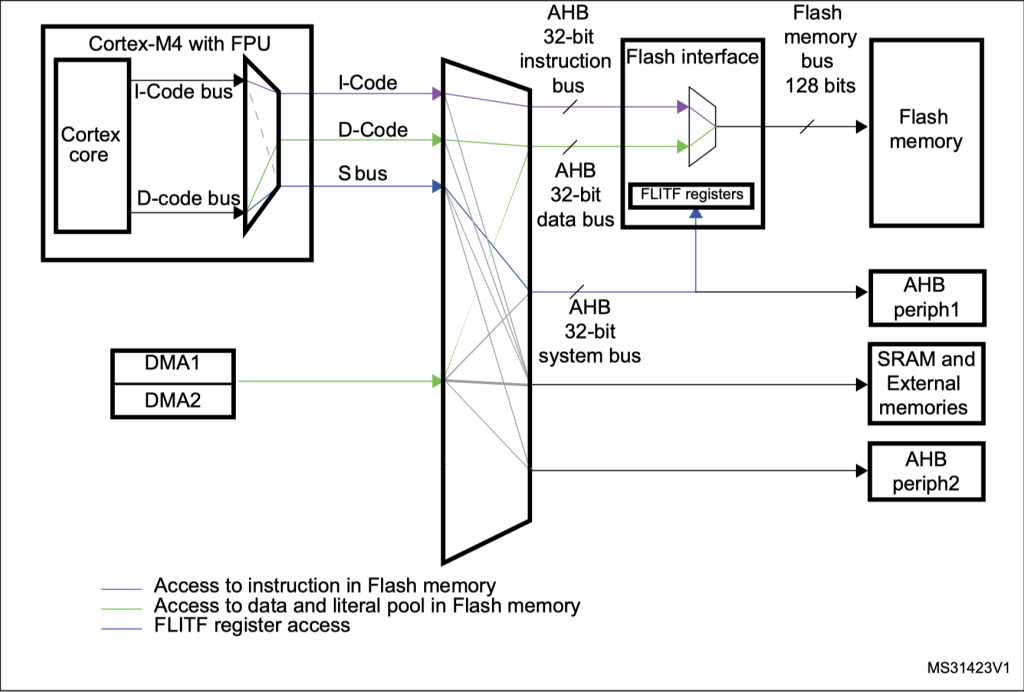
The Flash memory has the following main features:
• Capacity up to 512 KBytes for STM32F411xC/E
• 128 bits wide data read
• Byte, half-word, word and double word write
• Sector and mass erase
• Memory organization The Flash memory is organized as follows:
– A main memory block divided into 4 sectors of 16 KBytes, 1 sector of 64 KBytes, 3 sectors of 128 Kbytes (STM32F411xC/E).
– System memory from which the device boots in System memory boot mode
– 512 OTP (one-time programmable) bytes for user data The OTP area contains 16 additional bytes used to lock the corresponding OTP data block.
– Option bytes to configure read and write protection, BOR level, watchdog software/hardware and reset when the device is in Standby or Stop mode.
• Low-power modes (for details refer to the Power control (PWR) section of the reference manual)
Figure below shows the flash storage organization within STM32F411:
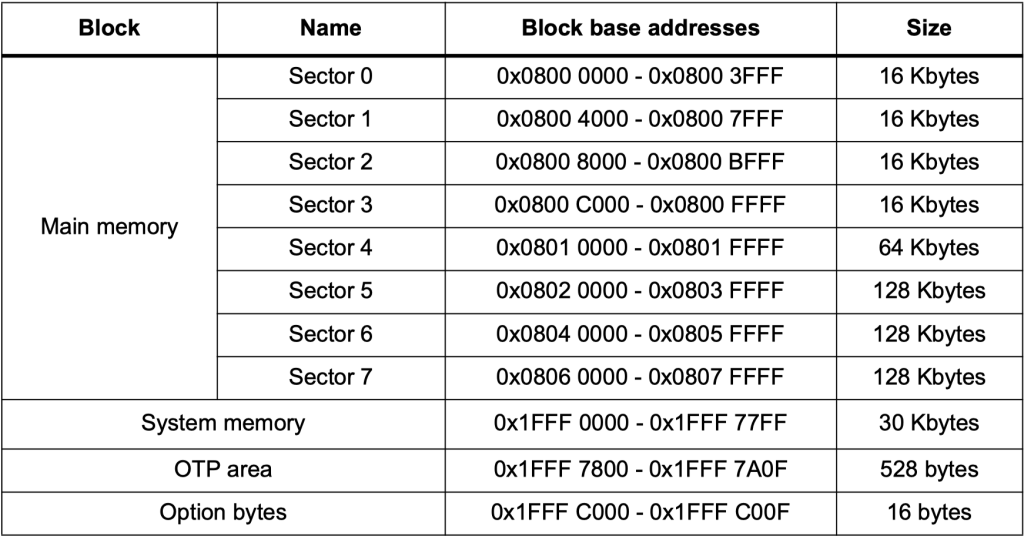
2. Developing the Driver:
We start off by creating new source and header file with name of flash.c and flash.h respectively.
Within the header, include stdint as following:
#include "stdint.h"
Declare the following function:
void flash_read32(uint32_t addrs, uint32_t *rd32, uint32_t len);
The function takes three arguments:
- The address to be read.
- pointer to hold the read address.
- Length to be read.
And the function returns nothing.
Within source code, include the flash.h and stm32f4xx.h header files as following:
#include "Flash.h" #include "stm32f4xx.h"
For the function to read from flash:
void flash_read32(uint32_t addrs, uint32_t *rd32, uint32_t len) {
Within the function:
Store the passed address into temporary variable:
uint32_t tempAddrs = addrs;
Declare a for loop starts from 0 to the length:
for(uint32_t i=0; i<len; i++)
Within the loop:
rd32[i] = *(__IO uint32_t*) tempAddrs;
convert the temporary variable into pointer then dereference the pointer and store the read memory location into rd32 array.
Increment the address by 4 since the datawidth is 4byte:
tempAddrs+=4;
close the function:
}
Hence, the function as following:
void flash_read32(uint32_t addrs, uint32_t *rd32, uint32_t len) { //Read data can be accessed straight away uint32_t tempAddrs = addrs; for(uint32_t i=0; i<len; i++) { rd32[i] = *(__IO uint32_t*) tempAddrs; tempAddrs+=4; } }
Within main.c file:
#include "stdio.h" #include "delay.h" #include "flash.h" #include "uart.h" uint32_t flash_data[4]; int main(void) { uart2_tx_init(115200,16000000); systick_init_ms(16000000); flash_read32(0x08000000,flash_data,4); printf("First 4 word data in flash are:\r\n"); for (int i=0;i<4;i++) { printf("%d -> 0x%x\r\n",i,flash_data[i]); } while(1) { } }
3. Code:
You may download the entire source code from here:
4. Results:
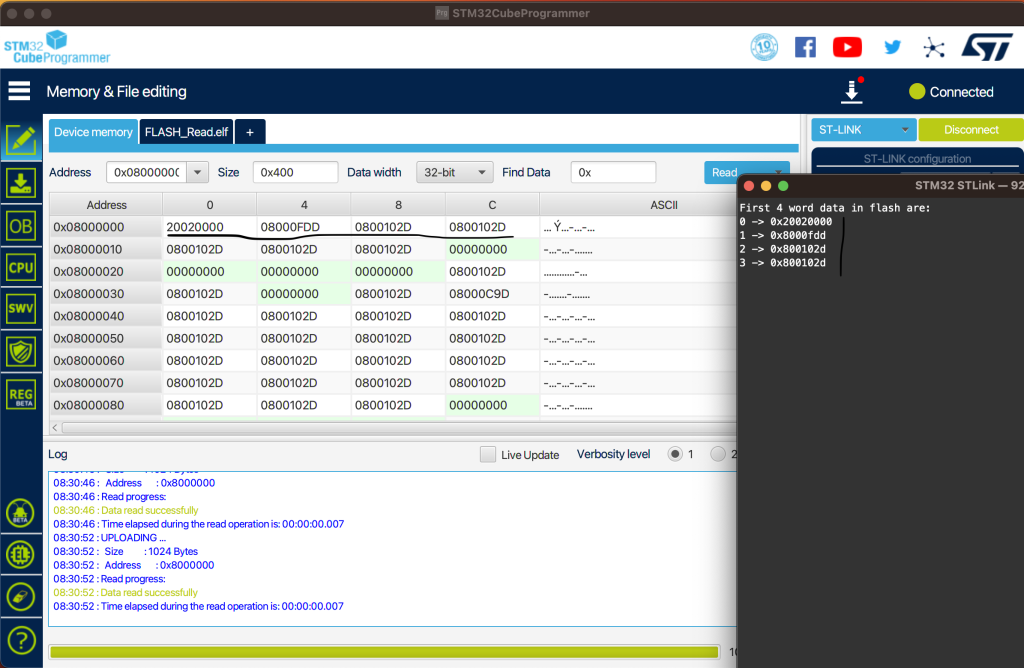
Successfully Read the first 16 Bytes of the flash storage.
Happy coding 🙂
Add Comment